Wildcards are special symbols that can be used to match characters in string values. TestComplete supports two standard wildcards: the asterisk (*) and the question mark (?). The asterisk wildcard corresponds to a string of any length (including an empty string). The question mark corresponds to any single character.
TestComplete allows you to use wildcards when specifying:
-
Variable parts in window captions and class names.
-
The URLs of dynamic web pages (see Ignoring Query String Parameters).
-
The values of a mapped object’s identification properties.
The following sections of this topic provide detailed information and samples on how to use wildcards in TestComplete:
Using Wildcards in Object Identification Functions
You can use wildcards to specify variable parts in window captions and class names in calls to Window
, WaitWindow
, WaitChild
and some other methods. A window's class name and caption are parameters used to recognize a particular window. Class names and captions of some windows may change from one application run to another, so using wildcards makes the created tests stable.
The following code demonstrates how you can use wildcard characters in the WndCaption parameter of the Window
method in place of variable parts of a window caption:
JavaScript, JScript
var wndNotepad = Sys.Process("notepad").Window("Notepad", "* - Notepad");
Python
wndNotepad = Sys.Process("notepad").Window("Notepad", "* - Notepad")
VBScript
Set wndNotepad = Sys.Process("notepad").Window("Notepad", "* - Notepad")
DelphiScript
wndNotepad := Sys.Process('notepad').Window('Notepad', '* - Notepad');
C++Script, C#Script
var wndNotepad = Sys["Process"]("notepad")["Window"]("Notepad", "* - Notepad");
The code will return an object containing "- Notepad" in the value of its WndCaption
property.
Using Wildcards in Object Searching Functions
You can also use wildcards in a string passed to the Find
, FindChild
and FindID
methods. The following example demonstrates how you can use wildcards to specify a search mask for the FindChild
method. The example searches for a web page’s link containing the string test and clicks on it. Note that the sought-for text is specified along with asterisks.
JavaScript, JScript
function Test()
{
var url = "http://smartbear.com/";
// Launch Internet Explorer if it is not started yet and open a web page
if (! Sys.WaitBrowser("iexplore", 10000).Exists)
{
Browsers.Item(btIexplorer).Run(url);
var page = Sys.Browser("iexplore").Page(url);
}
else
var page = Sys.Browser("iexplore").ToUrl(url);
// Specify the sought-for properties and values
var props = Array("tagName", "contentText");
var values = Array("a", "*test*");
// Find an object that corresponds to the specified criteria
var link = page.FindChild(props, values, 10);
if (link.Exists)
link.Click();
}
Python
def Test():
url = "https://smartbear.com/"
# Launch Internet Explorer if it is not started yet and open a web page
if not Sys.WaitBrowser("iexplore", 10000).Exists:
Browsers.Item[btIExplorer].Run(url)
page = Sys.Browser("iexplore").Page(url)
else:
page = Sys.Browser("iexplore").ToUrl(url)
# Specify the sought-for properties and values
props = ["tagName", "contentText"]
values = ["a", "*test*"]
# Find an object that corresponds to the specified criteria
link = page.FindChild(props, values, 10)
if (link.Exists):
link.Click()
VBScript
Sub Test
Dim url, page, props, values, link
url = "http://smartbear.com/"
' Launch Internet Explorer if it is not started yet and open a web page
If Not Sys.WaitBrowser("iexplore", 10000).Exists Then
Browsers.Item(btIexplorer).Run url
Set page = Sys.Browser("iexplore").Page(url)
Else
Set page = Sys.Browser("iexplore").ToUrl(url)
End If
' Specify the sought-for properties and values
props = Array("tagName", "contentText")
values = Array("a", "*test*")
' Find an object that corresponds to the specified criteria
Set link = page.FindChild(props, values, 10)
If link.Exists Then
link.Click
End If
End Sub
DelphiScript
procedure Test();
var url, page, props, values, link;
begin
url := 'http://smartbear.com/';
// Launch Internet Explorer if it is not started yet and open a web page
if not Sys.WaitBrowser('iexplore', 10000).Exists then
begin
Browsers.Item(btIexplorer).Run(url);
page := Sys.Browser('iexplore').Page(url);
end
else
page := Sys.Browser('iexplore').ToUrl(url);
// Specify the sought-for properties and values
props := ['tagName', 'contentText'];
values := ['a', '*test*'];
// Find an object that corresponds to the specified criteria
link := page.FindChild(props, values, 10);
if link.Exists then
link.Click;
end;
C++Script, C#Script
function Test()
{
var url = "http://smartbear.com/";
// Launch Internet Explorer if it is not started yet and open the sample web page
if (! Sys["WaitBrowser"]("iexplore", 10000).Exists)
{
Browsers["Item"](btIexplorer)["Run"](url);
var page = Sys["Browser"]("iexplore")["Page"](url);
}
else
var page = Sys["Browser"]("iexplore")["ToUrl"](url);
// Specify the sought-for properties and values
var props = Array("tagName", "contentText");
var values = Array("a", "*test*");
// Find an object that corresponds to the specified criteria
var link = page["FindChild"](props, values, 10);
if (link["Exists"])
link["Click"]();
}
Using Wildcards in Name Mapping
You can also use wildcards to make your Name Mapping repository more flexible. Depending on your application’s specifics, the recognition parameters of the tested objects may change from one application run to another, while TestComplete cannot predict such changes. So, TestComplete may use mapping criteria that are not reliable. However, you can make recognition criteria independent of changes in object properties by using wildcards when specifying values of identification properties. For example, you can use wildcards to mask those parts of property values that can occasionally change, like window class names or captions.
Suppose you want to map a control so that its WndCaption
property contains any non-empty value. In this case, you can use the ?* wildcard in Name Mapping. The value means that the property value must contain at least one symbol.
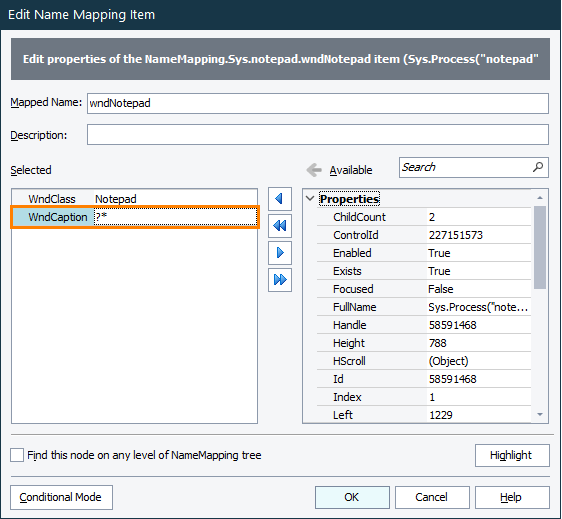
Another example of using wildcards is specifying web page URLs. The fact is that URLs of dynamic web pages can contain a query string (that is, a list of parameters passed after the question mark in the URL). The values of query string parameters depend on the current context. Some of them (for instance, the IDs of requested web pages) can be static, while others (such as session IDs) change from time to time. To make mapping settings independent of dynamic changes in web page URLs, you can use the asterisk (*) wildcard in place of the values -- http://www.example.com/index.asp?act=*&sid=*
, or in place of the entire query string -- http://www.example.com/index.asp*
.
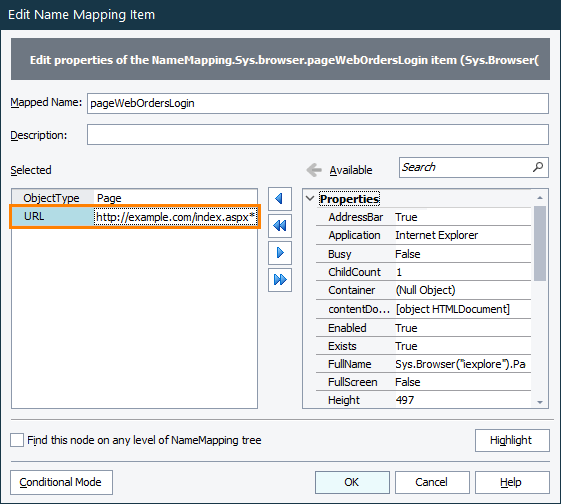
To match any URL, specify a single asterisk (*) as the identification property value.