In your tests, you may need to get a text block residing in the desired area of your tested application to simulate user actions on it:
By using search preference
When obtaining the needed text block by using the OCR.Recognize.BlockByText
method or the OCR Action operation, you can specify the area where the text block will be searched for (for example, at the bottom of the tested object). Usually, you use this approach to distinguish several text blocks that contain the same text.
In keyword tests
For an OCR Action operation, specify your search preference:
In scripts
When calling the OCR.Recognize.BlockByText
method, specify the SelectionPreference parameter. For example:
OCR.Recognize(testedObject).BlockByText("substring", spNearestToCenter)
By coordinates
Sometimes, specifying search preferences when getting a text block may not be enough. For example, there can be a situation where you cannot specify the preferred search area explicitly. In this case, you can specify the search area by its coordinates:
-
Get an image of the needed search area by its coordinates. To do this, use the
Picture
method that all onscreen objects have. -
Use the
OCR.Recognize
method to recognize the text the obtained image contains. -
Get the needed block of the recognized text by using either the
Block
property or theBlockByText
method. -
TestComplete cannot simulate user actions on text blocks obtained from images. It can simulate user actions only on text blocks it obtains from onscreen objects.
As a workaround, you can do the following:
-
Get the screen area to which the text block corresponds. To do this, you can use the
TextBlock.Bounds
property that contains information on the text block size and location. -
Simulate user actions on the screen area. You can simulate coordinate-based actions like clicks, double-clicks, touches (for mobile applications), and so on.
-
In scripts
The code below contains the GetTextBlockByCoordinates
routine that obtains a tested onscreen object, the coordinates of the search area and the text to search for. The routine returns the Rect
object that describes the area within the tested object where the text block resides.
The returned Rect
object can be used to simulate user actions on the appropriate area of the tested onscreen object containing the needed text. The Main
routine shows how to simulate a click in the center of the returned area.
JavaScript, JScript
{
var res = Utils.Rect;
// Get an image of the specified area of an onscreen object
var pic = anObject.Picture(x, y, width, height);
if (pic != null)
{
// Recognize the text in the image
var obj = OCR.Recognize(pic);
if (obj.FullText != "")
{
if (aqString.Find(obj.FullText, aText, 0, false ) > -1)
{
// Use the BlockByText method to find the specified text
// in the text recognized in the image
var block = obj.BlockByText(aText);
res.Left = block.Bounds.Left + x;
res.Top = block.Bounds.Top + y;
res.Bottom = res.Top + block.Bounds.Height;
res.Right = res.Left + block.Bounds.Width;
return res;
}
}
return null;
}
return null;
}
function Main()
{
var textToSearch = "substring";
// Get an onscreen object
var p = Sys.WaitProcess("MyApp").WaitWindow("Window", "*", -1, 1000);
// Get info on the area that contains the needed substring
// and resides at the specified coordinates
var b = GetTextBlockByCoordinates(p, 50, 250, 300, 50, textToSearch);
if (b != null)
{
// Simulate user actions
…
// Simulate a click in the center of the area
p.Click(b.Left + b.Width / 2, b.Top + b.Height / 2);
…
}
}
Python
def GetTextBlockByCoordinates(anObject, x, y, width, height, aText):
res = Utils.Rect;
# Get an image of the specified area of an onscreen object
pic = anObject.Picture(x, y, width, height);
if pic != None:
# Recognize the text in the image
obj = OCR.Recognize(pic)
if obj.FullText != "":
if aqString.Find(obj.FullText, aText, 0, False ) > -1:
# Use the BlockByText method to find the specified text
# in the text recognized in the image
block = obj.BlockByText(aText)
res.Left = block.Bounds.Left + x
res.Top = block.Bounds.Top + y
res.Bottom = res.Top + block.Bounds.Height
res.Right = res.Left + block.Bounds.Width
return res
return None
return None
def Main():
textToSearch = "substring"
# Get an onscreen object
p = Sys.WaitProcess("MyApp").WaitWindow("Window", "*", -1, 1000)
# Get info on the area that contains the needed substring
# and resides at the specified coordinates
b = GetTextBlockByCoordinates(p, 50, 270, 320, 55, textToSearch);
if b != None:
# Simulate user actions
# Simulate a click in the center of the area
p.Click(b.Left + b.Width / 2, b.Top + b.Height / 2)
VBScript
Set GetTextBlockByCoordinates = Nothing
' Get an image of the specified area of an onscreen object
Set pic = anObject.Picture(x, y, width, height)
If Not pic Is Nothing Then
' Recognize the text in the image
Set obj = OCR.Recognize(pic)
If obj.FullText <> "" Then
If aqString.Find(obj.FullText, aText, 0, False ) > -1 Then
' Use the BlockByText method to find the specified text
' in the text recognized in the image
Set res = Utils.Rect
Set block = obj.BlockByText(aText)
res.Left = block.Bounds.Left + x
res.Top = block.Bounds.Top + y
res.Bottom = res.Top + block.Bounds.Height
res.Right = res.Left + block.Bounds.Width
Set GetTextBlockByCoordinates = res
End If
End If
End If
End Function
Sub Main
textToSearch = "substring"
' Get an onscreen object
Set p = Sys.WaitProcess("MuApp").WaitWindow("Window", "*", -1, 1000)
' Get info on the area that contains the needed substring
' and resides at the specified coordinates
Set b = GetTextBlockByCoordinates(p, 50, 250, 300, 50, textToSearch)
If Not b Is Nothing Then
' Simulate user actions
…
' Simulate a click in the center of the area
Call p.Click(b.Left + b.Width / 2, b.Top + b.Height / 2)
…
End If
End Sub
DelphiScript
var res, pic, obj, block;
begin
result := nil;
// Get an image of the specified area of an onscreen object
pic := anObject.Picture(x, y, width, height);
if not (pic = nil) then
begin
// Recognize the text in the image
obj := OCR.Recognize(pic);
if obj.FullText <> '' then
begin
if aqString.Find(obj.FullText, aText, 0, false ) > -1 then
begin
// Use the BlockByText method to find the specified text
// in the text recognized in the imag
res := Utils.Rect;
block := obj.BlockByText(aText);
res.Left := block.Bounds.Left + x;
res.Top := block.Bounds.Top + y;
res.Bottom := res.Top + block.Bounds.Height;
res.Right := res.Left + block.Bounds.Width;
result : = res;
end;
end;
end;
end;
procedure Main();
var textToSearch, p, b;
begin
textToSearch := 'substring';
// Get an onscreen object
p := Sys.WaitProcess('MyApp').WaitWindow('Window', '*', -1, 1000);
// Get info on the area that contains the needed substring
// and resides at the specified coordinates
b := GetTextBlockByCoordinates(p, 50, 250, 300, 50, textToSearch);
if not (b = nil) then
begin
// Simulate user actions
…
// Simulate a click in the center of the area
p.Click(b.Left + b.Width / 2, b.Top + b.Height / 2);
…
end;
end;
C++Script, C#Script
{
var res = Utils["Rect"];
// Get an image of the specified area of an onscreen object
var pic = anObject["Picture"](x, y, width, height);
if (pic != null)
{
// Recognize the text in the image
var obj = OCR["Recognize"](pic);
if (obj["FullText"] != "")
{
if (aqString["Find"](obj["FullText"], aText, 0, false ) > -1)
{
// Use the BlockByText method to find the specified text
// in the text recognized in the image
var block = obj["BlockByText"](aText);
res.Left = block["Bounds"]["Left"] + x;
res.Top = block["Bounds"]["Top"] + y;
res.Bottom = res["Top"] + block["Bounds"]["Height"];
res.Right = res["Left"] + block["Bounds"]["Width"];
return res;
}
}
return null;
}
return null;
}
function Main()
{
var textToSearch = "substring";
// Get an onscreen object
var p = Sys["WaitProcess"]("MyApp")["WaitWindow"]("Window", "*", -1, 1000);
// Get info on the area that contains the needed substring
// and resides at the specified coordinates
var b = GetTextBlockByCoordinates(p, 50, 250, 300, 50, textToSearch);
if (b != null)
{
// Simulate user actions
…
// Simulate a click in the center of the area
p["Click"](b["Left"] + b["Width"] / 2, b["Top"] + b["Height"] / 2);
…
}
}
In keyword tests
-
Copy the
GetTextBlockByCoordinates
function code from the example above to a script unit in your project in TestComplete. -
Call the function from your keyword test by using the Run Script Routine operation.
-
Save the area the function returns to a variable.
To learn how to get the results of a routine called from a keyword test, see Checking Operation Result. To learn how to set a variable value in a keyword test, see Keyword Test Variables.
-
Simulate user actions on the area, for example, by using the Run Code Snippet operation.
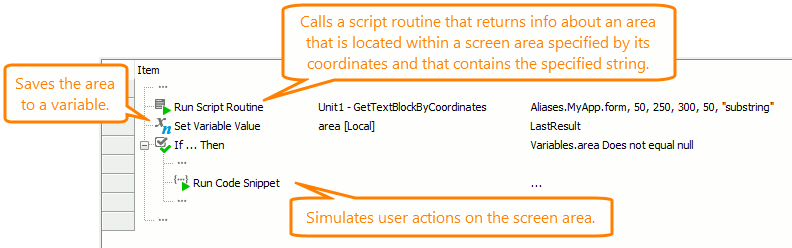
As an alternative, copy the Main
routine from the example above to a script unit and modify it to work with your tested objects and coordinates. After that, you can call that routine from your keyword test.
Important
TestComplete can also recognize text in images loaded from a file. However, it cannot simulate user actions on recognized text blocks. To simulate user actions on screen areas specified by images you load from files, we recommend that you perform Image-Based testing. See Image-Based Testing.
See Also
Optical Character Recognition
Picture Object
Image-Based Testing
Get Controls With No Text Contents