With TestComplete, you can test web applications on real iOS and Android devices. To do this, you need to install the SmartBearBrowser application on your device. This browser allows recording, creating, and running mobile web tests with TestComplete. This topic explains how to install SmartBearBrowser and use it for testing.
Note that a mobile browser is essentially a hybrid mobile application, that is, an application that uses the WebView control to display web content. So, all concepts of a hybrid mobile application also apply to mobile web testing.
Requirements
-
A license for the TestComplete Mobile module. A license for the Web module is not necessary.
-
One of the supported iOS or Android devices.
-
Your device and the TestComplete computer must be prepared for mobile testing.
-
You need to install SmartBearBrowser on your device (see below). This is the browser you will use for testing. Regular browsers like Mobile Safari or Chrome are not supported.
-
The device must have an Internet connection to access web sites.
About SmartBearBrowser
SmartBearBrowser is a simple WebView-based browser for iOS and Android devices. You can find it in the following locations:
Android
<TestComplete 15>\Bin\Extensions\Android\SmartBearBrowser.apk
The .apk file is not instrumented, but it will be instrumented automatically when you start it from TestComplete. Install SmartBearBrowser.apk on your Android device and start testing right away.
iOS
<TestComplete 15>\Bin\Extensions\iOS\SmartBearBrowser_NotPatched.ipa
You must have an Apple developer account and Mac.
Instrument and sign this IPA file using your developer certificate. Then, you can deploy and run the application on your test devices as any other iOS tested app.
Testing Websites in SmartBearBrowser
SmartBearBrowser is a basic browser that contains only the page, address bar and navigation controls. The browser can show only one page at a time and does not support tabs. For example, links with target="_blank" rel="noopener noreferrer"
are opened in the same window, replacing the previously opened page.
Testing web pages in the SmartBearBrowser mobile browser is similar to testing hybrid mobile applications. Some common tasks are explained below.
![]() SmartBearBrowser for iOS |
![]() SmartBearBrowser for Android |
Running SmartBearBrowser
SmartBearBrowser can be launched automatically from tests.
Assuming you have added SmartBearBrowser to TestedApps, you can run it as any other tested application. When recording tests, you can run it from the Recording toolbar:
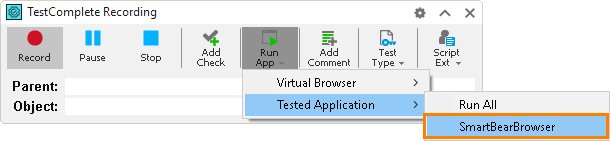
In tests, you can run SmartBearBrowser by using the Run TestedApp keyword test operation or the TestedApps.app_name.Run()
scripting method.
Running iOS or Android version of SmartBearBrowser depending on device type
Add both iOS and Android versions of SmartBearBrowser to TestedApps and name them SmartBearBrowser_iOS and SmartBearBrowser_Android. Now, you can use this code to run the appropriate version of SmartBearBrowser:
JavaScript, JScript
Mobile.SetCurrent("Nexus 6");
TestedApps.Items("SmartBearBrowser_" + Mobile.Device().OSType).Run();
Python
def Test():
Mobile.SetCurrent("Nexus 6");
TestedApps.Items["SmartBearBrowser_" + Mobile.Device().OSType].Run();
VBScript
Call Mobile.SetCurrent("Nexus 6")
TestedApps.Items("SmartBearBrowser_" & Mobile.Device.OSType).Run
DelphiScript
Mobile.SetCurrent('Nexus 6');
TestedApps.Items('SmartBearBrowser_' + Mobile.Device().OSType).Run();
C++Script, C#Script
Mobile["SetCurrent"]("Nexus 6");
TestedApps["Items"]("SmartBearBrowser_" + Mobile["Device"]["OSType"]).Run();
In keyword tests, you can use If...Then to check the device’s OSType
(iOS or Android) and run the corresponding version of SmartBearBrowser:

Accessing WebView and Page Objects
To create cross-platform tests that work on both Android and iOS devices, you need to design your tests with the platform differences in mind. One such thing is the “path” to the Page
object in the SmartBearBrowser. You can use techniques like Name Mapping and the Find
methods to handle differences in the object hierarchy.
The following image shows the SmartBearBrowser object hierarchy. Like other hybrid mobile applications, SmartBearBrowser contains a WebView
control with a child Page
object. WebView
provides methods to navigate web pages - ToUrl(...)
, goBack()
and goForward()
. The Page
object provides access to web page elements.
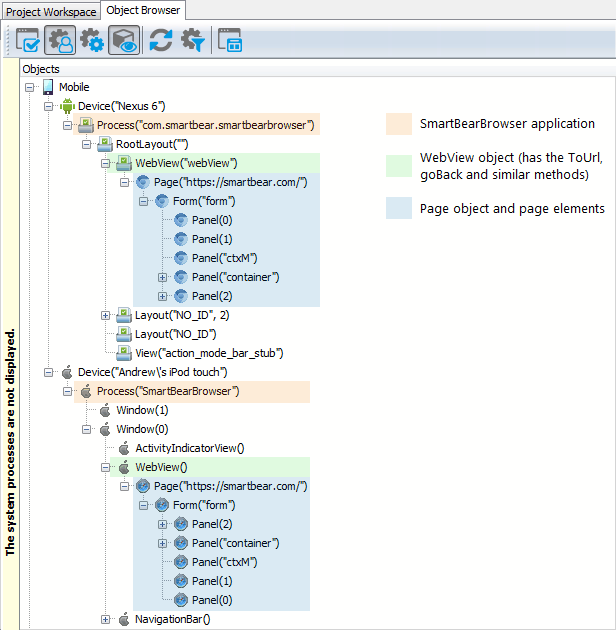
Using Name Mapping
To create cross-platform tests using Name Mapping, configure your name map as follows. This way, the aliases will work for both iOS and Android versions of SmartBearBrowser.
Note: This is not a default configuration. You will need to edit the name map created during the recording, or map the objects manually.
Mapped Objects:
Mobile
+- Device (DeviceName = *)
+- Browser (ProcessName = *SmartBearBrowser)
+- WebView (ObjectType = WebView, Extended Find option enabled)
+- pageMyPage1 (ObjectType = Page, ObjectIdentifier = page URL)
+- pageMyPage2 (ObjectType = Page, ObjectIdentifier = page URL)
+- ...
Here, the *SmartBearBrowser wildcard name matches both the iOS and Android process names, SmartBearBrowser and com.smartbear.smartbearbrowser.
Note that any objects between Browser and WebView should be excluded from the map.
Aliases:
Device
+- Browser
+- WebView
+- pageMyPage1
+- pageMyPage2
+- ...
Using Find Methods
You can also create cross-platform tests by using scripts with no Name Mapping. In this case, you can use wildcards and the FindChild
method to access objects in SmartBearBrowser uniformly on iOS and Android. Typical code would look like this:
JavaScript, JScript
function Test()
{
Mobile.SetCurrent("Nexus 6");
var browser = Mobile.Device().Process("*SmartBearBrowser");
var webView = browser.FindChild("ObjectType", "WebView", 2);
var page = webView.ToUrl("http://example.com/");
// Do something ...
}
Python
def Test():
Mobile.SetCurrent("Nexus 6")
browser = Mobile.Device().Process("*SmartBearBrowser")
webView = browser.FindChild("ObjectType", "WebView", 2)
page = webView.ToUrl("http://example.com/")
# Do something ...
VBScript
Sub Test
Dim browser, webView, page
Call Mobile.SetCurrent("Nexus 6")
Set browser = Mobile.Device.Process("*SmartBearBrowser")
Set webView = browser.FindChild("ObjectType", "WebView", 2)
Set page = webView.ToUrl("http://example.com/")
' Do something ...
End Sub
DelphiScript
procedure Test;
var browser, webView, page;
begin
Mobile.SetCurrent('Nexus 6');
browser := Mobile.Device.Process("*SmartBearBrowser");
webView := browser.FindChild('ObjectType', 'WebView', 2);
page := webView.ToUrl('http://example.com/');
// Do something ...
end;
C++Script, C#Script
function Test()
{
Mobile["SetCurrent"]("Nexus 6");
var browser = Mobile["Device"]["Process"]("*SmartBearBrowser");
var webView = browser["FindChild"]("ObjectType", "WebView", 2);
var page = webView["ToUrl"]("http://example.com/");
// Do something ...
}
Navigating to Web Pages
Finding Objects on Web Pages
Running Test on Multiple Devices in a Loop
Limitations and Known Issues
Common:
-
All limitations specific to hybrid mobile applications also apply to SmartBearBrowser.
-
Typing a URL into the address bar and clicking the Go button are recorded as the text input and button click rather than
WebView.ToUrl(url)
. You can edit the recorded tests to use theToUrl
method instead. -
The
Page
object and page elements have fewer DOM properties than in desktop browsers, and DOM methods are not available. This is by design and is done for performance reasons.
iOS:
-
All limitations of iOS test recording also apply to SmartBearBrowser.
-
For iOS 13 or later, UIWebView controls are deprecated. For this reason, we do not guarantee that TestComplete will work correctly with applications that use such controls.
Android:
-
To record text input, click the text field and type the text on your computer keyboard, not on the device’s onscreen keyboard. TestComplete cannot record text input directly from Android devices.
-
SmartBearBrowser uses the standard Android WebView component to display web pages. WebView uses the WebKit rendering engine in Android versions up to 4.3 and the Chromium (Blink) engine in Android 4.4 and later. So, on Android versions up to 4.3, SmartBearBrowser might display pages differently than Chrome does.
-
See also Possible Issues With Android Applications (Legacy).
FAQ
Is there an analog of Browsers.Item(...).Run(url) for SmartBearBrowser?
In mobile web tests, you would run SmartBearBrowser as a tested application, and then call the WebView.ToUrl(url)
method to navigate to a URL.
How do I specify the initial URL for the test?
Assuming that SmartBearBrowser is running, you call the ToUrl(url)
method of the WebView
object to navigate to a URL.
What is the user agent of SmartBearBrowser?
Go to http://useragentstring.com in SmartBearBrowser and see what this site says.