TestComplete provides support for a variety of third-party controls, including those of the Developer Express XtraBars Suite. Generally, all of the controls in TestComplete are supported in the same way: a specific program object is associated with controls of certain classes and provide access to internal properties and methods of these controls. Using these properties and methods you can interact with tested controls. For detailed information on how to specify the controls to be supported, see Object Mapping.
This topic describes the specifics of working with the BarControl, RibbonControl and PopupMenu controls of the Developer Express XtraBars Suite.
Working with the Ribbon Status Bar
Working with Items of the BarButtonItem Type
About Program Objects
BarControl represents a typical Windows-like toolbar, RibbonControl is a control that replaces traditional toolbars and tabbed pages, and PopupMenu represents a Developer Express context menu (see figures below).

BarControl
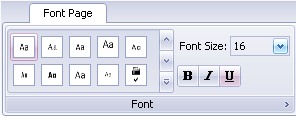
RibbonControl
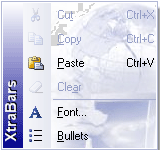
XtraPopupMenu
In spite of different functionality, the structure of these controls is similar, since all of them represent a set of specific items (buttons, labels, editors, galleries, pages, menus and so on). TestComplete lets your work with BarControl, RibbonControl and PopupMenu controls via the DevExpressBarControl
, DevExpressRibbonControl
and XtraPopupMenu
objects, respectively.
All of these objects have similar sets of specific properties and methods. Although the names of properties and methods of the XtraPopupMenu
object are different from those of DevExpressBarControl
and DevExpressRibbonControl
, however, they are similar too. That is, for instance, Check
, Click
and DblClick
perform the same as CheckItem
, ClickItem
and DblClickItem
.
In addition to a number of properties and methods provided by TestComplete, the above-mentioned program objects provide access to .NET-specific properties and methods. So, you can get deeper access to the tested control’s internals.
Subitem Types
TestComplete provides specific program objects that allow you to work with XtraBars controls and their items. Note that XtraBars controls are complex controls that consist of various items, including containers. Each container component can contain different items that in general can be of different types (including other containers). To simplify addressing certain items, TestComplete treats XtraBars controls as a tree-like structure. Using successive addressing, you can refer to any control item.
When working with items of supported XtraBars controls from tests, you can get access to their internal properties and methods via special program objects provided by TestComplete. Note that TestComplete analyzes the type of an item and associates it with one of three available object types:
-
Standard item (the
Item
object). These program objects contain only those properties and methods that are common for all XtraBars items. They are associated with those items for which specific actions are of no importance (like labels, for example). -
Clickable item (the
ClickItem
object). In addition to common properties and methods, these program objects contain a number of click actions. They are associated with clickable items, like buttons and menu items. -
Checkable item (the
CheckItem
object). In addition to properties and methods of clickable items, these program objects contains properties and methods specific to checkable items, like checkable buttons, checkable menu items and so on.
Using specific properties and methods provided by these objects, you can interact with the needed items from tests.
Addressing Items
General principles of addressing controls’ items are similar for all supported controls of the XtraBars suite. TestComplete treats underlying XtraBars controls as hierarchical lists of items, in other words, as tree-like structures. When referring to any control item, you have to obtain the application object that corresponds to the tested control. Then you can either obtain the target item directly, or obtain the parent items and the target item one-by-one. For a detailed description on how to address items in tree-like structures, see Addressing Tree View Items in Desktop Windows Applications.
You can get access to the desired item via the wItems
(or Items
) property of the corresponding program object. You can access a certain item of a tested BarControl, RibbonControl or PopupMenu control by specifying its caption or index. Here is an example:
JavaScript, JScript
function MyTest()
{
var w1;
w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("QuickAccessToolbar").Items.Item("Paint style");
// or
// w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item(1).Items.Item(4);
w1.Click();
}
Python
def MyTest():
w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("QuickAccessToolbar").Items.Item("Paint style")
# or
# w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item(1).Items.Item(4)
w1.Click()
VBScript
Sub MyTest
Dim w1
Set w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("QuickAccessToolbar").Items.Item("Paint style")
' or
' Set w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item(1).Items.Item(4)
w1.Click
End Sub
DelphiScript
procedure MyTest;
var w1 : OleVariant;
begin
w1 := Sys.Process('MyRibbonApp').WinFormsObject('frmMain').WinFormsObject('ribbonControl').wItems.Item('QuickAccessToolbar').Items.Item('Paint style');
// or
// w1 = Sys.Process('MyRibbonApp').WinFormsObject('frmMain').WinFormsObject('ribbonControl').wItems.Item(1).Items.Item(4);
w1.Click();
end;
C++Script, C#Script
function MyTest()
{
var w1;
w1 = Sys["Process"]("MyRibbonApp")["WinFormsObject"]("frmMain")["WinFormsObject"]("ribbonControl")["wItems"]["Item"]("QuickAccessToolbar")["Items"]["Item"]("Paint style");
// or
// w1 = Sys["Process"]("MyRibbonApp")["WinFormsObject"]("frmMain")["WinFormsObject"]("ribbonControl")["wItems"]["Item"](1)["Items"]["Item"](4);
w1["Click"]();
}
A tested control can have a lot of subitems. So, you may experience some difficulties when addressing certain control items. If identifying a certain item is not obvious to you, you can use one of the following workarounds to get access to the desired item:
-
Explore the tested control in the Object Browser. You can iterate through the hierarchy of its items via specific properties of the corresponding program object. For detailed information, see Exploring Applications.
-
Record a simple test over the desired item, for example, click on it. After you stop recording, you will get the needed test operations.
-
If you cannot obtain the name of the container to which the desired item belongs, you can address it by the index. This way is inconvenient, since it makes tests less readable. Addressing by caption is usually more intuitive than addressing by index (see the sample code below). The following code snippet demonstrates how you can click an item using different statements:
JavaScript, JScript
function Example()
{
var w1;
w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl");
w1.ClickItem("Home|Edit|CaptionButton");
w1.ClickItem("[1]|[1]|[4]");
}Python
def Example(): w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl") w1.ClickItem("Home|Edit|CaptionButton") w1.ClickItem("[1]|[1]|[4]")
VBScript
Sub Example
Dim w1
Set w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl")
Call w1.ClickItem("Home|Edit|CaptionButton")
Call w1.ClickItem("[1]|[1]|[4]")
End SubDelphiScript
function Example();
var w1:OleVariant;
begin
w1 := Sys.Process('MyRibbonApp').frmMain.WinFormsObject('ribbonControl');
w1.ClickItem('Home|Edit|CaptionButton');
w1.ClickItem('[1]|[1]|[4]');
end;C++Script, C#Script
function Example()
{
var w1;
w1 = Sys["Process"]("MyRibbonApp")["frmMain"]["WinFormsObject"]("ribbonControl");
w1["ClickItem"]("Home|Edit|CaptionButton");
w1["ClickItem"]("[1]|[1]|[4]");
}
Working with the Ribbon Status Bar
Ribbon status bars are a single control connected with a ribbon control. Make sure that no application has a ribbon status bar by itself. A ribbon status bar always accompanies a ribbon control. So, TestComplete combines support for ribbon controls and ribbon status bar controls in one program object (DevExpressRibbonControl
). While recording and playing back tests, TestComplete treats actions over a ribbon status bar as actions over one of the top-level ribbon items. See the example below to learn how to interact with a ribbon status bar via the DevExpressRibbonBar
program object:
JavaScript, JScript
function Test()
{
var w1;
// Obtain a ribbon control
w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl");
// Simulate clicks using specific actions of the corresponding program object
w1.ClickItem("StatusBar| New Document 1");
w1.ClickItem("StatusBar|About");
}
Python
def Test():
# Obtain a ribbon control
w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl")
# Simulate clicks using specific actions of the corresponding program object
w1.ClickItem("StatusBar| New Document 1")
w1.ClickItem("StatusBar|About")
VBScript
Sub Test
Dim w1
' Obtain a ribbon control
Set w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl")
' Simulate clicks using specific actions of the corresponding program object
Call w1.ClickItem("StatusBar| New Document 1")
Call w1.ClickItem("StatusBar|About")
End Sub
DelphiScript
procedure Test;
var w1 : OleVariant;
begin
// Obtain a ribbon control
w1 := Sys.Process('MyRibbonApp').frmMain.WinFormsObject('ribbonControl');
// Simulate clicks using specific actions of the corresponding program object
w1.ClickItem('StatusBar| New Document 1');
w1.ClickItem('StatusBar|About');
end;
C++Script, C#Script
function Test()
{
var w1;
// Obtain a ribbon control
w1 = Sys["Process"]("MyRibbonApp")["frmMain"]["WinFormsObject"]("ribbonControl");
// Simulate clicks using specific actions of the corresponding program object
w1["ClickItem"]("StatusBar| New Document 1");
w1["ClickItem"]("StatusBar|About");
}
As you can see, a ribbon status bar is recognized as a ribbon item named "StatusBar". This name is the same for all applications. If needed, you can explore your application in the Object Browser to find the index of the ribbon item that corresponds to the ribbon status bar. However, it is more convenient to address this item by its name, since it does not vary in applications.
Tip: | Note that, if needed, you can still access the tested ribbon status bar from tests by addressing the status bar’s window directly. In this case, you can test your ribbon status bar as an ordinary onscreen object (that is, by simulating clicks and keystrokes):
JavaScript, JScript Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonStatusBar").Click(5,5);
Python
VBScript Call Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonStatusBar").Click(5,5)
DelphiScript Sys.Process('MyRibbonApp').frmMain.WinFormsObject('ribbonStatusBar').Click(5,5);
C++Script, C#Script Sys["Process"]("MyRibbonApp")["frmMain"]["WinFormsObject"]("ribbonStatusBar").Click(5,5);
|
Working with Items of the BarButtonItem Type
This note applies to those items of the BarButtonItem type that have the DropDown
style (for detailed information on item types, see the Developer Express documentation). These items represent a component (a button or a menu item) that can invoke a context menu. There are two possible cases:
-
The native
ActAsDropDown
property of a BarButtonItem item is set to True. In this case, you address items of the corresponding context menu as child items of the given item (see the example below):JavaScript, JScript
function MyTest()
{
var w1, Item;
w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("TopItem").Items.Item("SubItem");
Item = w1.Items.Item("TargetItem");
Item.Click();
}Python
def MyTest(): w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("TopItem").Items.Item("SubItem") Item = w1.Items.Item("TargetItem") Item.Click()
VBScript
Sub MyTest
Dim w1, Item
Set w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("TopItem").Items.Item("SubItem")
Set Item = w1.Items.Item("TargetItem")
Call Item.Click
End SubDelphiScript
procedure MyTest;
var w1, Item : OleVariant;
begin
w1 := Sys.Process('MyRibbonApp').WinFormsObject('frmMain').WinFormsObject('ribbonControl').wItems.Item('TopItem').Items.Item('SubItem');
Item := w1.Items.Item('TargetItem');
Item.Click();
end;C++Script, C#Script
function MyTest()
{
var w1, Item;
w1 = Sys["Process"]("MyRibbonApp")["WinFormsObject"]("frmMain")["WinFormsObject"]("ribbonControl")["wItems"]["Item"]("TopItem")["Items"]["Item"]("SubItem");
Item = w1["Items"]["Item"]("TargetItem");
Item["Click"]();
} -
The native
ActAsDropDown
property of the BarButtonItem item is set to False. In this case, the given item has only one child item named "DropDownButton". Clicking this item invokes the context menu that contains the items. See the sample code below to learn how to address these items:JavaScript, JScript
function MyTest()
{
var w1, Item;
w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("TopItem").Items.Item("SubItem").Items.Item("DropDownButton");
Item = w1.Items.Item("TargetItem");
Item.Click()
}Python
def MyTest(): w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("TopItem").Items.Item("SubItem").Items.Item("DropDownButton") Item = w1.Items.Item("TargetItem") Item.Click()
VBScript
Sub MyTest
Dim w1, Item
Set w1 = Sys.Process("MyRibbonApp").WinFormsObject("frmMain").WinFormsObject("ribbonControl").wItems.Item("TopItem").Items.Item("SubItem").Items.Item("DropDownButton")
Set Item = w1.Items.Item("TargetItem")
Call Item.Click
End SubDelphiScript
procedure MyTest;
var w1, Item : OleVariant;
begin
w1 := Sys.Process('MyRibbonApp').WinFormsObject('frmMain').WinFormsObject('ribbonControl').wItems.Item('TopItem').Items.Item('SubItem').Items.Item('DropDownButton');
Item := w1.Items.Item('TargetItem');
Item.Click();
end;C++Script, C#Script
function MyTest()
{
var w1, Item;
w1 = Sys["Process"]("MyRibbonApp")["WinFormsObject"]("frmMain")["WinFormsObject"]("ribbonControl")["wItems"]["Item"]("TopItem")["Items"]["Item"]("SubItem")["Items"]["Item"]("DropDownButton");
Item = w1["Items"]["Item"]("TargetItem");
Item["Click"]();
}
Note that the item’s native DropDownControl
property must be associated with the corresponding context menu.
Working with the Ribbon’s Options Menu
You may need to work with certain subitems of a context menu that specifies ribbon control options. This menu is invoked by right-clicking over any ribbon item. When working with this menu from tests, address it as the CustomizationPopupMenu item of the ribbon control. This name is the same for all applications. Here is the sample code:
JavaScript, JScript
function MyTestRoutine()
{
var w1;
// Specify the top-level ribbon object
w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl");
// Invoke the context menu and simulate a single click over its item
w1.ClickItemR("First Page");
w1.ClickItem("CustomizationPopupMenu|Show Quick Access Toolbar Below the Ribbon");
}
Python
def MyTestRoutine():
# Specify the top-level ribbon object
w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl")
# Invoke the context menu and simulate a single click over its item
w1.ClickItemR("First Page")
w1.ClickItem("CustomizationPopupMenu|Show Quick Access Toolbar Below the Ribbon")
VBScript
Sub MyTestRoutine
Dim w1
' Specify the top-level ribbon object
Set w1 = Sys.Process("MyRibbonApp").frmMain.WinFormsObject("ribbonControl")
' Invoke the context menu and simulate a single click over its item
Call w1.ClickItemR("First Page")
Call w1.ClickItem("CustomizationPopupMenu|Show Quick Access Toolbar Below the Ribbon")
End Sub
DelphiScript
procedure MyTestRoutine;
var w1 : OleVariant;
begin
// Specify the top-level ribbon object
w1 := Sys.Process('RibbonApp').frmMain.WinFormsObject('ribbonControl');
// Invoke the context menu and simulate a single click over its item
w1.ClickItemR('First Page');
w1.ClickItem('CustomizationPopupMenu|Show Quick Access Toolbar Below the Ribbon');
end;
C++Script, C#Script
function MyTestRoutine()
{
var w1;
// Specify the top-level ribbon object
w1 = Sys["Process"]("MyRibbonApp")["frmMain"]["WinFormsObject"]("ribbonControl");
// Invoke the context menu and simulate a single click over its item
w1["ClickItemR"]("First Page");
w1["ClickItem"]("CustomizationPopupMenu|Show Quick Access Toolbar Below the Ribbon");
}
Note that you cannot perform actions over CustomizationPopupMenu, that is, if you write w1.ClickItem("CustomizationPopupMenu")
in the sample code above, it will fail. You can only work with CustomizationPopupMenu subitems.
Note on Working with Context Menus in XtraForms
If you are testing a .NET application that uses PopupMenu controls in forms derived from the Developer Express XtraForm class, you may encounter the “Cannot obtain the popup menu” error when accessing the context menu via the XtraPopupMenu
property during the test run. The problem is caused by the specifics of XtraForm forms. It may occur if your test invokes the popup menu and selects a command from it several times in succession. If you encounter this problem, we recommend that the tested application’s developers contact Developer Express support for assistance in resolving the problem.
See Also
Exploring Applications
Developer Express BarControl Support
Developer Express RibbonControl Support
Developer Express Popup Menu Support
Addressing Tree View Items in Desktop Windows Applications
Object-Specific Tasks