You can use TestComplete to test Oracle Forms and Oracle Forms-based applications included in Oracle E-Business Suite (EBS) embedded into a web page or running out-of-browser (launched by using Java Web Start).
Note: |
Support for Java applet and Web Start has been removed from Java version 11. |
You can record and play back user actions on Oracle Forms/EBS. When you are playing back a test, TestComplete repeats all the recorded actions you performed during test recording. You can also create tests manually, from scratch.
TestComplete can access the internals of Oracle applications:
TestComplete recognizes individual GUI elements of Oracle applications as Java objects and provides special properties and methods for simulating user actions on them. It also provides special objects for a number of Oracle controls. In addition, TestComplete provides access to native properties and methods of Oracle Forms controls.
Supported Oracle Forms/EBS Versions
About object identification and name mapping
Supported Oracle Forms/EBS Versions
-
Oracle Forms 12c
-
Oracle Forms 11g
-
Oracle Forms-based applications included in Oracle E-Business Suite (EBS) 12.1 and 12.2
You can test applets that run:
-
On a web page,
-
Out-of-browser, by using Java Web Start.
Note: |
Support for Java applet and Web Start has been removed from Java version 11. |
Requirements
-
A license for the TestComplete Desktop module.
-
The Oracle Forms Control Support plugin. This plugin is installed and enabled automatically.
Note: | Testing of Oracle Forms applications running with Java 9 is not supported. |
To learn how to prepare your testing environment for testing, see Testing Oracle Forms/EBS - Requirements.
Creating and recording tests
You can record and play back user actions on forms, or you can create tests manually from scratch.
When you record a test, you interact with the tested form as an end user would: populate form fields, click buttons and so on. TestComplete captures all the actions you are performing and adds them to your test.
A test consists of a sequence of operations that define various interactions with objects on the tested form. For example, in the sample test below you can see that item selection from a pop list is represented by the ClickItem
operation, clicking a button - by the ClickButton
operation, and so on:
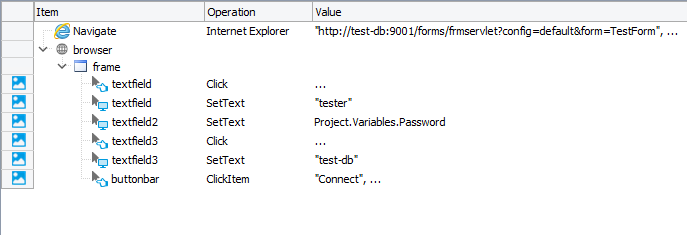
Sample keyword test recorded against an Oracle Forms applet
JavaScript, JScript
function Test()
{
var app = Aliases.jp2launcher;
var formDesktopContainer = app.frame.Main.MDIContainer.FormDesktopContainer;
var ewtContainer = formDesktopContainer.Container;
ewtContainer.textField0.Click(29, 10);
ewtContainer.textField0.Keys("tester");
ewtContainer.textField1.Click(15, 8);
ewtContainer.textField1.Keys("password");
ewtContainer.textField2.Click(22, 10);
ewtContainer.textField2.Keys("test-db");
ewtContainer.buttonBar.ClickItem("Connect");
var drawnPanel = formDesktopContainer.ExtendedFrame.DrawnPanel;
drawnPanel.popList.ClickItem("Item1");
drawnPanel.button.ClickButton(69, 10);
}
Python
def Test():
app = Aliases.jp2launcher
formDesktopContainer = app.frame.Main.MDIContainer.FormDesktopContainer
ewtContainer = formDesktopContainer.Container
ewtContainer.textField0.Click(29, 10)
ewtContainer.textField0.Keys('tester')
ewtContainer.textField1.Click(15, 8)
ewtContainer.textField1.Keys('password')
ewtContainer.textField2.Click(22, 10)
ewtContainer.textField2.Keys('test-db')
ewtContainer.buttonBar.ClickItem('Connect')
drawnPanel = formDesktopContainer.ExtendedFrame.DrawnPanel
drawnPanel.popList.Click(23, 13)
drawnPanel.button.Click(69, 10)
VBScript
Sub Test
Dim app
Dim formDesktopContainer
Dim ewtContainer
Dim drawnPanel
Set app = Aliases.jp2launcher
Set formDesktopContainer = app.Main.MDIContainer.FormDesktopContainer
Set ewtContainer = formDesktopContainer.Container
Call ewtContainer.textField0.Click(29, 10)
Call ewtContainer.textField0.Keys("tester")
Call ewtContainer.textField1.Click(15, 8)
Call ewtContainer.textField1.Keys("password")
Call ewtContainer.textField2.Click(22, 10)
Call ewtContainer.textField2.Keys("test-db")
Call ewtContainer.buttonBar.ClickItem("Connect")
Set drawnPanel = formDesktopContainer.ExtendedFrame.DrawnPanel
Call drawnPanel.popList.ClickItem("Item1")
Call drawnPanel.button.ClickButton
End Sub
DelphiScript
procedure Test();
var app, formDesktopContainer, ewtContainer, drawnPanel;
begin
app := Aliases.jp2launcher;
formDesktopContainer := app.frame.Main.MDIContainer.FormDesktopContainer;
ewtContainer := formDesktopContainer.Container;
ewtContainer.textField0.Click(29, 10);
ewtContainer.textField0.Keys('tester');
ewtContainer.textField1.Click(15, 8);
ewtContainer.textField1.Keys('password');
ewtContainer.textField2.Click(22, 10);
ewtContainer.textField2.Keys('test-db');
ewtContainer.buttonBar.ClickItem('Connect');
drawnPanel := formDesktopContainer.ExtendedFrame.DrawnPanel;
drawnPanel.popList.ClickItem('Item1');
drawnPanel.button.ClickButton;
end;
C++Script, C#Script
function Test()
{
var app = Aliases["jp2launcher"];
var formDesktopContainer = app["frame"]["Main"]["MDIContainer"]["FormDesktopContainer"];
var ewtContainer = formDesktopContainer["Container"];
ewtContainer["textField0"]["Click"](29, 10);
ewtContainer["textField0"]["Keys"]("tester");
ewtContainer["textField1"]["Click"](15, 8);
ewtContainer["textField1"]["Keys"]("password");
ewtContainer["textField2"]["Click"](22, 10);
ewtContainer["textField2"]["Keys"]("test-db");
ewtContainer["buttonBar"]["ClickItem"]("Connect");
var drawnPanel = formDesktopContainer["ExtendedFrame"]["DrawnPanel"];
drawnPanel["popList"]["ClickItem"]("Item1");
drawnPanel["button"]["ClickButton"]();
}
You can modify and enhance the recorded test in a number of ways to create more flexible and efficient tests. For example, you can:
-
Add new operations, reorder operations and modify their parameters.
-
Delete or disable unneeded operations (for example, superfluous operations).
-
Insert checkpoints for verifying objects and values in the tested application.
-
Create data-driven tests that run multiple test iterations using different sets of data.
Refer to the following topics to learn more about creating and enhancing tests:
Task | See topic… |
---|---|
Creating tests using recording | Recording Tests |
Creating tests manually | Keyword Testing and Scripting |
Running tests | Running Tests |
Launching applications automatically at the beginning of the test run (for Oracle Forms applications that run out-of-browser, by using Java Web Start) | Adding Java Web Start Applications to the List of Tested Applications and Running Tested Applications |
Creating checkpoints for verifying application behavior and state | Checkpoints |
Running multiple test iterations using data from an external file | Data-Driven Testing |
About object identification and name mapping
Each object in Oracle Forms/EBS application has a number of properties, such as its location, text, type and so on. Some object properties are persistent and unchanging. TestComplete uses them to locate objects in applications and differentiate them among other objects.
When you record a test, TestComplete captures all the controls you interacted with during the recording session and adds them to the Name Mapping project item (also known as the object repository or GUI map). For each captured object, TestComplete does the following:
-
Selects a set of properties and values that uniquely identify the object and saves them to the Name Mapping repository as the object’s identification criteria. TestComplete will use these properties for locating the object during subsequent test recording, editing and run sessions.
-
Generates an alias (name) that it will use to refer to this object in tests. By default, TestComplete generates aliases based on object names that the application developers defined in the application's source code.
The following image shows sample Name Mapping for Oracle Forms controls:
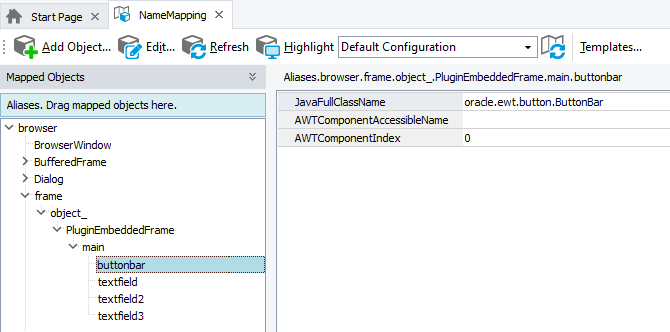
Note: | To make aliases for Oracle Forms objects shorter, TestComplete does not include intermediate objects (containers, scroll boxes, draw panels) in the alias tree. |
Here, the popList object is identified by using the AWTComponentName
property. The property holds the object name as the application developers defined it in the application’s source code.
You can also use other properties to locate objects in Oracle Forms, for example, their class name, text, and so on - whatever identifies the needed object best.
If needed, you can modify the default Name Mapping generated by TestComplete. For example, you can:
-
Change the identification properties and values to define more robust identification criteria or to reflect major GUI changes in newer versions of the tested application.
-
Rename aliases to more meaningful and descriptive aliases, so that it is easier for you to understand which object is which.
-
Manually add new objects to Name Mapping.
For more information, see Name Mapping.
Keep in mind that the object hierarchy in Name Mapping mirrors the object hierarchy in the tested application. When locating an object, TestComplete takes into account its entire parent hierarchy. If TestComplete cannot find any object in the parent hierarchy using the specified property values, it cannot find the target object as well. That is why it is important to select unique and unchanging properties for object identification.
About support for Oracle Forms/EBS controls
TestComplete recognizes individual controls of Oracle Forms/EBS. You can interact with them both at design time and during test recording and playback. TestComplete also provides extended support for a number of Oracle Forms controls. You use program objects that TestComplete provides for the controls, their methods and properties to automate user actions on the controls, get their state and so on. To learn more, see Support for Oracle Forms Controls.
You can also automate Oracle Forms controls by calling their native properties and methods (these are the same properties and methods that are available to the application developers). See Accessing Native Methods of Oracle Forms/EBS.
Viewing object properties, fields and methods
To see what operations (methods) are available to objects in your tested Oracle applications, as well as the object properties, fields and their values, you can use the Object Browser or Object Spy. The available properties and methods include those provided by TestComplete, as well as native object fields and methods defined by the developers in the application’s source code.
You can view object properties, fields and methods both at design time and at runtime when the test is paused.
For more information on using the Object Browser, see Object Browser and Exploring Applications.