The object-driven testing (ODT) functionality is deprecated. Do not use it to create new tests. It will be removed from the product in one of the future releases. As an alternative, you can create custom classes in your scripts. For more information, see Alternatives to the ODT functionality.
Description
The custom objects you create with the ODT project item contain methods that you can call to perform testing actions. Using the Data.Run
method you can easily go through the object hierarchy and call the objects’ methods.
The method starts from the first child of the ODT | Data node and goes down the node hierarchy:
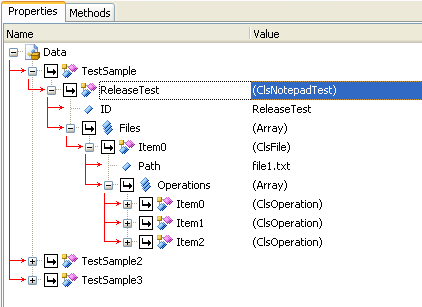
The method analyzes the node’s type and does the following:
-
If the node contains an object,
Data.Run
calls the methods of this object. -
If the node contains an ordinary value, no action is performed.
-
If the node contains an array, the method “processes” each item of this array.
After the method finishes “processing” the node, it continues with its child nodes. If there are no child nodes, the method proceeds with the next node in the hierarchy.
For complete information on how the method works, see the Controlling Object-Driven Tests topic.
Declaration
DataObj.Run()
DataObj | An expression, variable or parameter that specifies a reference to a Data object | |||
Result | None |
Applies To
The method is applied to the following object:
Result Value
None.
Example
The code below creates a custom object hierarchy and then walks down the object tree.
JavaScript, JScript
function DataObjExample()
{
// Creates a custom object tree
var Group = ODT.Data.AddGroup("NewDataGroup");
var Var1 = Group.AddVariable("MyVariable", 123);
var Var2 = Group.AddVarOfArrayType.AddItem("MyText");
// Specifies the object that will be used to specify
// a variable value
var Obj = Sys.Process("MyApplication");
var Var3 = Group.AddVariable(Obj);
// ...
// Walks down the custom object tree
ODT.Data.Run();
}
VBScript
Sub DataObjExample
' Creates a custom object tree
Set Group = ODT.Data.AddGroup("NewDataGroup")
Set Var1 = Group.AddVariable("MyVariable", 123)
Set Var2 = Group.AddVarOfArrayType.AddItem("MyText")
' Specifies the object that will be used to specify
' a variable value
Set Obj = Sys.Process("MyApplication")
Set Var3 = Group.AddVariable(Obj)
' ...
' Walks down the custom object tree
ODT.Data.Run()
End Sub
DelphiScript
function DataObjExample;
var
Group, Var1, Var2, Obj, Var3;
begin
// Creates a custom object tree
Group := ODT.Data.AddGroup('NewDataGroup');
Var1 := Group.AddVariable('MyVariable', 123);
Var2 := Group.AddVarOfArrayType.AddItem('MyText');
// Specifies the object that will be used to specify
// a variable value
Obj := Sys.Process('MyApplication');
Var3 := Group.AddVariable(Obj);
// ...
// Walks down the custom object tree
ODT.Data.Run();
end;
C++Script, C#Script
function DataObjExample()
{
// Creates a custom object tree
var Group = ODT["Data"]["AddGroup"]("NewDataGroup");
var Var1 = Group["AddVariable"]("MyVariable", 123);
var Var2 = Group["AddVarOfArrayType"]["AddItem"]("MyText");
// Specifies the object that will be used to specify
// a variable value
var Obj = Sys["Process"]("MyApplication");
var Var3 = Group["AddVariable"](Obj);
// ...
// Walks down the custom object tree
ODT["Data"]["Run"]();
}
See Also
Controlling Object-Driven Tests
Run Method
Run Method
Run Method