This tutorial explains how to convert a Selenium 2 script into a runnable JAR file. The resulting JAR file can be uploaded to the AlertSite monitoring platform to be run from many monitoring locations worldwide.
We will first export the script to a Java file using the JUnit test format, then create and configure a Java project using Eclipse IDE, and finally build the project into as a runnable JAR file.
If you already have a ready Eclipse JUnit project, you can skip to step 3 – Add Main Method.
What You Will Need
-
Selenium client libraries v 2.53 for Java: https://selenium-release.storage.googleapis.com/2.53/selenium-java-2.53.1.zip
-
Firefox version 123.0.1 (64-bit): https://ftp.mozilla.org/pub/firefox/releases/123.0.1/
This version works best with Selenium 2.53 and does not require any additional drivers. If you already have a later version of Firefox, you can install Firefox 123 side-by-side with your existing Firefox version. Make sure to disable auto-update in Firefox 123 options (Options > Advanced > Update) so that it does not update to an unsupported version.
-
Selenium IDE (an add-on for Firefox): https://addons.mozilla.org/en-US/firefox/addon/selenium-ide/
-
Java Development Kit (JDK) 8: http://www.oracle.com/technetwork/java/javase/downloads/jdk8-downloads-2133151.html
-
Eclipse IDE for Java Developers: http://www.eclipse.org/downloads/
1. Export Selenium Script
We assume that you already have a script recorded with Selenium IDE. Refer to the Selenium IDE documentation to learn how to create Selenium scripts.
![]() |
Make sure your script is played back successfully in the Selenium IDE before you export it. |
To export your Selenium script to a Java file:
-
In Selenium IDE, select File > Export Test Case As > Java / JUnit / Web Driver from the menu. (AlertSite supports only JUnit-based Selenium scripts.)
Or, if you have a test suite with several test cases and you want to export the entire suite, select File > Export Test Suite As > Java / JUnit / WebDriver.
-
Save the generated .java file.
2. Create Eclipse Project
To create a new Java project in Eclipse, select File > New > Java Project from the Eclipse menu.
Specify the project name and location. Leave other options as is and click Next.
On the next page, switch to the Libraries tab and click Add External JARs.
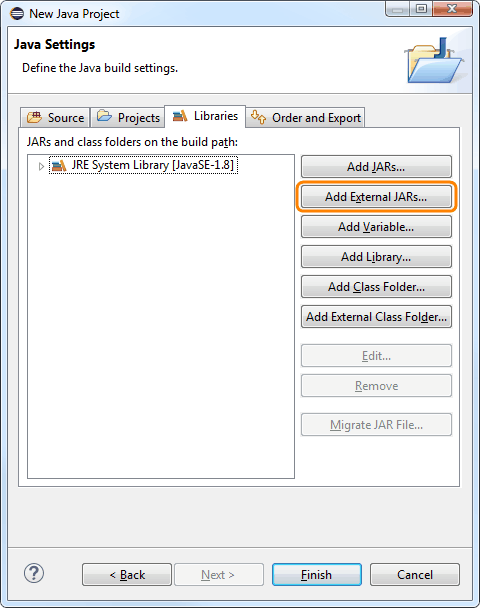
Browse to the folder where you unzipped the Selenium Java libraries and add the selenium-java-X.X.X.jar file (not selenium-java-X.X.X-srcs.jar).
Click Add External JARs again and add all files from the Selenium libs subfolder. To select all files, press Ctrl+A on Windows or Command+A on Mac.
Click Finish. Your project should look like this:
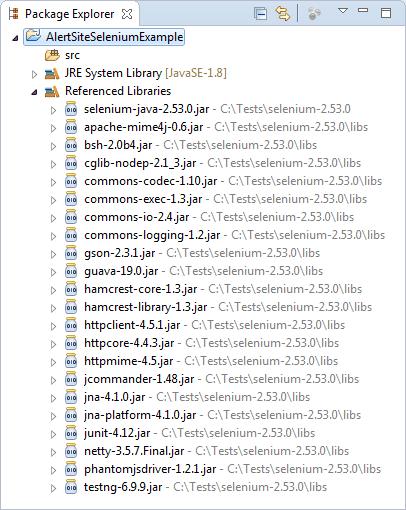
Next, create a package (namespace) for your Selenium test. To do that, right-click the project and select New > Package.
Enter the package name and click Finish.
Tip: | Java package names should be all lowercase and begin with your company’s reversed Internet domain name, for example, com.smartbear.tests or org.mycompany.productname.tests. |
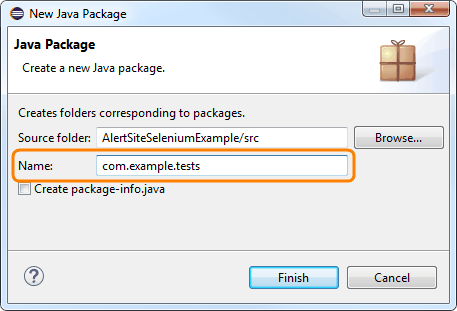
Copy or move your Selenium .java file to the folder of the created package (in the above example – to the src\com\example\tests subfolder of the Eclipse project).
After refreshing (F5) your project should look like this.
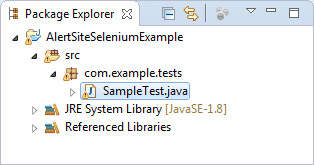
3. Add Main Method
A runnable JAR needs a main
method. Java code generated by Selenium does not include the main
method, but it is easy to add it.
Edit your .java file and add these lines at the beginning of the file, after other imports:
Java
import org.junit.After;
import org.junit.Before;
import org.junit.Test;
import org.junit.internal.TextListener;
import org.junit.runner.JUnitCore;
import org.junit.runner.Result;
Then, add this main
method inside the class:
Java
public static void main(String args[]) {
JUnitCore junit = new JUnitCore();
junit.addListener(new TextListener(System.out));
Result result = junit.run(SampleTest.class); // Replace "SampleTest" with the name of your class
if (result.getFailureCount() > 0) {
System.out.println("Test failed.");
System.exit(1);
} else {
System.out.println("Test finished successfully.");
System.exit(0);
}
}
If you have several Firefox versions installed, add the following lines at the beginning of the main
method to specify the path to Firefox 123:
Java
if (System.getProperty("webdriver.firefox.bin") == null) {
// Replace with your Firefox 46 path
System.setProperty("webdriver.firefox.bin", "C:\\Program Files (x86)\\Mozilla Firefox 123\\firefox.exe");
}
Your code should look like this:
(Optional.) If Eclipse shows warnings about unused imports and methods, remove unused code.
Now, let us run the code to make sure it works correctly. To do that, right-click the .java file and select Run As > Java Application.
This will run your Selenium script: start Firefox, navigate to the tested web page, perform the test actions, and then close the browser. You should see Test finished successfully in the Eclipse console.
If an error occurs, fix the code to avoid the error. Solutions for various Selenium and Java errors can be found on the Internet.
4. Export Project as Runnable JAR
Once your code plays back successfully, you need to export the Java project to a runnable JAR file so that it can be run by AlertSite.
To export your project, right-click it and select Export.
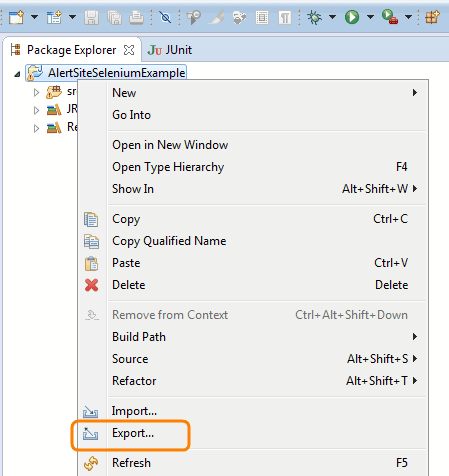
Select Java > Runnable JAR file as the export destination and click Next.
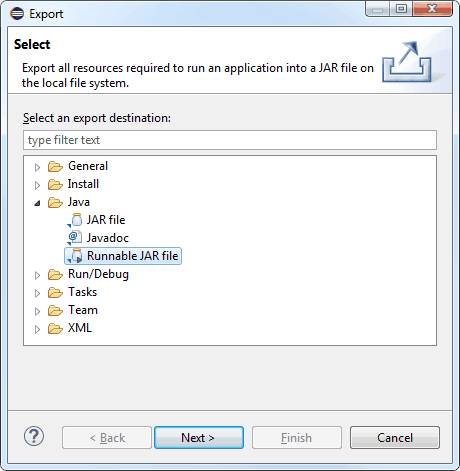
On the next page, specify the name and path of the JAR file to create and select the Launch configuration that includes the project name and the name of the test class.
Also, be sure to select Package required libraries into generated JAR to embed Selenium libraries into your JAR file. JARs created without this option cannot be run by AlertSite.
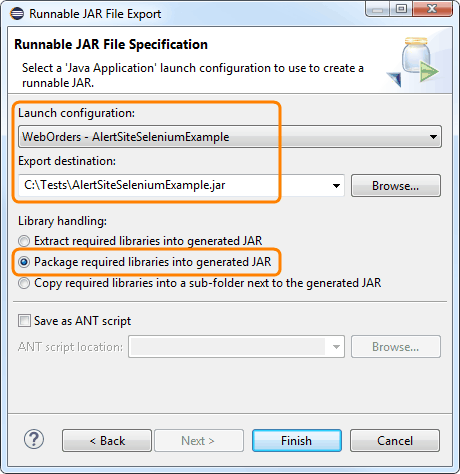
Click Finish to create the JAR file.
To confirm that the JAR file has been properly packaged, open the command prompt and run this command:
java -jar <path>\filename.jar
For example:
java -jar C:\Tests\AlertSiteSeleniumExample.jar
This will open Firefox and run your Selenium script. You should see Test finished successfully in the console.
What is Next
Now that you have a runnable JAR file containing your Selenium script, you can upload it to AlertSite to run your script from various locations around the world.
See Also
Website Monitoring With Selenium and AlertSite
Guidelines for Selenium Scripts