Description
A BrowserWindow
test object represents an opened browser window. Browser
has at least one BrowserWindow
object; additional windows can be opened using the browser’s New Window or Open Link In New Window command.
Note that BrowserWindow
is not the same as Page
. A Page
object represents a web page in a browser tab whereas a BrowserWindow
object corresponds to the browser window with menus, toolbars and so on.
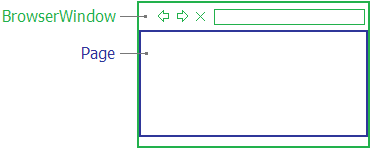
The BrowserWindow
object provides methods and properties for automating various browser window operations, such as:
-
the
Maximize
,Minimize
,Position
andActivate
methods for manipulating the window on the screen, -
the
Close
method for closing the window, -
the
WndCaption
property for getting the window title, -
and others.
![]() |
Operations recorded directly against the BrowserWindow are cross-browser. However, browser menus, toolbars and tab GUI objects are different in different browsers, so operations against them are not cross browser. If you need to include such operations in your test, you should run them conditionally depending on the current browser (see Checking the Current Browser). |
Requirements
-
A license for TestComplete Web module.
-
The Web Testing plugin. This plugin is installed and enabled automatically. The plugin implements the
BrowserWindow
test object, as well as the web testing functionality.
Members
Example
The following example runs a browser and maximizes its window on the screen:
JavaScript, JScript
function Maximizing()
{
Browsers.Item("iexplore").Run("http://smartbear.com");
Sys.Browser().BrowserWindow(0).Maximize();
}
Python
def Maximizing():
Browsers.Item["iexplore"].Run("http://smartbear.com")
Sys.Browser().BrowserWindow(0).Maximize()
VBScript
Sub Maximizing
Browsers.Item("iexplore").Run("http://smartbear.com")
Sys.Browser().BrowserWindow(0).Maximize
End Sub
DelphiScript
procedure Maximizing;
begin
Browsers.Item('iexplore').Run('http://smartbear.com');
Sys.Browser().BrowserWindow(0).Maximize();
end;
C++Script, C#Script
function Maximizing()
{
Browsers["Item"]("iexplore")["Run"]("http://smartbear.com");
Sys["Browser"]()["BrowserWindow"](0)["Maximize"]();
}
The following example calculates the number of windows opened in a browser:
JavaScript, JScript
function Test()
{
var browser = "iexplore";
Browsers.Item(browser).Run("http://smartbear.com");
Sys.Browser().BrowserWindow(0).Keys("^n"); // Open a new browser window using the Ctrl+N shortcut
Browsers.Item(browser).Navigate("http://www.google.com");
var windows = Sys.Browser().FindAllChildren("ObjectType", "BrowserWindow").toArray();
Log.Message("Number of opened browser windows: " + windows.length);
}
Python
def Test():
browser = "iexplore"
Browsers.Item[browser].Run("http://smartbear.com")
Sys.Browser().BrowserWindow(0).Keys("^n"); # Open a new browser window using the Ctrl+N shortcut
Browsers.Item[browser].Navigate("http://www.google.com")
windows = Sys.Browser(browser).FindAllChildren("ObjectType", "BrowserWindow")
Log.Message("Number of opened browser windows: " + str(len(windows)))
VBScript
Sub Test
Dim browser, windows
browser = "chrome"
Call Browsers.Item(browser).Run("http://smartbear.com")
Call Sys.Browser().BrowserWindow(0).Keys("^n") ' Open a new browser window using the Ctrl+N shortcut
Call Browsers.Item(browser).Navigate("http://www.google.com")
windows = Sys.Browser().FindAllChildren("ObjectType", "BrowserWindow")
Call Log.Message("Number of opened browser windows: " & (UBound(windows) + 1))
End Sub
DelphiScript
procedure Test;
var browser, windows;
begin
browser := 'chrome';
Browsers.Item(browser).Run('http://smartbear.com');
Sys.Browser().BrowserWindow(0).Keys('^n'); // Open a new browser window using the Ctrl+N shortcut
Browsers.Item(browser).Navigate('http://www.google.com');
windows := Sys.Browser().FindAllChildren('ObjectType', 'BrowserWindow');
Log.Message('Number of opened browser windows: ' + VarToStr(VarArrayHighBound(windows, 1)));
end;
C++Script, C#Script
function Test()
{
var browser = "iexplore";
Browsers["Item"](browser)["Run"]("http://smartbear.com");
Sys["Browser"]()["BrowserWindow"](0)["Keys"]("^n"); // Open a new browser window using the Ctrl+N shortcut
Browsers["Item"](browser)["Navigate"]("http://www.google.com");
var windows = Sys["Browser"]()["FindAllChildren"]("ObjectType", "BrowserWindow")["toArray"]();
Log["Message"]("Number of opened browser windows: " + windows["length"]);
}
See Also
Browser Object
Page Object
Classic Web Testing
Web Object Types Used by Web and Hybrid Mobile Applications