This section describes the classic approach to creating functional web tests with TestComplete. It relies on the support TestComplete provides for the most popular web browsers listed below.

Supported Web Browsers and Technologies
How TestComplete Identifies Web Applications
Viewing Object Properties and Methods
Supported Web Browsers and Technologies
-
TestComplete lets you test web applications in the following web browsers:
-
Microsoft Edge 83 - 121 (Chromium-based, both 32- and 64-bit versions)
Note: TestComplete does not support the non-Chromium version of Edge.
-
Microsoft Internet Explorer 11 (both 32- and 64-bit versions)
Note: The Modern UI (Metro) version of Internet Explorer 11 is currently not supported.
-
Google Chrome 121 (both 32- and 64-bit versions)
If you have a TestComplete version earlier than 12.42, your version of the SmartBear Test Extension will be incompatible with newer versions of the Chrome web browser. To test web applications in Chrome 121, you will have to upgrade your TestComplete to some later version. We recommend using version 15.63.
If you use a later version of Google Chrome, check whether a patch is available for it.
Chrome running in Modern UI (Metro) mode is currently not supported.
-
Mozilla Firefox 91 - 115.4.0 ESR, 94 - 116 (both 32- and 64-bit versions)
If you use a later version of Firefox, check whether a patch is available for it.
-
Applications with embedded Microsoft WebBrowser controls
Note: The Edge-based WebBrowser control is not supported.
-
Applications with Chromium Embedded Framework controls (both 32- and 64-bit editions)
Each supported browser has certain specifics that should be taken in account during automated web testing. Read Considerations for Web Testing for details. TestComplete web tests are cross-browser, which means you can record tests in one browser and run them in another browsers, instead of having to create individual tests for different browsers.
-
-
TestComplete works with web pages through their GUI that does not depend on the technology used on the server. It can test web applications created with ASP, ASP.NET, PHP, CGI and other technologies.
-
TestComplete supports various web controls: standard HTML elements, jQuery UI, YUI, GWT, Developer Express, Telerik controls and others. It records and simulates interactions with these controls using high-level object-specific operations and provides checkpoints for verifying data and properties of these controls.
-
You can create automated tests for Rich Internet Applications, such as Adobe Flash, Flex and Microsoft Silverlight.
For detailed information on the supported browsers and web application technologies, see Supported Web Browsers and Technologies and Support for Web Controls.
Creating Web Tests
You can create tests by recording operations in a web browser, or you can create tests manually. Usually, it is easier to record a test first and then modify and enhance it.
When you are recording a test, you interact with the tested web application as an end user would: navigate to pages, fill out forms and so on. TestComplete captures all the actions you performed in the web browser and adds them to the test.
A web test typically starts with the operation that opens the tested web site in the browser.
Keyword test |
Scripted test |
The other test commands define various interactions with web pages and elements. For example, the sample test below fills out and submits a login form:
Keyword test |
Scripted test JavaScript, JScript function Test1() Python def Test1(): VBScript Sub Test1() DelphiScript procedure Test1(); C++Script, C#Script function Test1() |
By default, the recorded tests use object names specified in Name Mapping (the “object repository”). For example, the Aliases.browser
object corresponds to the web browser launched by the test. It is an abstract object that matches the currently used browser rather than a specific browser. So, if you select another browser for a test run, the Aliases.browser
object will point to the new browser.
The recorded tests can be modified and enhanced in a number of ways to create more flexible and efficient tests. For example, you can:
-
Add new operations, reorder them and modify their parameters.
-
Delete or disable unneeded operations (for example, unneeded recorded commands).
-
Create data-driven tests that run multiple test iterations using different sets of data.
-
Insert checkpoints for verifying:
- data and attributes of web page elements,
- the absence of broken links,
- alternative (ALT) text of images,
- web page accessibility,
- static web page structure,
- and so on.
To get started with creating automated web tests, walk through the Testing Web Applications - Tutorial. It is recommended for novice users and for users who do not have experience in automating web tests.
To learn more about creating and enhancing web tests, refer to the following topics.
To learn more about… | See these topics… |
---|---|
Recording web tests | Creating and Recording Web Tests |
Creating and editing tests manually | Keyword Tests and About Script Tests |
Launching web browsers | Launch Web Browsers |
Finding objects on web pages | How To |
Verifying web page elements | Validate Web Pages |
Verifying web page contents | About Web Accessibility Checkpoints About Web Comparison Checkpoints |
Automating modal dialog boxes | Handling JavaScript Popups and Browser Dialogs |
Common tasks that you perform to create tests in TestComplete | How To |
Examples of typical web testing solutions | Web Testing - Examples |
Running web tests | Running Tests |
Running multiple test iterations using data from an external file | Data-Driven Testing |
Cross-Browser Web Testing
TestComplete enables you to perform cross-browser compatibility testing across multiple web browsers. You can record web tests in any supported browser and then run tests in other browsers with no or minimum changes in the test logic or Name Mapping (the “object repository”). There is no need to create individual tests for different browsers, which simplifies test development and maintenance greatly. For more information, see Cross-Browser Testing in TestComplete.
How TestComplete Identifies Web Applications
The following image shows web test objects that TestComplete uses to work with web browsers and web pages:
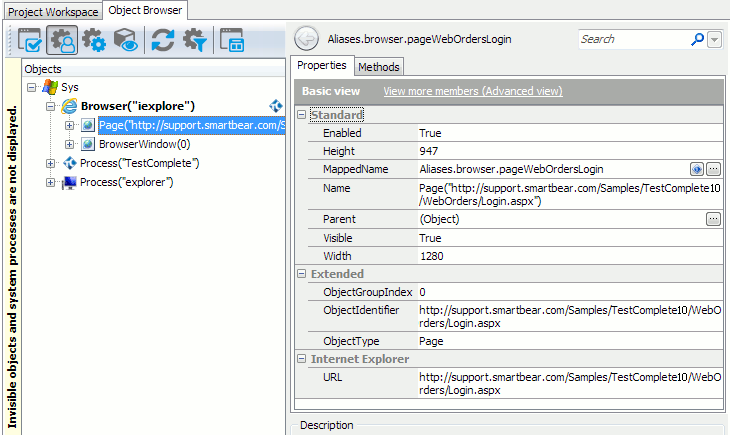
A web browser is represented by the Browser
test object that is a child object of the root Sys
object that, in its turn, represents the operating system.
Each Browser
test object has the following child objects:
-
One or more
Page
objects corresponding to web pages that are open in browser tabs. They provide further access to web page elements. -
One or more
BrowserWindow
objects that correspond to browser windows. These objects provide operations for automating the browser window: maximizing, minimizing, resizing, positioning on screen, closing and so on. -
Browser
objects corresponding to Firefox also have a childUIPage
object that exposes Firefox’s XUL-based UI elements - the address bar, menu items and so on.
To view the objects that TestComplete identifies on a web page, expand the Page
object in the Object Browser:
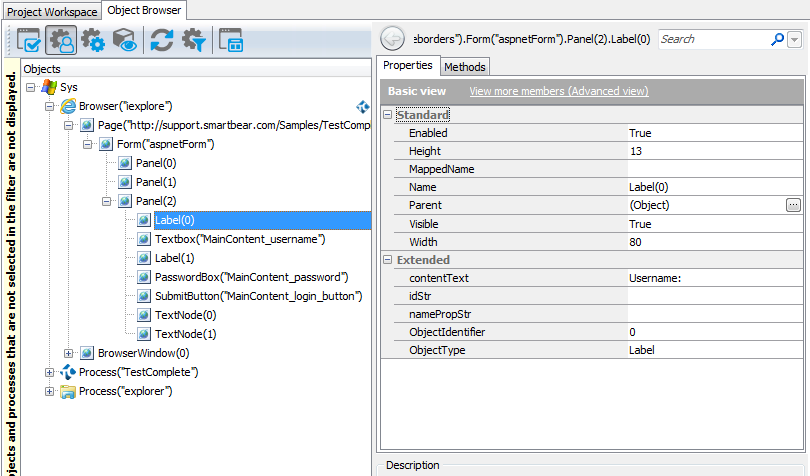
Viewing Object Properties and Methods
-
To see the web object properties and methods available for use in tests, you can use the Object Browser or Object Spy. The available methods and properties include those provided by TestComplete, as well as those provided by the web browser (for example, DOM methods and properties).
-
Important: some web element methods and properties provided by web browsers are not displayed in the Object Browser and Object Spy by default. For example, this happens to objects that return style information and that are accessed through the
style
,currentStyle
orruntimeStyle
property. If you try to view methods or properties of such objects, TestComplete will display an empty list in the Object Browser or Object Spy.To view a complete list of object methods and properties, enable the Show hidden members option in Tools | Options | Engines.
-
Depending on the web browser you are using and depending on the web page type, the properties of some web elements can be displayed on the Fields tab of the Object Browser or Object Spy. This is how the browser returns information about properties. So, if you do not find a property on the Properties tab, search for it in Fields.
-
You can view object properties and methods both at design time and at run time if the test is paused.
For more information on using the Object Browser, see Object Browser and Exploring Applications.
Access to Native Properties and Methods of Web Elements
TestComplete has access to all native methods and properties of web elements that the browser provides. Your tests have the same access to web elements that JavaScript scripts running on your tested page have. TestComplete provides this kind of access automatically, there is no need to prepare web pages for that.
Using native properties and methods of web elements, you can get their data, state and style and automate specific operations that are not available through TestComplete built-in operations.
For more information on using web element members provided by web browsers, see Access Native Web Attributes and Methods.
Samples
TestComplete includes a number of sample web applications and test projects for them. You can examine these samples to better understand how to implement some common automated testing scenarios. See Web Testing Samples.
See Also
Classic Web Testing
Testing With TestComplete
Working With TestComplete