![]() |
Flash Player has reached end of life on December 31, 2020. Support for Flash and Flex applications is now deprecated in TestComplete and will be removed in a future release. |
The information in this topic applies to web tests that locate web objects by using internal identification properties provided by TestComplete and run in local environments.
This topic describes how to address controls and objects of Flash and Flex applications when the Microsoft Active Accessibility (MSAA) engine is used for testing.
Addressing Application’s Main Window
Enabling Microsoft Active Accessibility (MSAA) for Flash and Flex applications provides moderate access to the tested application’s internals - TestComplete recognizes objects according to accessibility information provided by the application and names objects according to this info.
After you have obtained the container web page, you can get a Flash or Flex application’s window. A Flash or Flex application appears in the object hierarchy as an object named Object(Index)
, where Index is the application’s Id
or Name
attribute or its index among sibling objects of the same type. The image below shows how an application exposed via Microsoft Active Accessibility is displayed in the Object Browser:
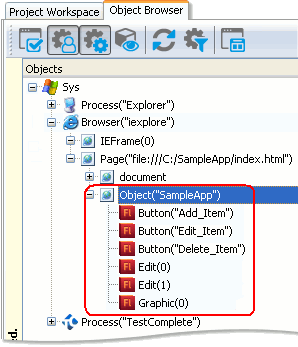
The objects of a Flash or Flex application exposed via the MSAA engine are marked with the glyph in the Object Browser panel.
The main window of a Flash or Flex application is a child object of the container web page. It appears in the object hierarchy as the object named Object(AppIdentifier)
, where AppIdentifier is the application’s Id
or Name
attribute or its index among sibling objects of the same type.
Addressing Application’s Child Objects
Once you have obtained a Flash or Flex application window, you can access its controls and objects, get and set their property values and call their methods. TestComplete recognizes Flash or Flex application objects relying on the accessibility information provided by the application and names the objects according to this info. The MSAA object addressing has the following specifics:
- The object type is determined by the object’s accessibility role. A list of recognizable object types is given in the MSAA Object Types by Names topic.
- If there is no appropriate accessibility role for a control type, then it is treated as the
Panel
type. - Depending on the object type, the accessibility name can be the text displayed by the object, the object’s caption or a static text that labels the object.
- If there are several objects with the same accessibility roles and names in a parent container, TestComplete addresses the object by the accessibility name plus its index among siblings of the same type (the
ControlIndexInGroup
property value). - If the object does not have an accessibility name specified, then TestComplete names it using the object type and its index within the parent container.
When addressing an object, it is possible to use wildcard characters (* and ?) to indicate variable parts of the object’s accessibility name. The accessibility names are case-insensitive.
An object name consists of the type name, which corresponds to the object’s accessibility role, and the accessibility name. For example:
Button("Ok")
RadioButton("Option_1")
Edit(0)
Panel("Calendar_View_November_2009")
Here, Button, RadioButton, Edit and Panel are the object types, which correspond to the objects’ accessibility roles; “Ok”, “Option_1” and “Calendar_View_November_2009” are the accessible names of the appropriate objects; 0 is the object’s index among sibling objects of the same type. Indexes are used to address the Text and Edit objects and objects that have no accessible name specified.
For more information about object naming in applications that support Active Accessibility, see Addressing Objects of MSAA Open Applications.
Available Object Members
Exposed objects of Flash and Flex applications contain the following members:
-
Properties and methods common for all tested objects:
Name
,Exists
,Find
and others. -
Properties, methods and actions of
onscreen
objects:Click
,Keys
,Drag
and others. -
Properties and methods added by the MSAA engine (see Methods and Properties Added to MSAA Objects):
Caption
,DoDefaultAction
and others. However, control-specific properties and methods, likeSelectedItem
,FocusedTab
and others, are not available for exposed Flash objects. - Also, to wait for an object that is exposed by the MSAA engine, TestComplete adds a number of
Wait
methods to parent objects:WaitPanel
,WaitTable
,WaitListItem
and so on. See methods description to learn about their syntax and usage examples.
Flash example
You can use the above-mentioned properties and methods exposed by TestComplete to obtain the object’s contents and state and simulate various user actions on the object. Note that native properties and methods of exposed Flash and Flex objects are not accessible if you use the MSAA engine for testing.
The following code snippet demonstrates how to obtain the Add Item button of the sample Flash application displayed in the image above and simulate a click on that button:
JavaScript, JScript
var page, app;
Browsers.Item(btIexplorer).Run("file:///C:/SampleApp/index.html");
var page = Sys.Browser("iexplore").Page("*");
app = page.Object("SampleApp");
app.Button("Add Item").Click();
Python
Browsers.Item[btIexplorer].Run("file:///C:/SampleApp/index.html");
page = Sys.Browser("iexplore").Page("*");
app = page.Object("SampleApp");
app.Button("Add Item").Click();
VBScript
Dim page, app
Browsers.Item(btIexplorer).Run("file:///C:/SampleApp/index.html")
Set page = Sys.Browser("iexplore").Page("*")
Set app = page.Object("SampleApp")
app.Button("Add Item").Click
DelphiScript
var page, app;
begin
Browsers.Item[btIexplorer].Run('file:///C:/SampleApp/index.html');
page := Sys.Browser('iexplore').Page('*');
app := page.Object('SampleApp');
app.Button('Add Item').Click;
C++Script, C#Script
var page, app;
Browsers["Item"](btIexplorer)["Run"]("file:///C:/SampleApp/index.html");
var page = Sys["Browser"]("iexplore")["Page"]("*");
app = page["Object"]("SampleApp");
app["Button"]("Add Item")["Click"]();
For more information on the ways to work with application objects, see Working With Application Objects and Controls and Simulating User Actions.
Flex example
You can use the above-mentioned properties and methods exposed by TestComplete to obtain the object’s contents and state and simulate various user actions on the object. The following example demonstrates how you can interact with controls in a Flex application exposed via Microsoft Active Accessibility.
JavaScript, JScript
function Test()
{
// Obtain the browser and the wrapper HTML page
Browsers.Item(btIexplorer).Run("file:///C:/SampleApp/SampleApp.html");
var browser = Sys.Browser("iexplore");
// Obtain the tested Flex application
var flexapp = browser.Page("*").Object("SampleApp");
// Interact with controls
flexapp.RadioButton("Option_1").Click();
var edit = flexapp.Edit(0);
edit.Keys("Some Text");
// Wait for the checkbox and mark it on success
var cbCacheData = flexapp.WaitCheckBox("Cache_data", 1000);
if (cbCacheData.Exists) flexapp.CheckBox("Cache_data").Checked = true;
flexapp.Button("Ok").ClickButton();
}
Python
def Test():
# Obtain the browser and the wrapper HTML page
Browsers.Item[btIExplorer].Run("file:///C:/SampleApp/SampleApp.html");
browser = Sys.Browser("iexplore");
# Obtain the tested Flex application
flexapp = browser.Page("*").Object("SampleApp");
# Interact with controls
flexapp.RadioButton("Option_1").Click();
edit = flexapp.Edit(0);
edit.Keys("Some Text");
# Wait for the checkbox and mark it on success
cbCacheData = flexapp.WaitCheckBox("Cache_data", 1000);
if (cbCacheData.Exists):
flexapp.CheckBox("Cache_data").Checked = True;
flexapp.Button("Ok").ClickButton();
VBScript
Sub Test
Dim browser, flexapp, edit, cbCacheData
' Obtain the browser and the wrapper HTML page
Browsers.Item(btIexplorer).Run("file:///C:/SampleApp/SampleApp.html")
Set browser = Sys.Browser("iexplore")
' Obtain the tested Flex application
Set flexapp = browser.Page("*").Object("SampleApp")
' Interact with controls
Call flexapp.RadioButton("Option_1").Click()
Set edit = flexapp.Edit(0)
Call edit.Keys("Some Text")
' Wait for the checkbox and mark it on success
Set cbCacheData = flexapp.WaitCheckBox("Cache_data", 1000)
If (cbCacheData.Exists) Then
flexapp.CheckBox("Cache_data").Checked = True
End If
Call flexapp.Button("Ok").ClickButton()
End Sub
DelphiScript
procedure Test();
var browser, flexapp, edit, cbCacheData;
begin
// Obtain the browser and the wrapper HTML page
Browsers.Item(btIexplorer).Run('file:///C:/SampleApp/SampleApp.html');
browser := Sys.Browser('iexplore');
// Obtain the tested Flex application
flexapp := browser.Page('*').Object('SampleApp');
// Interact with controls
flexapp.RadioButton('Option_1').Click();
edit := flexapp.Edit(0);
edit.Keys('Some Text');
// Wait for the checkbox and mark it on success
cbCacheData := flexapp.WaitCheckBox('Cache_data', 1000);
if (cbCacheData.Exists) then flexapp.CheckBox('Cache_data').Checked := true;
flexapp.Button('Ok').ClickButton();
end;
C++Script, C#Script
function Test()
{
var browser, flexapp, edit, cbCacheData;
// Obtain the browser and the wrapper HTML page
Browsers["Item"](btIexplorer)["Run"]("file:///C:/SampleApp/SampleApp.html");
var browser = Sys["Browser"]("iexplore");
// Obtain the tested Flex application
var flexapp = browser["Page"]("*")["Object"]("SampleApp");
// Interact with controls
flexapp["RadioButton"]("Option_1")["Click"]();
var edit = flexapp["Edit"](0);
edit["Keys"]("Some Text");
// Wait for the checkbox and mark it on success
var cbCacheData = flexapp["WaitCheckBox"]("Cache_data", 1000);
if (cbCacheData["Exists"]) flexapp["CheckBox"]("Cache_data")["Checked"] = true;
flexapp["Button"]("Ok")["ClickButton"]();
}
See Also
Testing Flash and Flex Applications via the MSAA Engine
Preparing Flash and Flex Applications for Testing via the MSAA Engine
Testing Flash and Flex Applications