TestComplete samples (both built-in and additional) are located in the <Users>\Public\Public Documents\TestComplete 15 Samples folder.
Some file managers display the Public Documents folder as Documents.
TestComplete lets you create and run automated tests for Java applications and applets. This topic explains the basic concepts of Java application testing.
Creating and Recording Tests for Java Applications
About Java Object Identification and Name Mapping
About Support for Java Controls
Using Native Java Methods and Fields in Testing
Supported Java Technologies
TestComplete lets you test Java applications that use the following technologies:
-
Java Runtime Environment (JRE) 7, 8, 11 and 17 (OpenJRE or Oracle JRE)
-
32-bit and 64-bit JRE
-
Java Web Start applications (.jnlp)
-
AWT, SWT, Swing libraries
Note: |
Support for Java applet and Web Start has been removed from Java version 11. |
TestComplete also supports testing of JavaFX applications.
Requirements for Java Testing
-
A license for TestComplete Desktop module.
-
TestComplete can test Java applications out-of-the-box - with no need to recompile the application. In some cases, you may need to edit the JRE configuration files or launch your application with specific command-line arguments. For more information, see:
Creating and Recording Tests for Java Applications
With TestComplete, you can record and play back user actions in Java applications, or you can create tests manually from scratch. Usually, it is easier to record the test first and then modify and enhance the recorded test.
When you record a test, you interact with the tested Java application as an end-user would: navigate through the application’s screens, fill out forms and so on. TestComplete captures all actions you perform in the application and adds them to the test.
A test consists of a sequence of operations that define various interactions with objects in the tested application. For example, in the sample test below you can see that item selection from a combo box is represented by the ClickItem
operation, clicking a button - as the ClickButton
operation, and so on.
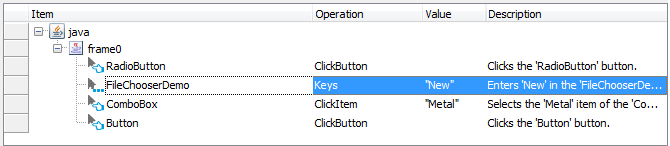
Sample keyword test recorded against a Java application
JavaScript, JScript
function Test1()
{
var fileChooserDemo;
var fileChooserDemo_InsetPanel;
var fileChooserDemo_1;
var panel;
fileChooserDemo = Aliases.java.frame0.RootPane.FileChooserDemo;
fileChooserDemo_InsetPanel = fileChooserDemo.Panel.FileChooserDemo_InsetPanel;
fileChooserDemo_InsetPanel.RadioButton.ClickButton();
fileChooserDemo_1 = fileChooserDemo_InsetPanel.Panel.FileChooserDemo_1;
fileChooserDemo_1.Keys("New");
panel = fileChooserDemo.Panel1;
panel.ComboBox.ClickItem("Metal");
panel.Button.ClickButton();
}
Python
def Test1():
fileChooserDemo = Aliases.java.frame0.RootPane.FileChooserDemo;
fileChooserDemo_InsetPanel = fileChooserDemo.Panel.FileChooserDemo_InsetPanel;
fileChooserDemo_InsetPanel.RadioButton.ClickButton();
fileChooserDemo_1 = fileChooserDemo_InsetPanel.Panel.FileChooserDemo_1;
fileChooserDemo_1.Keys("New");
panel = fileChooserDemo.Panel1;
panel.ComboBox.ClickItem("Metal");
panel.Button.ClickButton();
VBScript
Sub Test1
Dim fileChooserDemo
Dim fileChooserDemo_InsetPanel
Dim fileChooserDemo_1
Dim panel
Set fileChooserDemo = Aliases.java.frame0.RootPane.FileChooserDemo
Set fileChooserDemo_InsetPanel = fileChooserDemo.Panel.FileChooserDemo_InsetPanel
fileChooserDemo_InsetPanel.RadioButton.ClickButton
Set fileChooserDemo_1 = fileChooserDemo_InsetPanel.Panel.FileChooserDemo_1
Call fileChooserDemo_1.Keys("New")
Set panel = fileChooserDemo.Panel1
Call panel.ComboBox.ClickItem("Metal")
panel.Button.ClickButton
End Sub
DelphiScript
procedure Test1;
var fileChooserDemo : OleVariant;
var fileChooserDemo_InsetPanel : OleVariant;
var fileChooserDemo_1 : OleVariant;
var panel : OleVariant;
begin
fileChooserDemo := Aliases.java.frame0.RootPane.FileChooserDemo;
fileChooserDemo_InsetPanel := fileChooserDemo.Panel.FileChooserDemo_InsetPanel;
fileChooserDemo_InsetPanel.RadioButton.ClickButton;
fileChooserDemo_1 := fileChooserDemo_InsetPanel.Panel.FileChooserDemo_1;
fileChooserDemo_1.Keys('New');
panel := fileChooserDemo.Panel1;
panel.ComboBox.ClickItem('Metal');
panel.Button.ClickButton;
end;
C++Script, C#Script
function Test1()
{
var fileChooserDemo;
var fileChooserDemo_InsetPanel;
var fileChooserDemo_1;
var panel;
fileChooserDemo = Aliases["java"]["frame0"]["RootPane"]["FileChooserDemo"];
fileChooserDemo_InsetPanel = fileChooserDemo["Panel"]["FileChooserDemo_InsetPanel"];
fileChooserDemo_InsetPanel["RadioButton"]["ClickButton"]();
fileChooserDemo_1 = fileChooserDemo_InsetPanel["Panel"]["FileChooserDemo_1"];
fileChooserDemo_1["Keys"]("New");
panel = fileChooserDemo["Panel1"];
panel["ComboBox"]["ClickItem"]("Metal");
panel["Button"]["ClickButton"]();
}
The recorded tests can be modified and enhanced in a number of ways to create more flexible and efficient tests. For example, you can:
-
Add new operations, reorder operations and modify their parameters.
-
Delete or disable unneeded operations (for example, superfluous recorded operations).
-
Insert checkpoints for verifying objects and values in the tested application.
-
Create data-driven tests that run multiple test iterations using different sets of data.
Refer to the following topics to learn more about creating and enhancing tests:
Task | See topic… |
---|---|
Creating tests using recording | Recording Tests |
Creating tests manually | Keyword Testing and Scripting |
Simulating user actions | Working With Application Objects and Controls |
Running tests | Running Tests |
Launching applications automatically at the beginning of the test run | Adding Java and JavaFX Applications to the List of Tested Applications, Adding Java Web Start Applications to the List of Tested Applications and Running Tested Applications |
Creating checkpoints for verifying application behavior and state | Checkpoints |
Running multiple test iterations using data from an external file | Data-Driven Testing |
About Test Types
There are two major test formats in TestComplete:
-
Keyword tests - visually configured tests with grid-based editing interface. Best suited for novice users and those without programming knowledge.
-
Scripts - code written in one of the supported scripting languages. May be better suited for advanced users.
You select the test type when creating a test and cannot change it later. However, you can mix keyword tests and scripts within the same test project and call them from each other.
TestComplete also includes additional test types, such as low-level procedures, unit tests, and so on. You can use them to address specific testing needs. However, most automation is typically done using keyword tests and scripts.
About Java Object Identification and Name Mapping
Each object in an application has a number of properties, such as its location, text, type and so on. Some object properties are persistent and unchanging, and therefore can be used to locate objects in applications and differentiate among various objects.
When you record a test, TestComplete captures all windows and controls that you interacted with during the recording session and adds them to the Name Mapping project item (also known as the object repository or GUI map). For each captured object, TestComplete does the following:
-
Selects a set of properties and values that uniquely identify the object in the application and saves them to Name Mapping as the object identification criteria. These properties will be used for locating the object during subsequent test recording, editing and run sessions.
-
Generates an alias (name) that will be used to reference this object in tests. By default, TestComplete generates aliases based on object names defined in the application by developers.
-
Automatically captures and adds images of the mapped objects to the Name Mapping repository. This helps you understand which window or control one or another mapped object matches.
The following image shows sample Name Mapping for a Java application:
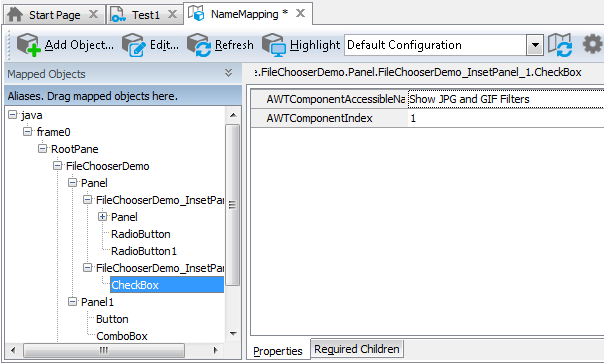
Here, the combobox object is identified using two properties - AWTComponentAccessibleName
and AWTComponentIndex
. These properties holds the object accessible name and the object index as they are defined by the application developers in the application’s source code. However, Java application objects can also be located by other properties, such as their class name, text and so on -- whatever best identifies a specific object.
If needed, you can modify the default Name Mapping generated by TestComplete. For example, you can:
-
Change the identification properties and values to define more robust identification criteria or to reflect major GUI changes in newer versions of the tested application.
-
Rename aliases to more meaningful and descriptive aliases, so that it is easier for you to understand which object is which.
-
Manually add new objects to Name Mapping.
For more information, see Name Mapping.
Keep in mind that the object hierarchy in Name Mapping mirrors the object hierarchy in the tested application. When locating an object, TestComplete takes into account its entire parent hierarchy. If any object in the parent hierarchy cannot be found using the specified property values, the target object cannot be located as well. That is why it is important to select unique and unchanging properties for object identification.
About Support for Java Controls
TestComplete recognizes individual controls of Java applications and lets you interact with them both at design time and during test recording or playback. It also simplifies testing of Java applications’ user interface, since it includes special features used to test the most frequently used Java controls.
For detailed information on support for Java application controls, see Support for Java Applications' Controls.
Using Native Java Methods and Fields in Testing
TestComplete makes visual objects of Java applications available for testing, that is you can recognize these objects, interact with them and work with their native members. TestComplete gets access to all the internal elements of the application. All private, protected and public methods and fields become available to it.
![]() |
TestComplete cannot get access to the internal methods and fields of Java objects if the tested application was launched with the -verbose or -verbose:class command-line argument. |
TestComplete also provides you with the JavaClasses object that allows you to call routines, which reside in Java classes, from scripts. To call these routines, you should first add the desired class name to the project’s Java Bridge options. Classes added to the Java Bridge list, as well as types and type members defined in these classes, become available in scripts and are displayed in the Code Completion window as child objects of the JavaClasses
object. For detailed information on calling routines from Java classes, see Calling Functions From Java Classes.
For detailed information on how to address exposed methods and fields from your tests, see Accessing Native Methods of Java Objects and Addressing Objects in Java Applications.
Viewing Object Properties, Fields and Methods
To see what operations (methods) are available to objects in your tested Java application, as well as the object properties, fields and their values, you can use the Object Browser or Object Spy. The available properties and methods include those provided by TestComplete, as well as native object fields and methods defined by the developers in the application’s source code.
You can view object properties, fields and methods both at design time and at runtime when the test is paused.
For more information on using the Object Browser, see Object Browser and Exploring Applications.
Samples
TestComplete includes a sample test project that demonstrates how to test Swing Java applications:
<TestComplete Samples>\Desktop\Orders\Swing\TCProjects
Note: | If you do not have the sample, download the TestComplete Samples installation package from the support.smartbear.com/testcomplete/downloads/samples page of our website and run it. |
Where to Go Next
For further information about automating tests with TestComplete, refer to the following sections:
See Also
Testing Java Applications
Requirements for Testing Java Applications