TestComplete samples (both built-in and additional) are located in the <Users>\Public\Public Documents\TestComplete 15 Samples folder.
Some file managers display the Public Documents folder as Documents.
![]() |
The information below concerns legacy mobile tests that work with mobile devices connected to the local computer. For new mobile tests, we recommend using the newer cloud-compatible approach. |
Most mobile devices have built-in sensors - accelerometers, gravity sensors, light sensors, magnetometers and others. Sensors provide various data about the environment. Different device models have a different set of sensors.
You can read sensor data using TestComplete. For example, you can use TestComplete with third-party sensor simulators to test if your mobile application reacts to changed sensor data correctly.
This topic explains how you can read sensor data from automated tests. You can also get and set a GPS location as explained in Geolocation Testing (Legacy).
Requirements
Android
-
The TestComplete Android Agent service must be installed and running on the device.
-
The device must be connected to Android Debug Bridge.
iOS
-
A prepared application must be running on the device.
Test Objects for Sensors
You can get the number of device sensors using the Device.SensorsCount
property, and enumerate the sensors using Device.Sensor(Index)
.
Android device sensors are represented by the AndroidSensor
object. You can get a sensor’s Name
, Type
and Values
– Value0
, Value1
and Value2
. For a list of Android sensor types, refer to the Android developer documentation:
iOS device sensors are represented by the iOSSensor
object. You can get a sensor’s Name
and values:
-
For an accelerometer, gyroscope and magnetometer -
X
,Y
andZ
. -
For an attitude -
Pitch
,Roll
andYaw
.
Remarks
TestComplete reads Android sensor values only when you access sensorObj.Values
. So, always read the values directly from the sensor object using the sensorObj.Values.Value[0-2]
syntax:
JavaScript, JScript
var sensor = Mobile.Device("MyDevice").Sensors(0);
Log.Message(sensor.Values.Value0);
Delay(1000);
Log.Message(sensor.Values.Value0); // new value
Python
sensor = Mobile.Device("MyDevice").Sensors(0);
Log.Message(sensor.Values.Value0);
Delay(1000);
Log.Message(sensor.Values.Value0); # new value
VBScript
Dim sensor
Set sensor = Mobile.Device("MyDevice").Sensors(0)
Call Log.Message(sensor.Values.Value0)
Call Delay(1000)
Call Log.Message(sensor.Values.Value0) ' new value
DelphiScript
sensor := Mobile.Device('MyDevice').Sensors(0);
Log.Message(sensor.Values.Value0);
Delay(1000);
Log.Message(sensor.Values.Value0); // new value
C++Script, C#Script
var sensor = Mobile["Device"]("MyDevice")["Sensors"](0);
Log["Message"](sensor["Values"]["Value0"]);
Delay(1000);
Log["Message"](sensor["Values"]["Value0"]); // new value
If you save a sensor’s Values
object to a variable, this variable will return the same cached values:
JavaScript, JScript
var sensor = Mobile.Device("MyDevice").Sensors(0);
var values = sensor.Values;
Log.Message(values.Value0);
Delay(1000);
Log.Message(values.Value0); // still the old value
Python
sensor = Mobile.Device("MyDevice").Sensors(0);
values = sensor.Values;
Log.Message(values.Value0);
Delay(1000);
Log.Message(values.Value0); # still the old value
VBScript
Dim sensor, values
Set sensor = Mobile.Device("MyDevice").Sensors(0)
Set values = sensor.Values
Call Log.Message(values.Value0)
Call Delay(1000)
Call Log.Message(values.Value0) ' still the old value
DelphiScript
sensor := Mobile.Device('MyDevice').Sensors(0);
values := sensor.Values;
Log.Message(values.Value0);
Delay(1000);
Log.Message(values.Value0); // still the old value
C++Script, C#Script
var sensor = Mobile["Device"]("MyDevice")["Sensors"](0);
var values = sensor["Values"];
Log["Message"](values["Value0"]);
Delay(1000);
Log["Message"](values["Value0"]); // still the old value
Also, because of this, clicking Refresh All in the Object Browser, when it shows the
Values
object properties, does not refresh the sensor values. You need to “re-enter” the Values
object to see the new sensor values.
Getting Sensor Data From Scripts
The script below demonstrates how you can list Android device sensors and their values.
JavaScript, JScript
function ListSensors()
{
var sensor, i;
Mobile.SetCurrent("Nexus 7");
for (i = 0; i < Mobile.Device().SensorsCount; i++)
{
sensor = Mobile.Device().Sensor(i);
Log.AppendFolder("Sensor " + i + ": " + sensor.Name);
Log.Message(sensor.Type);
Log.Message("Value0: " + sensor.Values.Value0);
Log.Message("Value1: " + sensor.Values.Value1);
Log.Message("Value2: " + sensor.Values.Value2);
Log.PopLogFolder();
}
}
Python
def ListSensors():
Mobile.SetCurrent("Nexus 7")
for i in range (0, Mobile.Device().SensorsCount):
sensor = Mobile.Device().Sensor[i]
Log.AppendFolder("Sensor " + IntToStr(i) + ": " + sensor.Name)
Log.Message(sensor.Type)
Log.Message("Value0: " + VarToStr(sensor.Values.Value0))
Log.Message("Value1: " + VarToStr(sensor.Values.Value1))
Log.Message("Value2: " + VarToStr(sensor.Values.Value2))
Log.PopLogFolder()
VBScript
Sub ListSensors
Dim sensor, i
Call Mobile.SetCurrent("Nexus 7")
For i = 0 To Mobile.Device.SensorsCount - 1
Set sensor = Mobile.Device.Sensor(i)
Call Log.AppendFolder("Sensor " & i & ": " & sensor.Name)
Call Log.Message(sensor.Type)
Call Log.Message("Value0: " & sensor.Values.Value0)
Call Log.Message("Value1: " & sensor.Values.Value1)
Call Log.Message("Value2: " & sensor.Values.Value2)
Call Log.PopLogFolder
Next
End Sub
DelphiScript
procedure ListSensors;
var sensor, i;
begin
Mobile.SetCurrent('Nexus 7');
for i := 0 to Mobile.Device.SensorsCount - 1 do
begin
sensor := Mobile.Device.Sensor(i);
Log.AppendFolder('Sensor ' + aqConvert.VarToStr(i) + ': ' + sensor.Name);
Log.Message(sensor.Type);
Log.Message('Value0: ' + aqConvert.VarToStr(sensor.Values.Value0));
Log.Message('Value1: ' + aqConvert.VarToStr(sensor.Values.Value1));
Log.Message('Value2: ' + aqConvert.VarToStr(sensor.Values.Value2));
Log.PopLogFolder;
end;
end;
C++Script, C#Script
function ListSensors()
{
var sensor, i;
Mobile["SetCurrent"]("Nexus 7");
for (i = 0; i < Mobile["Device"]["SensorsCount"]; i++)
{
sensor = Mobile["Device"]["Sensor"](i);
Log["AppendFolder"]("Sensor " + i + ": " + sensor["Name"]);
Log["Message"](sensor.Type);
Log["Message"]("Value0: " + ["sensor"]["Values"]["Value0"]);
Log["Message"]("Value1: " + ["sensor"]["Values"]["Value1"]);
Log["Message"]("Value2: " + ["sensor"]["Values"]["Value2"]);
Log["PopLogFolder"]();
}
}
Getting Sensor Data From Keyword Tests
You can access the device sensor objects in keyword tests using the Call Object Method or Run Code Snippet operation. To use sensor values in other operation parameters, use the Code Expression parameter mode. For details, see Getting and Setting Object Property Values.
You can also write a script for getting sensor data and call it from your keyword test using the Run Script Routine operation.
Below is a sample keyword test that lists all Android device sensors and their values.
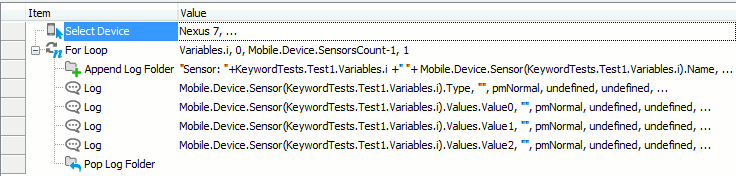
A keyword test that gets sensor data
Samples
TestComplete includes a sample project that demonstrates how to get data from the mobile devices' sensors:
Android
<TestComplete Samples>\Mobile\Android\Sensors
iOS
<TestComplete Samples>\Mobile\iOS\Sensors
Note: | If you do not have the sample, download the TestComplete Samples installation package from the support.smartbear.com/testcomplete/downloads/samples page of our website and run it. |
See Also
Geolocation Testing and Sensors (Legacy)
AndroidSensor Object
iOSSensor Object
Common Tasks for Mobile Testing (Legacy)
Testing Mobile Applications