Why Typecasting Is Needed
Each test object (a process, window, web page or control) implements multiple interfaces, as you can see this in the TestLeft UI Spy. Each of them provides various methods (and properties) for automation.
When you refer to an object in test code, you specify the target interface through which you want to work with the object. While you do not always need to declare a variable, your object references always need to be of a specific interface.
The interface you use determines which methods (and properties) you can apply to an object.
For example, in a .NET test code, if you save a text box object to an ITextEdit
-type variable, you can use the methods and properties provided by the ITextEdit
interface or by the interfaces it inherits from. In a Java test code, if you save a text box object to a TextEdit
-type variable, you can use the TextEdit
methods and methods of its ancestors:
C#
ITextEdit textbox = browser.Find<ITextEdit>(new WebElementPattern()
{
ObjectType = "Textbox"
idStr = "searchBox"
}, 4);
Visual Basic .NET
Dim textbox As ITextEdit = browser.Find(Of ITextEdit)(New WebElementPattern() With {
ObjectType = "Textbox"
idStr = "searchBox"
}, 4)
Java
TextEdit textbox = (TextEdit) browser.find(TextEdit.class, new WebElementPattern() {{
ObjectType = "Textbox";
idStr = "searchBox";
}}, 4);
You may need to cast an object to another interface to call methods and properties provided by this interface.
When to Typecast
Usually, you need to typecast if —
-
A test object implements two or more interfaces, and one of them does not inherit the other. A typical example is a test object that corresponds to a web check box. This object implements the interface that provides support for web elements and the interface that provide support for check boxes and those interfaces do not inherit each other.
-
You get an object as an “ancestor” interface and want to cast it to a “descendant” type. For example, a test object that corresponds to a web table’s cell. In many cases, its type is returned as
Control
. This interface is an ancestor of theWebElement
andWebCell
interfaces. You may want to typecast the test object to these interfaces to use their specific properties and methods.
If you get an object as a “descendant” interface, you do not need to typecast it to call the “ancestor” methods and properties - they are available without typecasting.
How to Typecast
To typecast, use these methods –
.NET: the Cast<T>
method of the IObject
interface that all test objects implement.
Java: the cast
method of the TestObject
class that all test objects implement.
Note: | Typecasting with as does not work for TestLeft test objects. |
C#
using SmartBear.TestLeft.TestObjects;
using SmartBear.TestLeft.TestObjects.Web;
IWebBrowser browser = Driver.Applications.RunBrowser(BrowserType.Chrome, "https://smartbear.com");
// Get the panel object
IControl panel = browser.Find<IControl>(new WebElementPattern()
{
ObjectType = "Panel",
ObjectIdentifier = "main"
}, 4);
// Typecast the object
IWebElement elem = panel.Cast<IWebElement>();
...
Visual Basic .NET
Imports SmartBear.TestLeft.TestObjects
Imports SmartBear.TestLeft.TestObjects.Web
Dim browser As IWebBrowser = Driver.Applications.RunBrowser(BrowserType.Chrome, "https://smartbear.com")
' Get the panel object
Dim panel As IControl = browser.Find(Of IControl)(New WebElementPattern() With {
.ObjectType = "Panel",
.ObjectIdentifier = "main"
}, 4)
' Typecast the object
Dim elem As IWebElement = panel.Cast(Of IWebElement)
...
Java
import com.smartbear.testleft.BrowserType;
import com.smartbear.testleft.testobjects.*;
import com.smartbear.testleft.testobjects.web*;
WebBrowser browser = driver.getApplications().runBrowser(BrowserType.Chrome, "https://smartbear.com");
// Get the panel object
Control panel = browser.find(Control.class, new WebElementPattern() {{
ObjectType = "Panel";
ObjectIdentifier = "main";
}}, 4);
// Typecast the object
WebElement elem = panel.cast(WebElement.class);
...
Determining Interfaces Supported by an Object
To determine to which interfaces you can typecast your test object, explore this object in the TestLeft UI Spy. The TestLeft UI Spy lists the interfaces to which the object can be cast.
Typecast only to these interfaces. Casting to some other interface will cause an error when you attempt to access methods or properties of this interface.
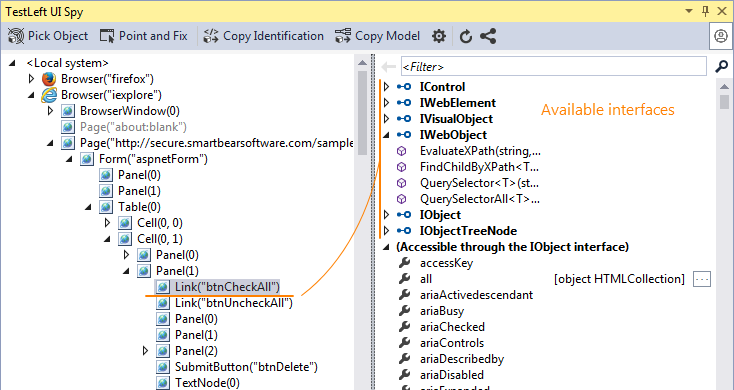
Note: | The methods and properties displayed under the Accessible through the IObject interface category (for example, “native” methods and properties) are called in a specific way, you cannot call them by using a typical object.method notation.
|
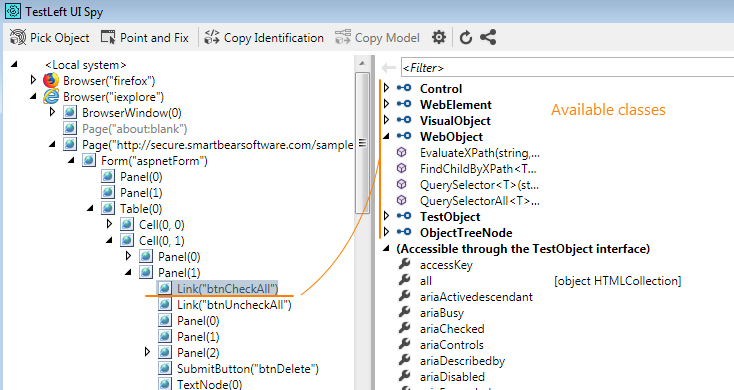
Note: | The methods and properties displayed under the Accessible through the TestObject interface category (for example, “native” methods and properties) are called in a specific way, you cannot call them by using a typical object.method notation.
|
Detecting Typecast Errors
As was said above, casting a test object to an unsupported interface will cause an error when you attempt to call methods or properties of this interface. To detect typecast errors earlier, you can do the following –
.NET: Set the IDriver.Options.Debug.RuntimeChecks
property to TypeCast or to All.
Java: Call the driver.getOptions().getDebug().setRuntimeChecks()
method with the RuntimeChecks.TypeCast
or RuntimeChecks.All
parameter.
This will make TestLeft raise an exception on attempts to cast a test object to an unsupported interface or class.
C#
using SmartBear.TestLeft.Options;
…
public void Test()
{
// Perform additional checks during code execution
Driver.Options.Debug.RuntimeChecks = Options.RuntimeChecks.TypeCast;
// Obtain and typecast objects
…
}
Visual Basic .NET
Imports SmartBear.TestLeft.Options
…
Public Sub Test()
' Perform additional checks during code execution
Driver.Options.Debug.RuntimeChecks = Options.RuntimeChecks.TypeCast
' Obtain and typecast objects
…
End Sub
Java
import com.smartbear.testleft.testobjects.*;
import com.smartbear.testleft.options.RuntimeChecks;
…
@Test
public void Test()
// Perform additional checks during code execution
driver.getOptions().getDebug().setRuntimeChecks(RuntimeChecks.TypeCast);
// Obtain and typecast objects
…
}
See Also
Creating TestLeft Tests
Methods and Properties Common for All Test Objects
Calling "Native" Properties and Methods