The information in this topic applies to web tests that implement the classic approach (rely on Name Mapping and run in local environments). To learn how to launch web browsers in cross-platform tests, see Connect to Device Lab and Launch Web Browsers.
This topic explains how you can automatically launch a web browser from your tests.
To learn how to determine if a browser is currently running, see Check if Browser Is Running.
Launching a Browser From Keyword Tests
Launching a Browser From Scripts
Running a Specific Browser Version
Specifying Browser Command-Line Arguments
Launching a Browser From Keyword Tests
To start a web browser from keyword tests, use the Run Browser operation and specify the needed browser and the web page to open.
![]() Run Browser wizard: selecting browser |
![]() Run Browser wizard: specifying URL |
To modify a test to run in another browser, select the browser in the Run Browser operation parameters. For example, you can choose a browser from a combo box in the Operation column of the Keyword Test editor.
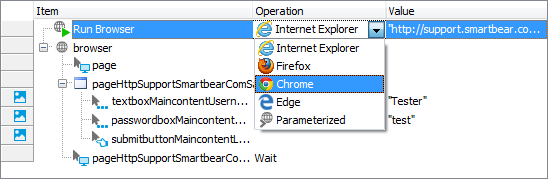
Selecting a web browser for a test run
To execute a test in multiple browsers, substitute the Run Browser operation with the Browser Loop operation and make the subsequent test actions over the web page as children of the Browser Loop operation. The operation allows you to define a list of browsers to be launched, starts them one by one and executes the child operations in each browser. See, Running Tests in Multiple Browsers.

Iterating through browsers with the Browser Loop operation
Launching a Browser From Scripts
To launch a web browser from scripts, use the Browsers.Item(...).Run
scripting method. For example:
JavaScript, JScript
// Open smartbear.com in Chrome browser:
Browsers.Item(btChrome).Run("http://smartbear.com");
Python
# Open smartbear.com in Chrome browser:
Browsers.Item[btChrome].Run("http://smartbear.com");
VBScript
' Open smartbear.com in Chrome browser:
Browsers.Item(btChrome).Run "http://smartbear.com"
DelphiScript
// Open smartbear.com in Chrome browser:
Browsers.Item(btChrome).Run('http://smartbear.com');
C++Script, C#Script
// Open smartbear.com in Chrome browser:
Browsers["Item"](btChrome)["Run"]("http://smartbear.com");
You can specify a web browser in one of the following ways:
Description | Possible Values | Example |
---|---|---|
Using predefined browser type constants | btIExplorer btFirefox btChrome btEdge |
Browsers.Item(btIExplorer).Run("http://smartbear.com")
|
Using the browser's file name | "iexplore" "firefox" "chrome" "edge" |
Browsers.Item("iexplore").Run("http://smartbear.com")
|
Using the index | 0 … Browsers.Count ‑1 |
Browsers.Item(0).Run("http://smartbear.com")
|
If you omit the URL in the Run
method call, the browser will open with a blank page (about:blank).
JavaScript, JScript
Browsers.Item("iexplore").Run();
Python
Browsers.Item["iexplore"].Run();
VBScript
Browsers.Item("iexplore").Run
DelphiScript
Browsers.Item('iexplore').Run;
C++Script, C#Script
Browsers["Item"]("iexplore")["Run"]();
In this case, you need to insert additional operations for pointing the browser to the needed page (see Navigate to Web Pages).
To modify a test to run it in another browser, just change the browser name in the Browsers.Item(...).Run
scripting method.

To execute a test in multiple browsers, use loops or enumerator statements to iterate through the collection of the supported browsers. For detailed information and examples, see Running Tests in Multiple Browsers.
Running a Specific Browser Version
If you have both 32- and 64-bit versions of a browser installed (for example, 32-bit and 64-bit editions of Internet Explorer on a 64-bit version of Windows), by default, TestComplete will launch the 32-bit version of the browser.
If you have several versions of the same browser installed (for example, Firefox 52 and Firefox 55), TestComplete will launch the first browser version it finds.
To launch a specific browser version or a browser with certain bitness, you need to specify additional parameters for the Run Browser operation or the Browsers.Item
method.
In keyword tests, select the Parameterized option in the parameters of the Run Browser operation and then specify the needed values for the Browser, Version and Platform parameters.
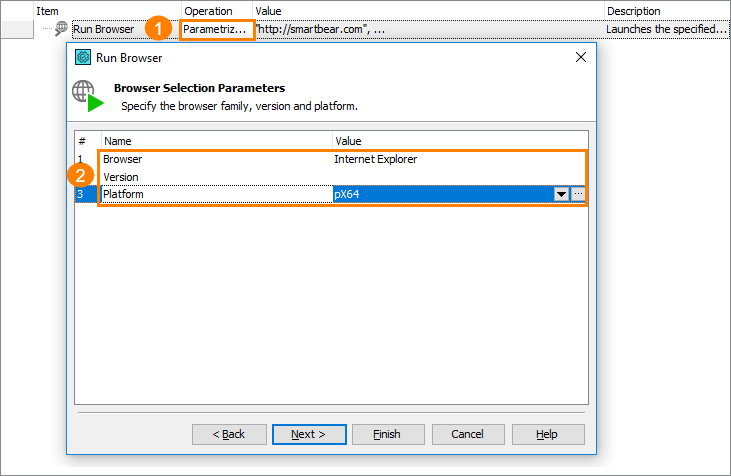
Running a specific browser version from keyword tests
In scripts, specify the needed values for the Index, Version and Platform parameters of the Browsers.Item
method.
JavaScript, JScript
Browsers.Item("iexplore", "11", Browsers.pX64).Run("http://smartbear.com");
Python
Browsers.Item["iexplore", "11", Browsers.pX64].Run("http://smartbear.com");
VBScript
Browsers.Item("iexplore", "11", Browsers.pX64).Run "http://smartbear.com"
DelphiScript
Browsers.Item('iexplore', '11', Browsers.pX64).Run('http://smartbear.com');
C++Script, C#Script
Browsers["Item"]("iexplore", "11", Browsers["pX64"])["Run"]("http://smartbear.com");
Specifying Browser Command-Line Arguments
You may need to pass specific command-line arguments to the web browser, for example, Firefox's profile name or Chrome’s --allow-file-access-from-files
key. For this purpose, specify them in the Browsers.Item(<browser_name>).RunOptions
property.
In scripts, this will look like the following:
JavaScript, JScript
Browsers.Item("firefox").RunOptions = "-P \"Tester\"";
Browsers.Item("firefox").Run("http://smartbear.com");
Python
Browsers.Item["firefox"].RunOptions = "-P \"Tester\"";
Browsers.Item["firefox"].Run("http://smartbear.com");
VBScript
Browsers.Item("firefox").RunOptions = "-P ""Tester"""
Browsers.Item("firefox").Run "http://smartbear.com"
DelphiScript
Browsers.Item('firefox').RunOptions := '-P "Tester"';
Browsers.Item('firefox').Run('http://smartbear.com');
C++Script, C#Script
Browsers["Item"]("firefox")["RunOptions"] = "-P \"Tester\"";
Browsers["Item"]("firefox")["Run"]("http://smartbear.com");
In keyword tests, use the Call Object Method or Run Code Snippet operation to access the Browsers.Item(<browser_name>).RunOptions
property. You should insert this operation before the Run Browser operation.

Parameterizing the Browser Name
Instead of hard-coding the browser name in your test, you can specify it using an external parameter, such as a test parameter or a project variable.
In scripts, this will look like the following:
JavaScript, JScript
// Specify the browser using a test parameter
function Test1(BrowserName)
{
Browsers.Item(BrowserName).Run("http://smartbear.com");
// Test the web site
...
}
// Specify the browser using a project variable
function Test2()
{
Browsers.Item(Project.Variables.BrowserName).Run("http://smartbear.com");
// Test the web site
...
}
Python
# Specify the browser using a test parameter
def Test1(BrowserName):
Browsers.Item[BrowserName].Run("http://smartbear.com");
# Test the web site
#...
# Specify the browser using a project variable
def Test2():
Browsers.Item[Project.Variables.BrowserName].Run("http://smartbear.com");
# Test the web site
#...
VBScript
' Specify the browser using a test parameter
Sub Test1(BrowserName)
Browsers.Item(BrowserName).Run "http://smartbear.com"
' Test the web site
...
End Sub
' Specify the browser using a project variable
Sub Test2
Browsers.Item(Project.Variables.BrowserName).Run "http://smartbear.com"
' Test the web site
...
End Sub
DelphiScript
// Specify the browser using a test parameter
procedure Test1(BrowserName);
begin
Browsers.Item(BrowserName).Run('http://smartbear.com');
// Test the web site
...
end;
// Specify the browser using a project variable
procedure Test2();
begin
Browsers.Item(Project.Variables.BrowserName).Run('http://smartbear.com');
// Test the web site
...
end;
C++Script, C#Script
// Specify the browser using a test parameter
function Test1(BrowserName)
{
Browsers["Item"](BrowserName)["Run"]("http://smartbear.com");
// Test the web site
...
}
// Specify the browser using a project variable
function Test2()
{
Browsers["Item"](Project["Variables"]["BrowserName"])["Run"]("http://smartbear.com");
// Test the web site
...
}
In keyword tests, select the Parameterized option in the parameters of the Run Browser operation and then set the needed value for the operation’s Browser parameter.
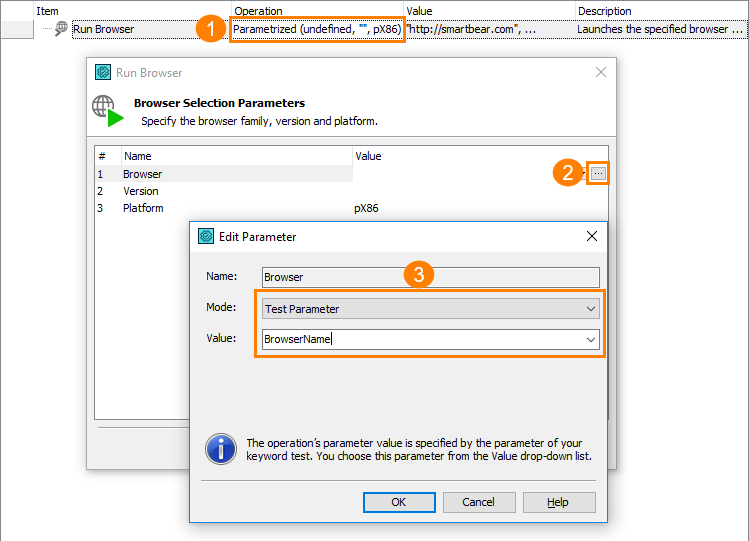
You can also make your web test a data-driven test and read the browser name from an external data file.
For more information, see Parameterizing the Browser for a Test Run.
Remarks
-
If your tests uninstall or install a browser during the run, then you need to call the
Browsers.Refresh
method in your tests to update the Browsers collection. Call this method before the first keyword test operation or script statement that launches a browser, navigates to a web page or performs any other operation with a browser after the installation or uninstallation is over. -
In earlier TestComplete versions, a web browser could be launched as a Tested Application. In the current TestComplete version, new tests are recorded using the Run Browser and
Browsers.Item(...).Run
operations. We recommend updating your existing tests to use these special-purpose operations.
See Also
How To
Preparing Web Browsers
Parameterizing the Browser for a Test Run
Running Tests in Multiple Browsers