This topic explains how you can navigate to web pages in web browsers.
Note: | If the tested web application is hosted on a local web server (localhost), use the computer name instead of localhost when specifying web page URLs. This will make your test computer-independent, and you will be able to run it from other computers as well. For more information, see Avoiding Computer-Specific Settings. |
Basic Concepts
You normally launch a web browser and open the tested web page in it at the beginning of a web test (see Launching Browsers). However, you may also need to navigate to web pages in an already running browser. For example, you may share the same browser instance among several test items. Or you may make sure that the desired browser is running using the approach described in the Checking if Browser Is Running help topic and then navigate the checked browser to the desired web page.
You can navigate to web pages in a running web browser in one of the following ways:
- By using navigation operations (as explained further in this topic).
- By simulating clicks on web page links (as explained in Simulating Mouse Wheel Rotation).
Navigating to Web Pages From Scripts
To navigate to an URL in a running browser, you can use one of the following methods:
-
Browsers.CurrentBrowser.Navigate
- navigates to an URL in the open web browser. The browser must be launched beforehand by using theBrowsers.Item(...).Run
method.JavaScript, JScript
function NavigateTest()
{
// Start the browser with a blank page
Browsers.Item(btIexplorer).Run();
// Navigate to an URL
Browsers.CurrentBrowser.Navigate("http://smartbear.com/");
}Python
def Navigate(): # Start the browser with a blank page Browsers.Item[btIExplorer].Run(); # Navigate to an URL Browsers.CurrentBrowser.Navigate("http://smartbear.com/");
VBScript
Sub NavigateTest
' Start the browser with a blank page
Browsers.Item(btIexplorer).Run
' Navigate to an URL
Browsers.CurrentBrowser.Navigate("http://smartbear.com/")
End SubDelphiScript
procedure NavigateTest;
begin
// Start the browser with a blank page
Browsers.Item(btIexplorer).Run();
// Navigate to an URL
Browsers.CurrentBrowser.Navigate('http://smartbear.com/');
end;C++Script, C#Script
function NavigateTest()
{
// Start the browser with a blank page
Browsers["Item"](btIexplorer)["Run"]();
// Navigate to an URL
Browsers["CurrentBrowser"]["Navigate"]("http://smartbear.com/");
} -
Aliases.browser.ToUrl
(if Name Mapping is used) orSys.Browser().ToUrl
(if Name Mapping is not used) - opens the specified URL in theBrowser
test object.JavaScript, JScript
function WebTest()
{
var Page;
// Start the browser with a blank page
Browsers.Item(btIexplorer).Run();
// Navigate to an URL
Page = Sys.Browser().ToUrl("http://smartbear.com/");
}Python
def BrowserToURL(): # Start the browser with a blank page Browsers.Item[btIExplorer].Run(); # Navigate to an URL Page = Sys.Browser().ToUrl("http://smartbear.com/");
VBScript
Sub WebTest
Dim Page
' Start the browser with a blank page
Browsers.Item(btIexplorer).Run
' Navigate to an URL
Set Page = Sys.Browser.ToUrl("http://smartbear.com/")
End SubDelphiScript
procedure WebTest;
var Page;
begin
// Start the browser with a blank page
Browsers.Item(btIexplorer).Run();
// Navigate to an URL
Page := Sys.Browser.ToUrl('http://smartbear.com/');
end;C++Script, C#Script
function WebTest()
{
var Page;
// Start the browser with a blank page
Browsers["Item"](btIexplorer)["Run"]();
// Navigate to an URL
Page = Sys["Browser"]()["ToUrl"]("http://smartbear.com/");
} -
Page.ToUrl
- opens the specified URL in thePage
test object.JavaScript, JScript
function PageToURL()
{
var Page;
// Start the browser
Browsers.Item(btIexplorer).Run("http://smartbear.com/");
// Get a web page test object
var Page = Sys.Browser().Page("*smartbear.com*");
// Navigate to an URL
Page.ToUrl("http://blog.smartbear.com");
}Python
def PageToURL(): # Start the browser Browsers.Item[btIExplorer].Run("http://smartbear.com/"); # Get a web page test object Page = Sys.Browser().Page("*smartbear.com*"); # Navigate to an URL Page.ToUrl("http://blog.smartbear.com");
VBScript
Sub PageToURL
Dim Page
' Start the browser
Browsers.Item(btIexplorer).Run("http://smartbear.com/")
' Get a web page test object
Set Page = Sys.Browser.Page("*smartbear.com*")
' Navigate to another page
Page.ToUrl("http://blog.smartbear.com")
End SubDelphiScript
procedure PageToURL;
var Page;
begin
// Start the browser
Browsers.Item(btIexplorer).Run('https://smartbear.com/');
// Get a web page test object
Page := Sys.Browser.Page('*smartbear.com*');
// Navigate to an URL
Page.ToUrl('http://blog.smartbear.com');
end;C++Script, C#Script
function PageToURL()
{
var Page;
// Start the browser
Browsers["Item"](btIexplorer)["Run"]("https://smartbear.com/");
// Get a web page test object
var Page = Sys["Browser"]()["Page"]("*smartbear.com*");
// Navigate to an URL
Page["ToUrl"]("http://blog.smartbear.com");
}
The main difference between the mentioned approaches is that the Browser.ToUrl
and Page.ToUrl
methods are applied to tested objects only. The Browser.CurrentBrowser.Navigate
method is a scripting method that is not applied to tested objects. Despite the mentioned difference, all the mentioned approaches work equally well and you can choose the one that suits your needs best.
Navigating to Web Pages From Keyword Tests
In keyword tests, use the Navigate operation (from the Web category) to attach to a running web browser and navigate it to the specified URL. Note that this operation does not run a new instance of the browser. It just navigates to an existing instance of the specified browser. The operation has the following parameters:
-
Browser - the web browser to attach to. You can set this parameter to CurrentBrowser or directly specify the name of the browser to attach to.
If the parameter is set to CurrentBrowser, the operation is equal to
Browsers.CurrentBrowser.Navigate
. The operation will navigate to an URL in the web browser that is current for your test. If a test does not have a current browser, an error occurs.You can also directly specify the browser name and, optionally, the version and bitness to attach to a specific browser. Note that specifying CurrentBrowser is more suitable for most cross-browser tests. If you create a test for one browser and need to execute it in any other browser, you will not need to modify parameters of all Navigate operations within your test.
-
Url - the URL to navigate to.
- WaitTime - the time to wait until the browser loads the page and becomes ready to accept user input. If this parameter is omitted, the timeout is specified by the project's Web page loading timeout option.
For more information on the operation’s parameters, see the description of the operation
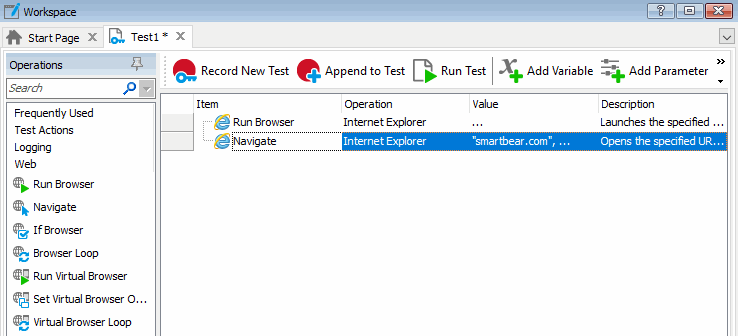
Alternatively, you can use the On-Screen Action, Call Object Method and Run Code Snippet keyword test operations to run the scripting methods mentioned above.
Web Testing Events
When running tests in Internet Explorer, TestComplete raises a number of events when web pages are opened in the browser:
You can handle these events in your test to perform specific operations when navigating to web pages. For more information, see Creating Event Handlers for TestComplete Events.
![]() |
These events are fired only when the test is run by using the Internet Explorer or Microsoft WebBrowser control. They are not raised when using other supported browsers. |
See Also
Testing Web Applications
Launching Browsers
Common Tasks for Web Testing
Waiting For Web Pages