While testing tree view controls, you can use specific properties and methods of the corresponding program object to perform certain actions and obtain data stored in controls. You can call these methods and properties from your keyword tests, as well as from scripts. This topic describes how to work with the needed properties and methods from your scripts. However, when testing a control from your keyword test, you can use the same methods and properties calling them from keyword test operations. For more information, see Keyword Tests Basic Operations.
A tree view item can be identified by its path in the tree or by a Win32TreeViewItem
object that provides a scripting interface to the item and its child items. This topic explains how you can address tree view items you are working with:
Using Item Captions and Indexes
There are several ways you can address a tree view item. Depending on the item’s location in the tree view, you can address it by its caption, index or path.
If the target item is a direct child of the current item, you can address it using its caption or index (to determine which item is focused, use the Win32TreeView.wSelection
property):
treeObj.ClickItem("Item 2.3"); // Clicks the selected item’s child item "Item 2.3"
treeObj.ClickItem(2); // Clicks the selected item’s 3rd child item
treeItemObj.Items.Item("Item 3.1").Click(); // Clicks the treeItemObj’s child item "Item 3.1"
treeItemObj.Items.Item(4).Click(); // Clicks the treeItemObj’s 5th child item
Indexes are zero-based: the first subitem has an index of 0, the second - 1, and so on. The total number of the current item’s child items is specified by the Win32TreeView.wItemCount
property, so the last subitem’s index is wItemCount
-1.
If the target item is either an indirect child of the current item (for example, a grandchild or great-grandchild), or belongs to another tree branch, you should specify the “path” to this item. The path should be relative to the current item or to the tree root, respectively. To indicate that the path starts from the tree root, place the pipeline character ( | ) at the beginning of the path. Please note the difference in the statements below:
treeObj.ClickItem("|Item 4"); // Clicks the root item "Item 4"
treeObj.ClickItem("Item 4"); // Clicks the selected item's child item "Item 4"
Note: | In the Win32TreeView.wItems.Item property, however, you should not place the pipeline character at the beginning of the item path, although this path is relative to the tree root:
treeObj.wItems.Item("TopItem 2|Item 2.3").Click(); // Correct treeObj.wItems.Item("|TopItem 2|Item 2.3").Click(); // Incorrect!!! |
Each item in the path can be specified either by its caption, or index (from 0). To separate individual items in the path, use the pipeline character ( | ). Note that any other delimiter characters except the pipeline character (for example, additional spaces) are not allowed:
treeObj.ClickItem("|TopItem 2|Item 2.3"); // Correct
treeObj.ClickItem("| TopItem 2 | Item 2.3"); // Incorrect!!!
If the item caption contains a special character (|, [ or ]), you should address it using its index or question mark wildcards:
treeObj.ClickItem("|?Item1?") // Clicks the root item "[Item1]"
If the item caption contains the asterisk character (*), you should double it:
treeObj.ClickItem("|Item **") // Clicks the root item "Item*"
To include the item’s index in the path, enclose it in brackets - [ and ]. At that, the brackets should contain only the index number; other symbols (such as spaces) are not allowed:
treeObj.ClickItem("|[1]|[2]"); // Correct
treeObj.ClickItem("|[ 1 ]|[ 2 ]"); // Incorrect!!!
As already mentioned, indexes are zero-based. For example, indexes of root items vary from 0 to Win32TreeView.wRootItemCount
-1.
When using the Win32TreeView.wItems.Item
or Win32TreeViewItem.Items.Item
properties, you can either obtain the target item directly, or obtain the parent items and the target item one by one. In the former case, you should address the item’s by its path; in the latter case you address each subsequent item by its caption or index:
treeObj.wItems.Item("TopItem 2|Item 2.3").Click();
treeObj.wItems.Item("TopItem 2").Items.Item("Item 2.3").Click();
You can combine both indexes and captions in the item path. Let’s summarize the above and illustrate this by an example. Suppose you are working with a tree view that has the structure as shown on the image below:
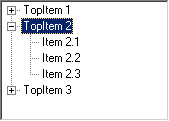
To click the Item 2.3 item, you can use any of the following statements:
treeObj.ClickItem("|TopItem 2|Item 2.3")
treeObj.ClickItem("|TopItem 2|[2]")
treeObj.ClickItem("|[1]|Item 2.3")
treeObj.ClickItem("|[1]|[2]")
treeObj.wItems.Item("TopItem 2|Item 2.3").Click()
treeObj.wItems.Item("TopItem 2|[2]").Click()
treeObj.wItems.Item("[1]|Item 2.3").Click()
treeObj.wItems.Item("[1]|[2]").Click()
treeObj.wItems.Item("TopItem 2").Items.Item("Item 2.3").Click()
treeObj.wItems.Item("TopItem 2").Items.Item(2).Click()
treeObj.wItems.Item(1).Items.Item("Item 2.3").Click()
treeObj.wItems.Item(1).Items.Item(2).Click()
In addition, taking into account that the currently selected item, TopItem 2 is the parent of the Item 2.3, you can also use the following statements:
treeObj.ClickItem("Item 2.3")
treeObj.ClickItem(2)
Usually, it is preferable to specify tree view items by captions, because this makes the test easy to understand. However, indexes can make the script shorter. In addition, indexes are useful for addressing items in owner-drawn tree view controls, since these items may not have a caption (see Working With Owner-Drawn Tree View Controls in Desktop Windows Applications).
Using Case-Sensitive and Case-Insensitive Item Caption
Depending on the Use case-sensitive parameters project option, TestComplete treats string parameters as case-sensitive or case-insensitive. By default, this option is turned off, so that you can specify the tree view item captions in action parameters in any case (all upper case, all lower case, mixed case, and so on):
treeObj.ClickItem("|RootItem|SubItem")
treeObj.ExpandItem("|rootitem|subitem")
treeObj.wItems.Item("ROOTITEM|SUBITEM").Click()
treeObj.wItems.Item("RooTiTeM|SuBiTeM").Select()
If the Use case-sensitive parameters is checked, you can specify tree view items only by their actual captions in the correct case:
// Correct:
treeObj.ClickItem("|RootItem|SubItem")
treeObj.wItems.Item("RootItem|SubItem").Select()
// Incorrect:
treeObj.ExpandItem("|rootitem|subitem")
treeObj.wItems.Item("rootitem|subitem").Click()
Using Wildcards in Item Captions
If tree view item captions change according to the current context, it may be useful to specify items only by constant parts of their captions. To specify arbitrary parts in item captions, TestComplete lets you use wildcard characters - * and ?. The asterisk (*) corresponds to a string of any length (including an empty string), the question mark corresponds to any single character (including none).
To illustrate the wildcards usage, suppose that you want to expand the disk C node in Windows Explorer’s Folders tree. In different operating systems the path to this item can be different. For example, it can be “|Desktop|My Computer|System (C:)”, or “|Desktop|Computer|System (C:)”. In addition, the disk C label can be different on different computers – it can be, for instance, System, Windows, or Local Disk (in case the disk has no name).
All things considered, to create a script that will work on different computers you should replace variable parts of the item path with wildcards:
treeObj.ClickItem("|Desktop|*Computer|*(C:)")
In this path, “*Computer” matches both “My Computer” and “Computer”, and the asterisk in the “*(C:)” string matches any name the disk C can have.
See Also
Working With Tree View Controls in Desktop Windows Applications