![]() |
Information in this topic applies to generic desktop applications (.NET, WPF, Delphi and others). |
TestComplete can handle events of COM objects and ActiveX controls in any running application. This topic explains how you can create event handlers for these events in TestComplete.
This topic explains how to handle events in Microsoft Outlook. You can handle events only if your application is Open or a COM server. Microsoft Outlook is a COM server, so you do not need to prepare it for testing. For information on preparing your application for testing, see the following topics:
Preparing Desktop Applications for Testing
To handle an external event:
Prepare the Test Project
To handle events, add the Events project item to your test project:
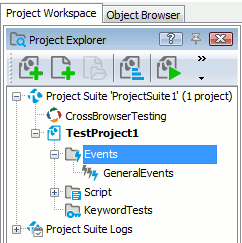
The Events item is created in new projects by default if the Generate the default content checkbox is selected in the Create Project dialog. If the item is created by default, it contains an Event control named GeneralEvents.
If your project does not include this item, you should add it to the project:
-
Right-click the project item in the Project Explorer and select Add | New Item. This will open the Create Project Item dialog.
-
In the dialog, select the Events project item, specify its location in the edit box and press OK to save the changes. The Events project item will appear in the Advanced project folder. It will contain an Event control named GeneralEvents.
Add an Event to the Event Control
By default, the GeneralEvents control contains only TestComplete events. TestComplete does not import external events. To add these events to the list:
-
Right-click the GeneralEvents control in the Project Explorer and select Edit from the context menu. TestComplete will show the Event Control editor in the Workspace panel.
-
In the Source object field of the editor, specify the type library that contains the declaration of Outlook COM objects. Click the ellipsis button in the Source object field and select Microsoft Outlook <VersionNumber> Object Library in the Select ActiveX Library dialog.
-
After you specify the library in the “Source object” edit field, TestComplete will display Outlook events in the Available Events list box.
Note: By default, the Available Events list does not display events with the Hidden attribute. To make these events visible, enable the Show hidden members option in the Engines - General options dialog. -
Expand the Outlook.Application item and drag the required event from the Available Events list to the Events to Handle list box (it is displayed on the right). You can also double-click the event in the Available Events list.
Create an Event Handler
After creating the event, you need to create an event handler script or keyword test for it. You can find an example of how to send email via Microsoft Outlook in the Office sample. To handle the event in your script:
-
In order for the event handler to work, you need to first attach it to the Outlook object. You can do this by using the
AttachTo
method. After the test is over, detach the Event control by using theDetachFrom
method:JavaScript
var objOutlook;
...
objOutlook = getActiveXObject("Outlook.Application");
Events.GeneralEvents.AttachTo(objOutlook);
...
// Run tests
...
Events.GeneralEvents.DetachFrom(objOutlook);JScript
var objOutlook;
...
objOutlook = Sys.OleObject("Outlook.Application");
Events.GeneralEvents.AttachTo(objOutlook);
...
// Run tests
...
Events.GeneralEvents.DetachFrom(objOutlook);Python
... objOutlook = Sys.OleObject["Outlook.Application"] Events.GeneralEvents.AttachTo(objOutlook) ... # Run tests ... Events.GeneralEvents.DetachFrom(objOutlook)
VBScript
Dim objOutlook
...
Set objOutlook = Sys.OleObject("Outlook.Application")
Events.GeneralEvents.AttachTo(objOutlook)
...
' Run your tests
...
Events.GeneralEvents.DetachFrom(objOutlook)DelphiScript
var
objOutlook : OleVariant;
begin
...
objOutlook := Sys.OleObject('Outlook.Application');
Events.GeneralEvents(objOutlook);
...
// Run tests
...
Events.GeneralEvents.DetachFrom(objOutlook);
end;C++Script, C#Script
var objOutlook;
...
objOutlook = Sys["OleObject"]("Outlook.Application");
Events["GeneralEvents"]["AttachTo"](objOutlook);
...
// Run tests
...
Events["GeneralEvents"]["DetachFrom"](objOutlook); -
Select an event in the Events to Handle list, for example the
ItemSend
event. This event will trigger when an email is sent during test run. -
Click the New button that is displayed in the Event Handler cell. TestComplete displays the New Event Handler dialog where you can specify a unit and name for the event handling routine. In this dialog, you can also specify a keyword test name if you want to use a keyword test for event handling.
After you click OK in this dialog, TestComplete will create the event handler and display it in the Code Editor (or in the Keyword Test editor if you are creating an event handling keyword test).
-
Modify the event handler. This script will be executed every time the event triggers during the test run.
JavaScript, JScript
function GeneralEvents_NewMail(Sender)
{
Log.Message("The message has been sent!");
}Python
def GeneralEvents_NewMail(Sender): Log.Message("The message has been sent!")
VBScript
Sub GeneralEvents_NewMail(Sender)
Log.Message "The message has been sent!"
End SubDelphiScript
procedure GeneralEvents_NewMail(Sender);
begin
Log.Message('The message has been sent!');
end;C++Script, C#Script
function GeneralEvents_NewMail(Sender)
{
Log["Message"]("The message has been sent!");
}
See Also
Event Control
Handling Events - Overview
Creating Event Handlers for TestComplete Events
Typical Event Handler Tasks
Creating Event Handlers in TestComplete