Description
This object has a mechanism that allows you to work with sections and options stored in the system registry, INI, XML and binary files. To illustrate what sections and options are, let’s have a look at the registry:
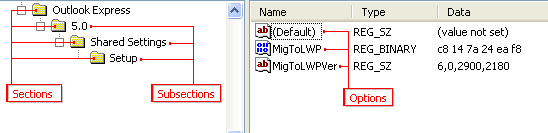
The structure used in binary, INI and XML files is similar to this one.
A section can hold any number of subsections. A section (or a subsection) can hold any number of options. An option can hold data of the string, integer, float or date/time formats.
A Section
object is returned by the Registry
method of the Storages
object. This method returns the Section
object for a system registry key. Using methods and properties of the Section
object, you can create and delete sections and options in any of the mentioned sources. Also, you can get and change the contents of the sections and options.
The GetSubSection
and GetSubSectionByIndex
methods of the Section
object return Section
objects for a section. Thus, this section becomes another start point in the structure of subsections and options you can manage using the methods and properties of the Section
objects.
The Section
object is an ancestor of the FileSection
object. FileSection
is meant for working with sections and options stored in XML, INI or binary files. You use the same methods and properties as in Section
, since FileSection
derives all of them from Section
. The way you use the methods and properties of the Section
object does not depend on where the sections and options are kept.
Members
Example
The following example demonstrates how to get a Section
object that provides scripting access to the Windows system registry key:
JavaScript, JScript
function Test()
{
var Key, RootKey, Section, RegistryType, RO;
// Specifies the full path to the needed registry key from the root key
Key = "Software\\Microsoft\\Windows\\CurrentVersion\\Explorer\\Shell Folders";
// Specifies the root key
RootKey = HKEY_CURRENT_USER;
// Specifies that the 32-bit registry view will be accessed
RegistryType = AQRT_32_BIT;
// Specifies that the registry key is opened in read-only mode
RO = true;
// Obtains a Section object that provides access to the specified registry key
Section = Storages.Registry(Key, RootKey, RegistryType, RO);
…
}
Python
def Test():
# Specifies the full path to the needed registry key from the root key
Key = "Software\\Microsoft\\Windows\\CurrentVersion\\Explorer\\Shell Folders"
# Specifies the root key
RootKey = HKEY_CURRENT_USER
# Specifies that the 32-bit registry view will be accessed
RegistryType = AQRT_32_BIT
# Specifies that the registry key is opened in read-only mode
RO = True
# Obtains a Section object that provides access to the specified registry key
Section = Storages.Registry(Key, RootKey, RegistryType, RO)
Log.Message(Section.Name)
# ...
VBScript
Sub Test
Dim Key, RootKey, Section, RegistryType, RO
' Specifies the full path to the needed registry key from the root key
Key = "Software\Microsoft\Windows\CurrentVersion\Explorer\Shell Folders"
' Specifies the root key
RootKey = HKEY_CURRENT_USER
' Specifies that the 32-bit registry view will be accessed
RegistryType = AQRT_32_BIT
' Specifies that the registry key is opened in read-only mode
RO = True
' Obtains a Section object that provides access to the specified registry key
Set Section = Storages.Registry(Key, RootKey, RegistryType, RO)
…
End Sub
DelphiScript
procedure Test();
var Key, RootKey, Section, RegistryType, RO, valueName;
begin
// Specifies the full path to the desired registry key from the root key
Key := 'Software\Microsoft\Windows\CurrentVersion\Explorer\Shell Folders';
// Specifies the root key
RootKey := HKEY_CURRENT_USER;
// Specifies that the 32-bit registry view will be accessed
RegistryType := AQRT_32_BIT;
// Specifies that registry key is opened in a read-only mode
RO := true;
// Obtains a Section object that provides an access to the specified registry key
Section := Storages.Registry(Key, RootKey, RegistryType, RO);
…
end;
C++Script, C#Script
function Test()
{
var Key, RootKey, Section, RegistryType, RO;
// Specifies the full path to the desired registry key from the root key
Key = "Software\\Microsoft\\Windows\\CurrentVersion\\Explorer\\Shell Folders";
// Specifies the root key
RootKey = HKEY_CURRENT_USER;
// Specifies that the 32-bit registry view will be accessed
RegistryType = AQRT_32_BIT;
// Specifies that registry key is opened in a read-only mode
RO = true;
// Obtains a Section object that provides an access to the specified registry key
Section = Storages["Registry"](Key, RootKey, RegistryType, RO);
…
}
See Also
Storages Object
FileSection Object
Working With XML Files From Scripts