![]() |
The information below concerns legacy mobile tests that work with mobile devices connected to the local computer. For new mobile tests, we recommend using the newer cloud-compatible approach. |
In your tests, you often need to check the state of a control in your tested Android application. For example, you may need to check whether a specific control is enabled and is visible on screen. Also, you may need to check whether the control displays the needed data.
This topic describes how to check the state or data of tested objects in Android Open Applications.
Basic Concepts
If your mobile application is Open, to check whether a property or a method of a tested object returns the expected value, you can use special functionality provided by TestComplete - property checkpoints. They let you check whether a property or a set of properties of the tested application's object meet the specified conditions.
To check whether a control that displays data in a tabular form contains the correct data, you can use table checkpoints. Table checkpoints compare the actual data displayed by a control in your mobile application with the baseline data stored in your project. The baseline table must be stored in the Stores | Tables collection of your project. For information on how to work with table checkpoints, see About Table Checkpoints.
To check whether an object is displayed correctly, you can use region checkpoints. The region checkpoints obtain the actual image of the specified control and verify the image against the expected image of the object stored in the Stores | Regions collection in your project. Note that the region checkpoints allow you to set verification parameters - you can specify pixels to be treated as transparent and areas to be ignored during the verification. You can also command TestComplete to ignore slight differences between images. For more information on how region checkpoints work, see How Image Comparison Works.
You can create checkpoints during test recording and at design time when editing your test. For information on how to create checkpoints, see appropriate topics in the About Checkpoints section.
Checking an Object's State and Data in Keyword Tests
To check an object’s state in an Android Open Application in your keyword test, use the Property Checkpoint operation. For example, the Property Checkpoint operation in the keyword test shown in the image below checks whether a button is enabled.
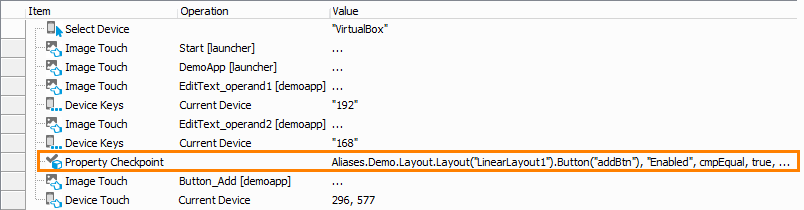
Alternatively, you can use the If Object operation to quickly check an object’s state - whether the object exists, whether it is visible on screen or enabled. You can also create a custom verification procedure by using the If Then operation.
To check tabular data displayed by controls in your mobile application, use the Table Checkpoint operation. To check if an object in your mobile application is displayed correctly, use the Region Checkpoint operation.
The image below displays the Region Checkpoint operation that verifies that the control in a tested mobile application displays the correct data.
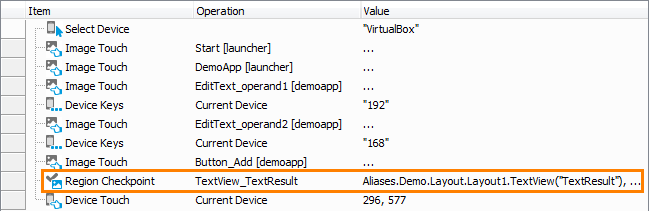
You can also use the Compare Picture operation or call methods TestComplete provides for image comparison (see Alternatives to Region Checkpoints for more information).
Checking an Object's State and Data in Scripts
To check the state of an object in script tests, you can use the aqObject.CheckProperty
method (this method is a scripting analog of the Property Checkpoint operation). It allows you to verify an object’s property against a condition:
JavaScript, JScript
function UseCheckProperty()
{
Mobile.SetCurrent("MyDevice");
// ...
var btnObj = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").Button("addBtn");
aqObject.CheckProperty(btnObj, "Enabled", 0, true);
}
Python
def UseCheckProperty():
Mobile.SetCurrent("MyDevice");
# ...
btnObj = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").Button("addBtn");
aqObject.CheckProperty(btnObj, "Enabled", 0, True);
VBScript
Sub UseCheckProperty
Mobile.SetCurrent("MyDevice")
' ...
Set btnObj = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").Button("addBtn")
Call aqObject.CheckProperty(btnObj, "Enabled", 0, True)
End Sub
DelphiScript
procedure UseCheckProperty();
var btnObj;
begin
Mobile.SetCurrent('MyDevice');
// ...
btnObj := Mobile.Device('MyDevice').Process('smartbear.example.demoapp').Layout('NO_ID').Button('addBtn');
aqObject.CheckProperty(btnObj, 'Enabled', 0, true);
end;
C++Script, C#Script
function UseCheckProperty()
{
Mobile["SetCurrent"]("MyDevice");
// ...
var btnObj = Mobile["Device"]("MyDevice")["Process"]("smartbear.example.demoapp")["Layout"]("NO_ID")["Button"]("addBtn");
aqObject["CheckProperty"](btnObj, "Enabled", 0, true);
}
Alternatively, you can use the if … then
statement in your script test to check an object’s state:
JavaScript, JScript
function UseObjectNameCheck()
{
Mobile.SetCurrent("MyDevice");
// ...
var result = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").TextView("TextResult");
if (result.ControlText == "100")
{
// The property value equals the baseline value
}
else
{
// The property value does not equal the baseline value
}
}
Python
def UseObjectNameCheck():
Mobile.SetCurrent("MyDevice");
# ...
result = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").TextView("TextResult");
if (result.ControlText == "100"):
# The property value equals the baseline value
else:
# The property value does not equal the baseline value
VBScript
Sub UseObjectNameCheck
Mobile.SetCurrent("MyDevice")
' ...
Set result = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").TextView("TextResult")
If result.ControlText = "100" Then
' The property value equals the baseline value
Else
' The property value does not equal the baseline value
End If
End Sub
DelphiScript
procedure UseObjectNameCheck();
var result;
begin
Mobile.SetCurrent('MyDevice');
// ...
result := Mobile.Device('MyDevice').Process('smartbear.example.demoapp').Layout('NO_ID').TextView('TextResult');
if result.ControlText = '100' then
// The property value equals the baseline value
else
// The property value does not equal the baseline value
end;
C++Script, C#Script
function UseObjectNameCheck()
{
Mobile["SetCurrent"]("MyDevice");
// ...
var result = Mobile["Device"]("MyDevice")["Process"]("smartbear.example.demoapp")["Layout"]("NO_ID")["TextView"]("TextResult");
if (result.ControlText == "100")
{
// The property value equals the baseline value
}
else
{
// The property value does not equal the baseline value
}
}
To check tabular data displayed by a control in a mobile application (for example, by the Android GridView control), use the Tables.StoredTableName.Check
method, which is a scripting analog of the Table Checkpoint operation:
JavaScript, JScript
function UseStoredTableNameCheck()
{
Mobile.SetCurrent("MyDevice");
// ...
var gridView = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").GridView("gridView_Sample");
Tables.BaselineTable.Check(gridView);
}
Python
def UseStoredTableNameCheck():
Mobile.SetCurrent("MyDevice");
# ...
gridView = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").GridView("gridView_Sample");
Tables.BaselineTable.Check(gridView);
VBScript
Sub UseStoredTableNameCheck
Mobile.SetCurrent("MyDevice")
// ...
Set gridView = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").GridView("gridView_Sample")
Call Tables.BaselineTable.Check(gridView)
End Sub
DelphiScript
procedure UseStoredTableNameCheck();
var gridView;
begin
Mobile.SetCurrent('MyDevice');
// ...
gridView := Mobile.Device('MyDevice').Process('smartbear.example.demoapp').Layout('NO_ID').GridView('gridView_Sample');
Tables.BaselineTable.Check(gridView);
end;
C++Script, C#Script
function UseStoredTableNameCheck()
{
Mobile["SetCurrent"]("MyDevice");
// ...
var gridView = Mobile["Device"]("MyDevice")["Process"]("smartbear.example.demoapp")["Layout"]("NO_ID")["GridView"]("gridView_Sample");
Tables["BaselineTable"]["Check"](gridView);
}
To check whether an object in an Android Open Application is displayed correctly in script tests, use the Regions.RegionCheckpoint.Check
method (it is a scripting analog of the Region Checkpoint operation):
JavaScript, JScript
function UseRegionCheckpointCheck()
{
Mobile.SetCurrent("MyDevice");
// ...
var textView = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").TextView("TextResult");
Regions.TextResult.Check(textView);
}
Python
def UseRegionCheckpointCheck():
Mobile.SetCurrent("MyDevice");
# ...
textView = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").TextView("TextResult");
Regions.TextResult.Check(textView);
VBScript
Sub UseRegionCheckpointCheck
Mobile.SetCurrent("MyDevice")
' ...
Set textView = Mobile.Device("MyDevice").Process("smartbear.example.demoapp").Layout("NO_ID").TextView("TextResult")
Call Regions.TextResult.Check(textView)
End Sub
DelphiScript
procedure UseRegionCheckpointCheck();
var textView;
begin
Mobile.SetCurrent('MyDevice');
// ...
textView := Mobile.Device('MyDevice').Process('smartbear.example.demoapp').Layout('NO_ID').TextView('TextResult');
Regions.TextResult.Check(textView);
end;
C++Script, C#Script
function UseRegionCheckpointCheck()
{
Mobile["SetCurrent"]("MyDevice");
// ...
var textView = Mobile["Device"]("MyDevice")["Process"]("smartbear.example.demoapp")["Layout"]("NO_ID")["TextView"]("TextResult");
Regions["TextResult"]["Check"](textView);
}
Alternatively, you can use the Picture
method to obtain the object’s image and then use one of the following methods to check whether the object is displayed correctly:
-
Regions.Compare
,Picture.Compare
andPicture.Difference
- Use these methods to compare the actual image of the object with the image stored in the Regions collection or with an arbitrary image. -
Regions.Find
,Regions.FindRegion
andPicture.Find
- Use these methods to check whether the image of a tested object contains specified image or vice versa to check whether the image of the tested object belongs to the specified image.
See Also
Checking Object State (Legacy)
Testing Android Applications (Legacy)
Property Checkpoints
Object Checkpoints
Table Checkpoints
Region Checkpoints
Checking Object State and Data in Image-Based Tests