The information in this topic applies to web tests that implement the classic approach (rely on Name Mapping and run in local environments). In cross-platform web tests, you connect to a device lab and launch a needed web browser at the beginning of your test run (see Connect to Device Lab and Launch Web Browser). After the test run is over, the web browser is closed automatically, and the testing session is terminated. You do not have to close your web browser manually. Doing so will terminate your remote testing session prematurely.
To run an automated test successfully, you need to make sure that the initial conditions of each test run correspond to the initial conditions you had when creating the test. Therefore, you may want to return your tested application to its initial state before each new simulation starts. You may want to do it at the end or at the beginning of each test iteration.
Typically, when testing web applications, you open a web page in a web browser and simulate user actions on the page. We recommend that you close the browser instance when the test iteration is over. Otherwise, your test will launch a new browser instance while the previous instance of the web browser is running, and you may end up with multiple redundant web browser instances, which may cause ambiguous object recognition, and your tests will fail. In addition, cookies or browser cache that the existing browser instance uses may store the information specific to the open session that may influence your test.
That is why we recommend that you configure your tests to close the current web browser automatically before each new test iteration starts.
In Keyword Tests
To close a browser window
To close a web browser, you can call the Close
method of the BrowserWindow
object that corresponds to the main window of the current web browser. The method has the WaitTimeout parameter that pauses the test run for the specified time or until the window closes. If any of your browsers closes slowly, to avoid starting a new test before the browser closes, set the parameter value accordingly.
To add the method to your keyword test, use On-Screen Action or Call Object Method that calls the Close
method of the browser window.
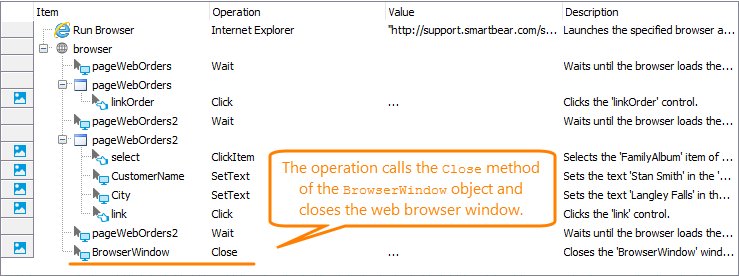
To close a browser process
Another way to close the browser is to call the Close
method of the corresponding Browser
process. This method closes the browser process directly. For browsers with a multi-process architecture (like Google Chrome or Internet Explorer 11), the Browser.Close
method closes the main browser process along with the child browser processes. The method’s WaitTimeout parameter pauses the test execution for the specified time or until the main and auxiliary browser processes are closed.
To add the method to your test, use the Call Object Method operation that calls the Close
method of the browser process.
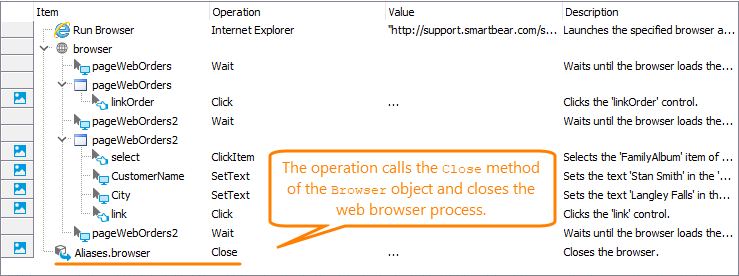
To close multiple browsers
To close all the instances of running browsers, check for them within the While Loop operation. For each browser instance, call the Close
method:

In Scripts
To close a browser window
To close a web browser window from scripts, you can use the BrowserWindow.Close
method. It closes the appropriate browser window. Typically, a browser has only one window. If there is a single browser window, closing the window will result in closing the browser.
The method uses the WaitTimeout parameter that specifies the amount of time to wait before closing the browser window. Its default value is 2000 milliseconds.
To close a browser process
To close a web browser from script, you can use the Browser.Close
method. It closes the browser process directly. In addition, it also closes all browser windows and all auxiliary browser processes.
The method has the WaitTimeout parameter that specifies the amount of time to wait before closing the browser process. Its default value is 60000 milliseconds.
JavaScript, JScript
function Test1()
{
// Run the web browser and obtain a web page
Browsers.Item(btIExplorer).Run("http://smartbear.com/");
var browser = Sys.Browser();
var page = browser.Page("*smartbear.com*")
...
// Test the page
...
// Close the web browser
browser.Close();
}
Python
def Test1():
# Run the web browser and obtain a web page
Browsers.Item[btIExplorer].Run("http://smartbear.com/");
browser = Sys.Browser();
page = browser.Page("*smartbear.com*")
# ...
# Test the page
# ...
# Close the web browser
browser.Close();
VBScript
Sub Test1
Dim page, browser
' Run the web browser and obtain a web page
Call Browsers.Item(btIExplorer).Run("http://smartbear.com/")
Set browser = Sys.Browser
Set page = browser.Page("*smartbear.com*")
...
' Test the page
...
' Close the web browser
browser.Close
End Sub
DelphiScript
procedure Test1;
var browser, page;
begin
// Run the web browser and obtain a web page
Browsers.Item(btIExplorer).Run('http://smartbear.com/');
browser := Sys.Browser;
page := browser.Page('*smartbear.com*');
...
// Test the page
...
// Close the web browser
browser.Close();
end;
C++Script, C#Script
function Test1()
{
// Run the web browser and obtain a web page
Browsers["Item"](btIExplorer)["Run"]("http://smartbear.com/");
var browser = Sys["Browser"]();
var page = browser.Page("https://smartbear.com/")
...
// Test the page
...
// Close the web browser
browser["Close"]();
}
To close multiple browser processes
To close all the instances of running browsers, check for them within the while
loop and close each instance:
JavaScript, JScript
function Close()
{
…
while (Sys.WaitBrowser().Exists)
Sys.WaitBrowser().Close();
…
}
Python
procedure Close():
…
while (Sys.WaitBrowser().Exists):
Sys.WaitBrowser().Close()
…
VBScript
Sub Close()
While Sys.WaitBrowser().Exists
Call Sys.WaitBrowser().Close()
Wend
…
End Sub
DelphiScript
procedure Close();
begin
…
while Sys.WaitBrowser().Exists do
Sys.WaitBrowser().Close();
…
end;
C++Script, C#Script
function Close()
{
…
while ( Sys["WaitBrowser"]()["Exists"] )
Sys["WaitBrowser"]()["Close"]();
…
}
See Also
How To
Check if Browser Is Running
Launch Web Browsers
Running Tests in Multiple Browsers
Checking the Current Browser
Preparing Web Browsers