TestComplete samples (both built-in and additional) are located in the <Users>\Public\Public Documents\TestComplete 15 Samples folder.
Some file managers display the Public Documents folder as Documents.
The information in this topic applies both to cross-platform and classic web tests.
TestComplete supports common JavaScript popups and browser dialogs used in web applications, such as alerts, confirmations, input prompts, basic authentication dialogs and others. TestComplete detects these dialogs in all the supported web browsers and lets you automate them as part of your tests.
Although popup dialogs are implemented differently in different browsers, TestComplete identifies them and their UI elements in a browser-independent fashion, which helps with cross-browser and cross-platform testing.
Alerts
An alert box is displayed using the JavaScript alert
function. It is a simple message box with a text message and the OK button.
![]() An alert box in Internet Explorer |
![]() An alert box in Firefox |
TestComplete records and plays back interactions with JavaScript alert boxes using the Alert
test object and its child UI objects. It also lets you get the alert text using the Alert.Message
property.
The following example demonstrates how you can automate an alert box:
Web (Cross-Platform)

JavaScript, JScript
function Alert_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers.CurrentBrowser.Navigate(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/");
var link = page.FindElement("//a[contains(text(),'Show Alert')]");
link.Click();
Log.Message("Alert text: " + page.Alert.Message);
page.Alert.Button("OK").Click();
}
Python
def Alert_Demo():
url = "http://secure.smartbearsoftware.com/samples/testcomplete14/dialogs/"
Browsers.CurrentBrowser.Navigate(url)
page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
link = page.FindElement("//a[contains(text(),'Show Alert')]")
link.Click()
Log.Message("Alert text: " + page.Alert.Message)
page.Alert.Button("OK").Click()
VBScript
Sub Alert_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/"
Browsers.CurrentBrowser.Navigate(url)
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
Set link = page.FindElement("//a[contains(text(),'Show Alert')]")
link.Click
Log.Message("Alert text: " & page.Alert.Message)
page.Alert.Button("OK").Click
End Sub
DelphiScript
procedure Alert_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/';
Browsers.CurrentBrowser.Navigate(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs/');
link := page.FindElement('//a[contains(text(),''Show Alert'')]');
link.Click();
Log.Message('Alert text: ' + page.Alert.Message);
page.Alert.Button('OK').Click();
end;
C++Script, C#Script
function Alert_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers["CurrentBrowser"]["Navigate"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs/");
var link = page["FindElement"]("//a[contains(text(),'Show Alert')]");
link["Click"]();
Log["Message"]("Alert text: " + page["Alert"]["Message"]);
page["Alert"]["Button"]("OK")["Click"]();
}
Classic

JavaScript, JScript
function Alert_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers.Item(btIExplorer).Run(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/");
var link = page.FindChild("contentText", "Show Alert", 10);
link.Click();
Log.Message("Alert text: " + page.Alert.Message);
page.Alert.Button("OK").Click();
}
Python
def Alert_Demo():
url = "http://secure.smartbearsoftware.com/samples/testcomplete14/dialogs/"
Browsers.Item[btIExplorer].Run(url)
page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
link = page.FindChild("contentText", "Show Alert", 10)
link.Click()
Log.Message("Alert text: " + page.Alert.Message)
page.Alert.Button("OK").Click()
VBScript
Sub Alert_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/"
Browsers.Item(btIExplorer).Run(url)
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
Set link = page.FindChild("contentText", "Show Alert", 10)
link.Click
Log.Message("Alert text: " & page.Alert.Message)
page.Alert.Button("OK").Click
End Sub
DelphiScript
procedure Alert_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/';
Browsers.Item(btIExplorer).Run(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs/');
link := page.FindChild('contentText', 'Show Alert', 10);
link.Click();
Log.Message('Alert text: ' + page.Alert.Message);
page.Alert.Button('OK').Click();
end;
C++Script, C#Script
function Alert_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers["Item"](btIExplorer)["Navigate"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs/");
var link = page["FindChild"]("contentText", "Show Alert", 10);
link["Click"]();
Log["Message"]("Alert text: " + page["Alert"]["Message"]);
page["Alert"]["Button"]("OK")["Click"]();
}
Confirmations
A confirmation box is displayed using the JavaScript confirm
function. It contains a text message and two buttons, OK and Cancel.
![]() A confirmation box in Internet Explorer |
![]() A confirmation box in Chrome |
TestComplete records and plays back interactions with confirmation boxes using the Confirm
test object. It also lets you get the confirmation message text using the Confirm.Message
property.
The following example demonstrates how you can automate a confirmation box:
Web (Cross-Platform)

JavaScript, JScript
function Confirm_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers.CurrentBrowser.Navigate(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/");
var link = page.FindElement("//a[contains(text(),'Show Confirm')]");
link.Click();
Log.Message("Message text: " + page.Confirm.Message);
page.Confirm.Button("OK").Click();
}
Python
VBScript
Sub Confirm_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/"
Browsers.CurrentBrowser.Navigate(url)
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
Set link = page.FindElement("//a[contains(text(),'Show Confirm')]")
link.Click
Log.Message("Message text: " & page.Confirm.Message)
page.Confirm.Button("OK").Click
End Sub
DelphiScript
procedure Confirm_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/';
Browsers.CurrentBrowser.Navigate(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs/');
link := page.FindElement('//a[contains(text(),''Show Confirm'')]');
link.Click();
Log.Message('Message text: ' + page.Confirm.Message);
page.Confirm.Button('OK').Click();
end;
C++Script, C#Script
function Confirm_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers["CurrentBrowser"]["Navigate"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs/");
var link = page["FindElement"]("//a[contains(text(),'Show Confirm')]");
link["Click"]();
Log["Message"]("Message text: " + page["Confirm"]["Message"]);
page["Confirm"]["Button"]("OK")["Click"]();
}
Classic

JavaScript, JScript
function Confirm_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers.Item(btIExplorer).Run(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/");
var link = page.FindChild("contentText", "Show Confirm", 10);
link.Click();
Log.Message("Message text: " + page.Confirm.Message);
page.Confirm.Button("OK").Click();
}
Python
VBScript
Sub Confirm_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/"
Browsers.Item(btIExplorer).Run(url)
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
Set link = page.FindChild("contentText", "Show Confirm", 10)
link.Click
Log.Message("Message text: " & page.Confirm.Message)
page.Confirm.Button("OK").Click
End Sub
DelphiScript
procedure Confirm_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/';
Browsers.Item(btIExplorer).Run(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs/');
link := page.FindChild('contentText', 'Show Confirm', 10);
link.Click();
Log.Message('Message text: ' + page.Confirm.Message);
page.Confirm.Button('OK').Click();
end;
C++Script, C#Script
function Confirm_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers["Item"](btIExplorer)["Navigate"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs/");
var link = page["FindChild"]("contentText", "Show Confirm", 10);
link["Click"]();
Log["Message"]("Message text: " + page["Confirm"]["Message"]);
page["Confirm"]["Button"]("OK")["Click"]();
}
Prompts
A prompt box is displayed using the JavaScript prompt
function. It contains a message asking the user to enter a value, an input field and two buttons, OK and Cancel.
![]() A prompt box in Chrome |
TestComplete records and plays back interactions with prompt boxes using the Prompt
test object and its child UI objects. It also lets you get the prompt text using the Prompt.Message
property.
The following examples demonstrate how you can automate a prompt box:
Web (Cross-Platform)
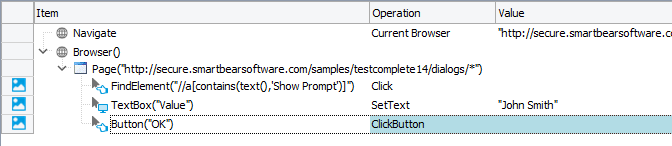
JavaScript, JScript
function Prompt_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers.CurrentBrowser.Navigate(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/");
var link = page.FindElement("//a[contains(text(),'Show Prompt')]");
link.Click();
Log.Message("Message text: " + page.Prompt.Message);
Log.Message("Default value: " + page.Prompt.Value);
page.Prompt.Value = "John Smith";
// You can also enter the value using SetText method of the input field object
// page.Prompt.Textbox("Value").SetText("John Smith");
page.Prompt.Button("OK").Click();
}
Python
def Prompt_Demo():
url = "http://secure.smartbearsoftware.com/samples/testcomplete14/dialogs/"
Browsers.CurrentBrowser.Navigate(url)
page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
link = page.FindElement("//a[contains(text(),'Show Prompt')]")
link.Click()
Log.Message("Message text: " + page.Prompt.Message)
Log.Message("Default value: " + page.Prompt().Value)
page.Prompt().Value = "John Smith"
# You can also enter the value using SetText method of the input field object
# page.Prompt.Textbox("Value").SetText("John Smith")
page.Prompt.Button("OK").Click()
VBScript
Sub Prompt_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/"
Browsers.CurrentBrowser.Navigate(url)
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
Set link = page.FindElement("//a[contains(text(),'Show Prompt')]")
link.Click
Log.Message("Message text: " & page.Prompt.Message)
Log.Message("Default value: " & page.Prompt.Value)
page.Prompt.Value = "John Smith"
' You can also enter the value using SetText method of the input field object
' page.Prompt.Textbox("Value").SetText("John Smith")
page.Prompt.Button("OK").Click
End Sub
DelphiScript
procedure Prompt_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/';
Browsers.CurrentBrowser.Navigate(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs/');
link := page.FindElement('//a[contains(text(),''Show Prompt'')]');
link.Click();
Log.Message('Message text: ' + page.Prompt.Message);
Log.Message('Default value: ' + page.Prompt.Value);
page.Prompt.Value := 'John Smith';
// You can also enter the value using SetText method of the input field object
// page.Prompt.Textbox('Value').SetText('John Smith');
page.Prompt.Button('OK').Click();
end;
C++Script, C#Script
function Prompt_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers["CurrentBrowser"]["Navigate"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs/");
var link = page["FindElement"]("//a[contains(text(),'Show Prompt')]");
link["Click"]();
Log["Message"]("Message text: " + page["Prompt"]["Message"]);
Log["Message"]("Default value: " + page["Prompt"]["Value"]);
page["Prompt"]["Value"] = "John Smith";
// You can also enter the value using SetText method of the input field object
// page["Prompt"]["Textbox"]("Value")["SetText"]("John Smith");
page["Prompt"]["Button"]("OK")["Click"]();
}
Classic
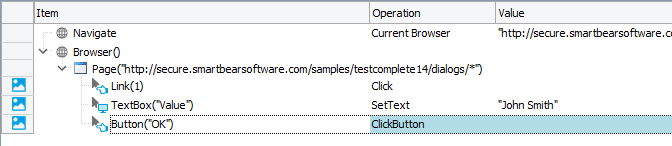
JavaScript, JScript
function Prompt_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers.Item(btIExplorer).Run(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/");
var link = page.FindChild("contentText", "Show Prompt", 10);
link.Click();
Log.Message("Message text: " + page.Prompt.Message);
Log.Message("Default value: " + page.Prompt.Value);
page.Prompt.Value = "John Smith";
// You can also enter the value using SetText method of the input field object
// page.Prompt.Textbox("Value").SetText("John Smith");
page.Prompt.Button("OK").Click();
}
Python
def Prompt_Demo():
url = "http://secure.smartbearsoftware.com/samples/testcomplete14/dialogs/"
Browsers.Item[btIExplorer].Run(url)
page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
link = page.FindChild("contentText", "Show Prompt", 10)
link.Click()
Log.Message("Message text: " + page.Prompt.Message)
Log.Message("Default value: " + page.Prompt().Value)
page.Prompt().Value = "John Smith"
# You can also enter the value using SetText method of the input field object
# page.Prompt.Textbox("Value").SetText("John Smith")
page.Prompt.Button("OK").Click()
VBScript
Sub Prompt_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/"
Browsers.Item(btIExplorer).Run(url)
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs/")
Set link = page.FindChild("contentText", "Show Prompt", 10)
link.Click
Log.Message("Message text: " & page.Prompt.Message)
Log.Message("Default value: " & page.Prompt.Value)
page.Prompt.Value = "John Smith"
' You can also enter the value using SetText method of the input field object
' page.Prompt.Textbox("Value").SetText("John Smith")
page.Prompt.Button("OK").Click
End Sub
DelphiScript
procedure Prompt_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/';
Browsers.Item(btIExplorer).Run(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs/');
link := page.FindChild('contentText', 'Show Prompt', 10);
link.Click();
Log.Message('Message text: ' + page.Prompt.Message);
Log.Message('Default value: ' + page.Prompt.Value);
page.Prompt.Value := 'John Smith';
// You can also enter the value using SetText method of the input field object
// page.Prompt.Textbox('Value').SetText('John Smith');
page.Prompt.Button('OK').Click();
end;
C++Script, C#Script
function Prompt_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/";
Browsers["Item"](btIExplorer)["Navigate"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs/");
var link = page["FindChild"]("contentText", "Show Prompt", 10);
link["Click"]();
Log["Message"]("Message text: " + page["Prompt"]["Message"]);
Log["Message"]("Default value: " + page["Prompt"]["Value"]);
page["Prompt"]["Value"] = "John Smith";
// You can also enter the value using SetText method of the input field object
// page["Prompt"]["Textbox"]("Value")["SetText"]("John Smith");
page["Prompt"]["Button"]("OK")["Click"]();
}
Form Resubmission Confirmations
When the user reloads a web page that contains a filled out form previously submitted to the server, the web browser asks the user whether to resubmit the form.
![]() The Resend information message in Internet Explorer |
![]() The Resend information message in Chrome |
TestComplete records and plays back interactions with form resubmission confirmations using the Confirm
test object. The “Resend” button is identified as Button("OK")
(or buttonOk
if Name Mapping is used), the “Cancel” button - as Button("Cancel")
(or buttonCancel
in Name Mapping). The button object names are predefined and do not depend on the UI languages used in the browser or operating system.
The following example demonstrates how you can interact with a form resubmission confirmation and cancel the action:
Web (Cross-Platform)
Note: The F5 shortcut may not be supported on a platform where your cross-platform web test is running. To reload the page, we use the location.reload()
method of the DOM object. To learn more about accessing the DOM document object, see Accessing DOM document Object. To learn more about calling scripts on web pages, see Run JavaScript on Web Pages.

JavaScript, JScript
function Resubmit_Demo()
{
var url = "https://www.whatsmyua.info/";
Browsers.CurrentBrowser.Navigate(url);
var browser = Sys.Browser();
var page = browser.Page("*whatsmyua*");
// Submit the form
var button = page.FindElement(".go");
button.Click();
page.Wait();
// Refresh the page and choose not to resubmit the form
page.contentDocument.Script.eval("location.reload()");
page.Confirm.Button("Cancel").Click();
}
Python
def Resubmit_Demo():
url = "https://www.whatsmyua.info/"
Browsers.CurrentBrowser.Navigate(url)
browser = Sys.Browser()
page = browser.Page("*whatsmyua*")
# Submit the form
button = page.FindElement(".go")
button.Click()
page.Wait()
# Refresh the page and choose not to resubmit the form
page.contentDocument.Script.eval("location.reload()")
page.Confirm.Button("Cancel").Click()
VBScript
Sub Resubmit_Demo()
url = "https://www.whatsmyua.info/"
Browsers.CurrentBrowser.Navigate(url)
Set browser = Sys.Browser()
Set page = browser.Page("*whatsmyua*")
' Submit the form
Set button = page.FindElement(".go")
button.Click
page.Wait
' Refresh the page and choose not to resubmit the form
page.contentDocument.Script.eval("location.reload()")
page.Confirm.Button("Cancel").Click
End Sub
DelphiScript
procedure Resubmit_Demo();
var url, browser, page, button;
begin
url := 'https://www.whatsmyua.info/';
Browsers.CurrentBrowser.Navigate(url);
browser := Sys.Browser();
page := browser.Page('*whatsmyua*');
// Submit the form
button := page.FindElement('.go');
button.Click();
page.Wait();
// Refresh the page and choose not to resubmit the form
page.contentDocument.Script.eval('location.reload()');
page.Confirm.Button('Cancel').Click();
end;
C++Script, C#Script
function Resubmit_Demo()
{
var url = "https://www.whatsmyua.info/";
Browsers["CurrentBrowser"]["Navigate"](url);
var browser = Sys["Browser"]();
var page = browser["Page"]("*whatsmyua*");
// Submit the form
var button = page["FindElement"](".go");
button["Click"]();
page["Wait"]();
// Refresh the page and choose not to resubmit the form
page["contentDocument"]["Script"]["eval"]("location.reload()");
page["Confirm"]["Button"]("Cancel")["Click"]();
}
Classic
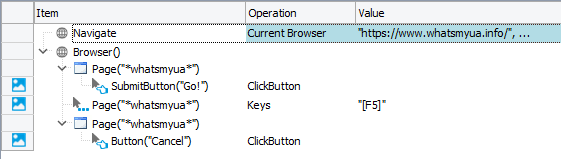
JavaScript, JScript
function Resubmit_Demo()
{
var url = "https://www.whatsmyua.info/";
Browsers.Item(btIExplorer).Run(url);
var browser = Sys.Browser();
var page = browser.Page("*whatsmyua*");
// Submit the form
var button = page.FindChild("ObjectIdentifier", "Go!", 5);
button.Click();
page.Wait();
// Refresh the page and choose not to resubmit the form
page.Keys("[F5]");
page.Confirm.Button("Cancel").Click();
}
Python
def Resubmit_Demo():
url = "https://www.whatsmyua.info/"
Browsers.Item[btIExplorer].Run(url)
browser = Sys.Browser()
page = browser.Page("*whatsmyua*")
# Submit the form
button = page.FindChild("ObjectIdentifier", "Go!", 5)
button.Click()
page.Wait()
# Refresh the page and choose not to resubmit the form
page.Keys("[F5]")
page.Confirm.Button("Cancel").Click()
VBScript
Sub Resubmit_Demo()
url = "https://www.whatsmyua.info/"
Browsers.Item(btIExplorer).Run url
Set browser = Sys.Browser()
Set page = browser.Page("*whatsmyua*")
' Submit the form
Set button = page.FindChild("ObjectIdentifier", "Go!", 5)
button.Click
page.Wait
' Refresh the page and choose not to resubmit the form
page.Keys("[F5]")
page.Confirm.Button("Cancel").Click
End Sub
DelphiScript
procedure Resubmit_Demo();
var url, browser, page, button;
begin
url := 'https://www.whatsmyua.info/';
Browsers.Item(btIExplorer).Run(url);
browser := Sys.Browser();
page := browser.Page('*whatsmyua*');
// Submit the form
button := page.FindChild('ObjectIdentifier', 'Go!', 5);
button.Click();
page.Wait();
// Refresh the page and choose not to resubmit the form
page.Keys('[F5]');
page.Confirm.Button('Cancel').Click();
end;
C++Script, C#Script
function Resubmit_Demo()
{
var url = "https://www.whatsmyua.info/";
Browsers["Item"](btIExplorer)["Run"](url);
var browser = Sys["Browser"]();
var page = browser["Page"]("*whatsmyua*");
// Submit the form
var button = page["FindChild"]("ObjectIdentifier", "Go!", 5);
button["Click"]();
page["Wait"]();
// Refresh the page and choose not to resubmit the form
page["Keys"]("[F5]");
page["Confirm"]["Button"]("Cancel")["Click"]();
}
OnBeforeUnload Messages
Web pages that handle the onbeforeunload
event display a confirmation message when they attempt to close the page or navigate to another page.
![]() The OnBeforeUnload message in Internet Explorer |
![]() The OnBeforeUnload message in Chrome |
TestComplete identifies and records interactions with the OnBeforeUnload message using the Confirm
test object. The “Leave page” option is identified as Button("OK")
(or buttonOk
if Name Mapping is used) and the “Stay on page” option - as Button("Cancel")
(buttonCancel
in Name Mapping). The button object names are predefined and do not depend on the UI language used in the browser or operating system.
The following example demonstrates how you can select the “Leave page” option in the OnBeforeUnload dialog box:
Web (Cross-Platform)

JavaScript, JScript
function OnBeforeUnload_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#";
Browsers.CurrentBrowser.Navigate(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs*");
var link = page.FindElement("//a[contains(text(),'http://smartbear.com/')]");
link.Click();
Log.Message("OnBeforeUnload message: " + page.Confirm.Message);
page.Confirm.Button("OK").Click(); // Click "Leave page"
}
Python
def OnBeforeUnload_Demo():
url = "http://secure.smartbearsoftware.com/samples/testcomplete14/dialogs/#"
Browsers.CurrentBrowser.Navigate(url)
page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs*")
link = page.FindElement("//a[contains(text(),'http://smartbear.com/')]")
link.Click()
Log.Message("OnBeforeUnload message: " + page.Confirm.Message)
page.Confirm.Button("OK").Click() # Click "Leave page"
VBScript
Sub OnBeforeUnload_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#"
Browsers.CurrentBrowser.Navigate(url)
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs*")
Set link = page.FindElement("//a[contains(text(),'http://smartbear.com/')]")
link.Click
Log.Message("OnBeforeUnload message: " & page.Confirm.Message)
page.Confirm.Button("OK").Click ' Click "Leave page"
End Sub
DelphiScript
procedure OnBeforeUnload_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#';
Browsers.CurrentBrowser.Navigate(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs*');
link := page.FindElement('//a[contains(text(),''http://smartbear.com/'')]');
link.Click();
Log.Message('OnBeforeUnload message: ' + page.Confirm.Message);
page.Confirm.Button('OK').Click(); // Click 'Leave page'
end;
C++Script, C#Script
function OnBeforeUnload_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#";
Browsers["CurrentBrowser"]["Navigate"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs*");
var link = page["FindElement"]("//a[contains(text(),'http://smartbear.com/')]");
link["Click"]();
Log["Message"]("OnBeforeUnload message: " + page["Confirm"]["Message"]);
page["Confirm"]["Button"]("OK")["Click"](); // Click "Leave page"
}
Classic

JavaScript, JScript
function OnBeforeUnload_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#";
Browsers.Item(btIExplorer).Run(url);
var page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs*");
var link = page.FindChild("contentText", "http://smartbear.com/", 10);
link.Click();
Log.Message("OnBeforeUnload message: " + page.Confirm.Message);
page.Confirm.Button("OK").Click(); // Click "Leave page"
}
Python
def OnBeforeUnload_Demo():
url = "http://secure.smartbearsoftware.com/samples/testcomplete14/dialogs/#"
Browsers.Item[btIExplorer].Run(url)
page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs*")
link = page.FindChild("contentText", "http://smartbear.com/", 10)
link.Click()
Log.Message("OnBeforeUnload message: " + page.Confirm.Message)
page.Confirm.Button("OK").Click() # Click "Leave page"
VBScript
Sub OnBeforeUnload_Demo()
url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#"
Browsers.Item(btIExplorer).Run url
Set page = Sys.Browser().Page("*smartbear*/samples/testcomplete*/dialogs*")
Set link = page.FindChild("contentText", "http://smartbear.com/", 10)
link.Click
Log.Message("OnBeforeUnload message: " & page.Confirm.Message)
page.Confirm.Button("OK").Click ' Click "Leave page"
End Sub
DelphiScript
procedure OnBeforeUnload_Demo();
var url, page, link;
begin
url := 'http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#';
Browsers.Item[btIExplorer].Run(url);
page := Sys.Browser().Page('*smartbear*/samples/testcomplete*/dialogs*');
link := page.FindChild('contentText', 'http://smartbear.com/', 10);
link.Click();
Log.Message('OnBeforeUnload message: ' + page.Confirm.Message);
page.Confirm.Button('OK').Click(); // Click 'Leave page'
end;
C++Script, C#Script
function OnBeforeUnload_Demo()
{
var url = "http://secure.smartbearsoftware.com/samples/testcomplete15/dialogs/#";
Browsers["Item"](btIExplorer)["Run"](url);
var page = Sys["Browser"]()["Page"]("*smartbear*/samples/testcomplete*/dialogs*");
var link = page["FindChild"]("contentText", "http://smartbear.com/", 10);
link["Click"]();
Log["Message"]("OnBeforeUnload message: " + page["Confirm"]["Message"]);
page["Confirm"]["Button"]("OK")["Click"](); // Click "Leave page"
}
Login Dialogs
This dialog is not supported in cross-platform web tests. For a workaround, see below.
If a web application uses Basic authentication, the browser displays a login dialog box where the user can enter the user name and password for accessing the application.
![]() The login dialog in Internet Explorer |
![]() The login dialog in Chrome |
TestComplete records and plays back actions with login dialogs using the Login
test object and its child UI objects. You can get and set the user name using the Login.UserName
property; to set a password, use the Login.Password
property.
![]() |
TestComplete does not record the login and password if they are populated by the browser’s password manager. You need to manually enter the login and password in order for them to be recorded. |
![]() |
The Login.Password property has write-only access. You cannot use it to get the password text. |
Below is a sample test that automates the login dialog:
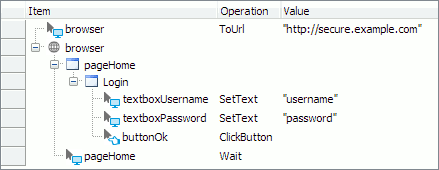
JavaScript, JScript
function Test()
{
var url = "http://secure.example.com";
Browsers.Item(btIExplorer).Run(url);
var page = Sys.Browser("*").Page(url);
page.Login.UserName = "username";
page.Login.Password = "password";
// Or you can enter the user name and password using the text box object methods
// page.Login.TextBox("UserName").SetText("username");
// page.Login.TextBox("Password").SetText("password");
page.Login.Button("OK").Click();
page.Wait();
}
Python
def Test():
url = "https://login.yahoo.com"
Browsers.Item[btIExplorer].Run(url)
page = Sys.Browser("*").Page(url)
page.Login().UserName = "username"
page.Login().Password = "password"
# Or you can enter the user name and password using the text box object methods
# page.Login.TextBox("UserName").SetText("username")
# page.Login.TextBox("Password").SetText("password")
page.Login().Button("OK").Click()
page.Wait()
VBScript
Sub Test
Dim url, page
url = "http://secure.example.com"
Browsers.Item(btIExplorer).Run url
Set page = Sys.Browser("*").Page(url)
page.Login.UserName = "username"
page.Login.Password = "password"
' Or you can enter the user name and password using the text box object methods
' page.Login.TextBox("UserName").SetText("username")
' page.Login.TextBox("Password").SetText("password")
page.Login.Button("OK").Click
page.Wait
End Sub
DelphiScript
procedure Test;
var url, page;
begin
url := 'http://secure.example.com/';
Browsers.Item[btIExplorer].Run(url);
page := Sys.Browser('*').Page(url);
page.Login.UserName := 'username';
page.Login.Password := 'password';
// Or you can enter the user name and password using the text box object methods
// page.Login.TextBox('UserName').SetText('username');
// page.Login.TextBox('Password').SetText('password');
page.Login.Button('OK').Click;
page.Wait();
end;
C++Script, C#Script
function Test()
{
var url = "http://secure.example.com";
Browsers["Item"](btIExplorer)["Run"](url);
var page = Sys["Browser"]("*")["Page"](url);
page["Login"]["UserName"] = "username";
page["Login"]["Password"] = "password";
// Or you can enter the user name and password using the text box object methods
// page["Login"]["TextBox"]("UserName")["SetText"]("username");
// page["Login"]["TextBox"]("Password")["SetText"]("password");
page["Login"]["Button"]("OK")["Click"]();
page["Wait"]();
}
Cross-platform web tests are not able to access the Login dialog directly. However, if your test is running in Chrome, Firefox, or Edge (Chromium-based), you can send the required credentials as part of the target web page URL. For example:
Where user-name and password are credentials required to access your tested web application.
Note: This workaround is not available for Internet Explorer, Safari, and other web browsers you may use in your cross-platform web tests.
Checking if a Dialog Is Displayed
In keyword tests, you can use the If Object operation to check if a specific JavaScript or a browser dialog is currently displayed. For example, the test below checks for an alert dialog and presses the dialog’s OK button on success:

In scripts, you should call the page’s WaitAlert()
, WaitConfirm()
, WaitPrompt()
or WaitLogin()
method to wait for the respective dialog. These methods delay the script execution until the given dialog appears or the specified time limit is reached. To determine whether the dialog was displayed, check the Exists
property of the returned object.
JavaScript, JScript
if (page.WaitAlert(10000).Exists) // Wait 10 sec for an alert to appear
{
page.Alert.Button("OK").ClickButton();
}
Python
if (page.WaitAlert(10000).Exists): # Wait 10 sec for an alert to appear
page.Alert.Button("OK").ClickButton();
VBScript
If page.WaitAlert(10000).Exists Then ' Wait 10 sec for an alert to appear
page.Alert.Button("OK").ClickButton
End If
DelphiScript
if page.WaitAlert(10000).Exists then // Wait 10 sec for an alert to appear
begin
page.Alert.Button('OK').ClickButton;
end;
C++Script, C#Script
if (page["WaitAlert"](10000)["Exists"]) // Wait 10 sec for an alert to appear
{
page["Alert"]["Button"]("OK")["ClickButton"]();
}
The timeout parameter is compulsory. There are also two special timeout values:
-
0 - check to see if the dialog exists only once and then resume the script execution;
-
-1 - an infinite timeout.
For more information, see Checking Whether an Object Exists and Waiting for an Object, Process or Window Activation.
Samples
TestComplete includes a sample test project that demonstrates how to automate JavaScript popups and browser dialogs:
<TestComplete Samples>\Web\Dialogs Sample
Note: | If you do not have the sample, download the TestComplete Samples installation package from the support.smartbear.com/testcomplete/downloads/samples page of our website and run it. |
For more information on this sample, refer to the Web Testing Samples help topic.
Possible Issues
Recent Firefox versions support the multiprocess mode. If this mode is active, then depending on the Firefox configuration TestComplete may fail to recognize the web dialogs. They will not be displayed in the object tree of the Object Browser, and TestComplete will not record user actions on them. To work around the problem, disable the multiprocess mode in Firefox.
See Also
How To
Accessing DOM document Object
Alert Object
Confirm Object
Prompt Object
Login Object