Testing conditions may vary from one test run to another. It is often necessary to wait until a process launches or a window becomes active. To make tests wait for tested objects to become available in the system, you can use one of the following approaches:
Using timeouts
Default auto-wait timeout
By default, TestComplete is waiting for tested objects to become available in the system for a time period specified by the Auto-wait timeout project option. By default, it is 10 seconds. If the object is not found by the end of the specified period, TestComplete reports an error to the test log.
The Auto-wait timeout affects keyword test operations that simulate user actions over UI elements, for example, the On-Screen Action operation. It also affects the following methods:
Custom timeouts in keyword tests
To wait for a needed tested object to become available in a keyword test, you can specify a custom timeout for the operation that interacts with the object. For information on how to do this, see Specifying Custom Timeouts For Operations.
Using WaitNNN Methods
You can use the WaitNNN
methods to wait for objects in tests. These methods have the Timeout
parameter that specifies the period during which TestComplete will wait for the needed object. The methods will return the needed object if it exists; otherwise, they will return a stub object. To check whether the returned object is a valid object, use the Exists
property.
-
Use the
Sys.WaitProcess
andProcess.WaitWindow
methods to wait for a process to be launched or a window to be opened respectively.Note: If your tested application is added to the Tested Applications collection of your project in TestComplete, and you start the application by using the Run Tested App operation or the
TestedApp.Run
method, you can use the Timeout parameter of the operation or method respectively to wait for the application’s process to start. -
In desktop applications, use the
WaitXXXX
methods that match the type of the tested objects:WaitWinFormsObject
,WaitCLXObject
,WaitJavaFXObject
,WaitQtObject
,WaitSlObject
,WaitWPFObject
, and so on. -
Use the
Sys.WaitBrowser
method to wait for a browser process. -
Use the
Browser.WaitPage
method to wait for a web page to load. Note: Dynamic web pages can load additional content after the page is loaded, you may need to configure your tests to wait for that content to become available. To learn more, see Wait For Web Pages. -
Use the
WaitElement
method to wait for objects on a web page. -
Use the
WaitChild
method to address the object directly by its name. This method waits for a child to appear in the parent’s child list for a specified timeout period. -
Use the
WaitNamedChild
andWaitAliasChild
methods to delay the test execution until the specified mapped child object or child alias appears in the system.
For detailed information about WaitNNN
methods, see their description.
In script tests
The following code sample shows how to wait for a desktop process:
JavaScript, JScript
p = Sys.WaitProcess("myapp", 2000);
if (p.Exists) // If the process exists ...
...
Python
p = Sys.WaitProcess("myapp", 2000)
if p.Exists: # If the process exists ...
...
VBScript
Set p = Sys.WaitProcess("myapp", 2000)
If p.Exists Then' If the process exists ...
...
DelphiScript
p := Sys.WaitProcess('myapp', 2000);
if p.Exists then// If the process exists ...
...
C++Script, C#Script
p = Sys["WaitProcess"]("myapp", 2000);
if (p["Exists"]) // If the process exists ...
...
The following code sample shows how to wait for a web browser:
JavaScript, JScript
p = Sys.WaitBrowser("chrome", 2000);
if (p.Exists) // if the browser exists ...
...
Python
p = Sys.WaitBrowser("chrome", 2000)
if p.Exists: # If the browser exists ...
...
VBScript
Set p = Sys.WaitBrowser("chrome", 2000)
If p.Exists Then' If the browser exists ...
...
DelphiScript
p := Sys.WaitBrowser('chrome', 2000);
if p.Exists then// If the browser exists ...
...
C++Script, C#Script
p = Sys["WaitBrowser"]("chrome", 2000);
if (p["Exists"]) // If the browser exists ...
...
The following code shows how to use the Process.WaitWindow
method to wait for a window of a desktop application to become available. Note: The window may be created, but not visible onscreen.
JavaScript, JScript
p = Sys.Process("Notepad");
// Waits for the window for 10 seconds
w = p.WaitWindow("*", "Open*", -1, 10000);
if (w.Exists)
{
w.Activate();
Log.Picture(w, "Open dialog picture");
}
else
Log.Warning("Incorrect window");
Python
p = Sys.Process("Notepad")
# Waits for the window for 10 seconds
w = p.WaitWindow("*", "Open*", -1, 10000)
if w.Exists:
w.Activate
Log.Picture(w, "Open dialog picture")
else:
Log.Warning("Incorrect window")
VBScript
Set p = Sys.Process("Notepad")
' Waits for the window for 10 seconds
Set w = p.WaitWindow("*", "Open*", -1, 10000)
If w.Exists Then
w.Activate
Log.Picture w, "Open dialog picture"
Else
Log.Warning "Incorrect window"
End If
DelphiScript
p := Sys.Process('Notepad');
// Waits for the window for 10 seconds
w := p.WaitWindow('*', 'Open*', -1, 10000);
if w.Exists then
begin
w.Activate;
Log.Picture(w, 'Open dialog picture');
end
else
Log.Warning('Incorrect window')
C++Script, C#Script
p = Sys["Process"]("Notepad");
// Waits for the window for 10 seconds
w = p["WaitWindow"]("*", "Open*", -1, 10000);
if (w["Exists"])
{
w["Activate"]();
Log["Picture"](w, "Open dialog picture");
}
else
Log["Warning"]("Incorrect window");
The example below shows how to use the WaitChild
method to pause the test run until the specified child object becomes available:
JavaScript, JScript
var p, s;
...
s = "Process(\"WINWORD\")";
p = Sys.WaitChild(s, 10000);
if (p.Exists)
Log.Message(p.Name);
else
Log.Message("Not found");
Python
s = 'Process("WINWORD")'
p = Sys.WaitChild(s, 10000)
if p.Exists:
Log.Message(p.Name)
else:
Log.Message("Not found")
VBScript
s = "Process(""WINWORD"")"
Set p = Sys.WaitChild(s, 10000)
If p.Exists Then
Log.Message p.Name
Else
Log.Message "Not found"
End If
DelphiScript
var s, p;
...
s := 'Process(''WINWORD'')';
p := Sys.WaitChild(s, 10000);
if p.Exists then
Log.Message(p.Name)
else
Log.Message('Not found');
C++Script, C#Script
var p, s;
...
s = "[\"Process\"](\"WINWORD\")";
p = Sys["WaitChild"](s, 10000);
if (p["Exists"])
Log["Message"](p["Name"]);
else
Log["Message"]("Not found");
In keyword tests
To call the WaitNNN
methods from keyword tests, you can use the Call Object Method operation. For example:
-
Add the Call Object Method operation to your keyword test. TestComplete will open the Operation Parameters wizard.
-
On the first page of the wizard, type
Sys
and click Next. -
The next page will display a list of available methods of the
Sys
object. To wait for a process of a desktop application, choose theWaitProcess
method from the list. To wait for a web browser, choose theWaitBrowser
method.Click Next to continue.
-
On the next page of the wizard, you can specify the name of the process or browser to wait, the number of milliseconds to wait, and, optionally, the process or browser instance, if there are several of them in the system.
-
Click Finish.
To check whether the process or browser, for which the method waits, exists in the system:
-
Create a variable that will store a reference to the object that the method will return. It can be either a project variable or a local keyword test variable.
-
Add the Set Variable Value operation to the keyword test, right after the Call Object Method operation, and configure the operation to store the last operation’s result to the created variable.
-
Add the If … Then operation to the keyword test and specify the condition to check:
-
In the Value 1 column, set the Mode to Code Expression and type the expression that will check if the object that the created variable stores exists. For example:
KeywordTests.Test1.Variables.p.Exists -
In the Condition column, select equal.
-
In the Value 2 column, set Type to Boolean and select True in the Value column.
-
-
Add operations that you want to run if the process exists as the child items of the If … Then operation.
-
If needed, add the Else operation after the If … Then operation, and then add operations that you want to run if the process does not exist, as the child items of the Else operation.
Desktop
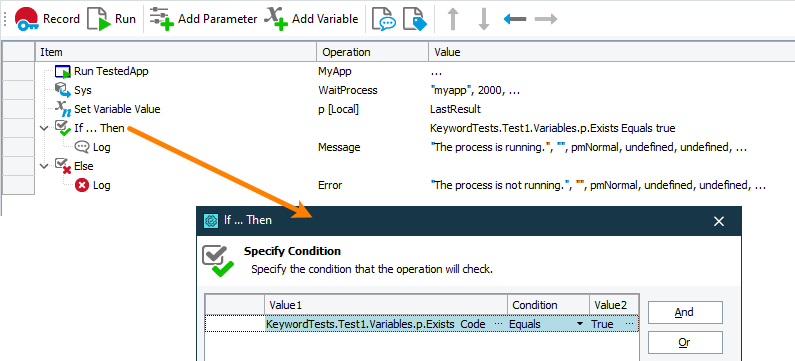
Web (Cross-Platform)
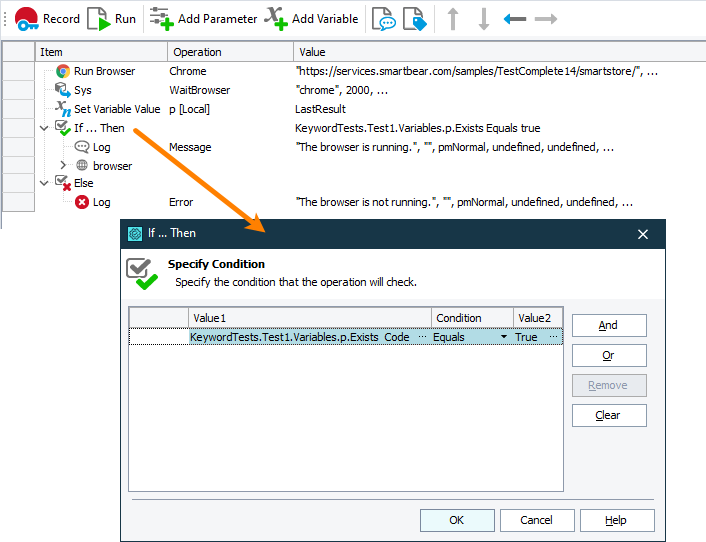
See Also
Working With Application Objects and Controls
Common Tasks
Wait For Web Pages
Waiting for Object State Changes
Waiting for an Object to Have a Specific Property Value
Delaying Test Execution