General Notes
TestComplete can recognize action bar controls of the Android Open Applications. It allows you to simulate actions on the action bar using its methods and properties.
Specifics of Working With Action Bars
TestComplete supports two groups of action bar elements: menu items and navigation items. To work with each of these groups, you should use different sets of methods and properties. For example, the TouchItem
action simulates a touch on a menu item, and the TouchNavItem
action simulates a touch on an item of the navigation menu. The images below demonstrate how these menus can be shown on a device’s screen.
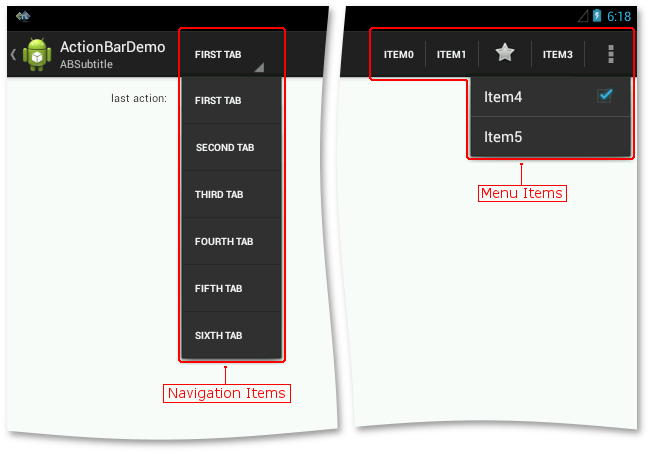
A possible location of action bar items with the landscape screen orientation.
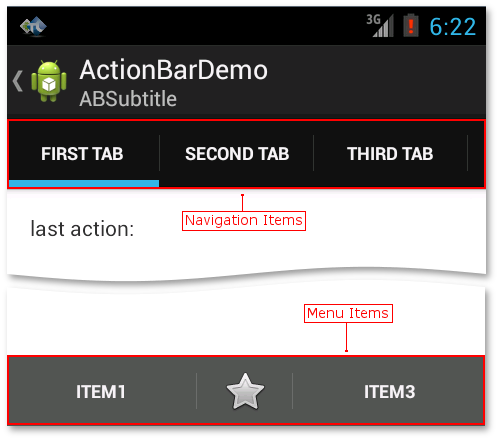
A possible location of action bar items with the portrait screen orientation.
It does not matter whether the action bar is split, you can work with it using the methods and properties of the Android ActionBar
object.
In order to work with other elements that the action bar contains (buttons, switches and so on), you need to find them in the Object Browser. To learn how to do this, see Addressing Objects in Android Open Applications (Legacy).
Addressing Action Bar Items
The information below describes how to specify action bar items in your tests:
Using Item Indexes and Names
It is possible to specify an action bar item by its name or index. In most cases, you can specify item by their names, in the following way:
actionbarObj.TouchItem("Item1") // Touch a menu item
actionbarObj.TouchNavItem("Tab1") // Touch a navigation item
The sample script below simulates a touch on the "Item1" menu item and on the "Tab1" navigation menu item of an application’s action bar.
JavaScript, JScript
function TouchItem()
{
// Select an Android device
Mobile.SetCurrent("MyDevice");
// Obtain an application
var app = Mobile.Device().Process("com.example.myapp");
// Obtain an action bar
var actionbarObj = app.RootLayout("").Layout("action_bar_container").ActionBar("action_bar");
// Simulating touch on menu item
actionbarObj.TouchItem("Item1");
// Simulating touch on navigation item
actionbarObj.TouchNavItem("Tab1");
}
Python
def TouchItem():
# Select an Android device
Mobile.SetCurrent("MyDevice")
# Obtain an application
app = Mobile.Device().Process("com.example.myapp")
# Obtain an action bar
actionbarObj = app.RootLayout("").Layout("action_bar_container").ActionBar("action_bar")
# Simulating touch on menu item
actionbarObj.TouchItem("Item1")
# Simulating touch on navigation item
actionbarObj.TouchNavItem("Tab1")
VBScript
Sub TouchItem
Dim app, actionbarObj
' Select an Android device
Mobile.SetCurrent("MyDevice")
' Obtain an application
Set app = Mobile.Device.Process("com.example.myapp")
' Obtain an action bar
Set actionbarObj = app.RootLayout("").Layout("action_bar_container").ActionBar("action_bar")
' Simulating touch on a menu item
Call actionbarObj.TouchItem("Item1")
' Simulating touch on a navigation item
Call actionbarObj.TouchNavItem("Tab1")
End Sub
DelphiScript
procedure TouchItem();
var
app, actionbarObj: OleVariant;
begin
// Select an Android device
Mobile.SetCurrent('MyDevice');
// Obtain an application
app := Mobile.Device.Process('com.example.myapp');
// Obtain an action bar
actionbarObj := app.RootLayout('').Layout('action_bar_container').ActionBar('action_bar');
// Simulating touch on a menu item
actionbarObj.TouchItem('Item1');
// Simulating touch on a navigation menu
actionbarObj.TouchNavItem('Tab1');
end;
C++Script, C#Script
function TouchItem()
{
// Select an Android device
Mobile["SetCurrent"]("MyDevice");
// Obtain an application
var app = Mobile["Device"]["Process"]("com.example.myapp");
// Obtain an action bar
var actionbarObj = app["RootLayout"]("")["Layout"]("action_bar_container")["ActionBar"]("action_bar");
// Simulating touch on a menu item
actionbarObj["TouchItem"]("Item1");
// Simulating touch on a navigation menu
actionbarObj["TouchItem"]("Tab1");
}
If the action bar does not display the text of items, you can find it using the properties of the Android ActionBar. Use the wNavItemText
property to obtain the name of a menu item and the wTextNavItem
property to obtain the name of a navigation menu item.
If an action bar item does not have a name, you can specify it using its index (in other words, the position within the action bar). Indexes are zero-based: the first item of each group in the action bar has index 0, the second - 1, and so on. The total number of items of an action bar menu is specified by the wItemCount
property. The last item will have index wItemCount - 1
. To obtain the total number of navigation menu items, you can use the wNavItemCount
property. In this case, the last item will have index wNavItemCount - 1
. For instance, the instruction below simulates a touch on the fifth menu item and on the second navigation menu item:
actionbarObj.TouchItem(4) // Touch a menu item
actionbarObj.TouchNavItem(1) // Touch a navigation menu item
The sample script below simulates a touch on the first menu item and on the last navigation menu item of an application’s action bar and posts their names to the log.
JavaScript, JScript
function TouchItem()
{
// Select an Android device
Mobile.SetCurrent("MyDevice");
// Obtain an application
var app = Mobile.Device().Process("com.example.myapp");
// Obtain an action bar
var actionbarObj = app.RootLayout("").Layout("action_bar_container").ActionBar("action_bar");
// Simulating a touch on the first menu item
actionbarObj.TouchItem(0);
// Posting its name to the log
Log.Message(actionbarObj.wItemText(0));
// Simulating a touch on the last navigation menu item
actionbarObj.TouchNavItem(actionbarObj.wNavItemCount - 1);
// Posting its name to the log
Log.Message(actionbarObj.wNavItemText(actionbarObj.wNavItemCount - 1));
}
Python
def TouchItem():
# Select an Android device
Mobile.SetCurrent("MyDevice")
# Obtain an application
app = Mobile.Device().Process("com.example.myapp")
# Obtain an action bar
actionbarObj = app.RootLayout("").Layout("action_bar_container").ActionBar("action_bar")
# Simulating a touch on the first menu item
actionbarObj.TouchItem(0)
# Posting its name to the log
Log.Message(actionbarObj.wItemText[0])
# Simulating a touch on the last navigation menu item
actionbarObj.TouchNavItem(actionbarObj.wNavItemCount - 1)
# Posting its name to the log
Log.Message(actionbarObj.wNavItemText[actionbarObj.wNavItemCount - 1])
VBScript
Sub TouchItem
Dim app, actionbarObj
' Select an Android device
Mobile.SetCurrent("MyDevice")
' Obtain an application
Set app = Mobile.Device.Process("com.example.myapp")
' Obtain an action bar
Set actionbarObj = app.RootLayout("").Layout("action_bar_container").ActionBar("action_bar")
' Simulating s touch on the first menu item
Call actionbarObj.TouchItem(0)
' Posting its name to the log
Call Log.Message(actionbarObj.wItemText(0))
' Simulating a touch on the last navigation menu item
Call actionbarObj.TouchNavItem(actionbarObj.wNavItemCount - 1)
' Posting its name to the log
Call Log.Message(actionbarObj.wNavItemText(actionbarObj.wNavItemCount - 1))
End Sub
DelphiScript
procedure TouchItem();
var
app, actionbarObj: OleVariant;
begin
// Select an Android device
Mobile.SetCurrent('MyDevice');
// Obtain an application
app := Mobile.Device.Process('com.example.myapp');
// Obtain an action bar
actionbarObj := app.RootLayout('').Layout('action_bar_container').ActionBar('action_bar');
// Simulating a touch on the first menu item
actionbarObj.TouchItem(0);
// Posting its name to the log
Log.Message(actionbarObj.wItemText(0));
// Simulating a touch on the last navigation menu item
actionbarObj.TouchNavItem(actionbarObj.wNavItemCount - 1);
// Posting its name to the log
Log.Message(actionbarObj.wNavItemText(actionbarObj.wNavItemCount - 1));
end;
C++Script, C#Script
function TouchItem()
{
// Select an Android device
Mobile["SetCurrent"]("MyDevice");
// Obtain an application
var app = Mobile["Device"]["Process"]("com.example.myapp");
// Obtain an action bar
var actionbarObj = app["RootLayout"]("")["Layout"]("action_bar_container")["ActionBar"]("action_bar");
// Simulating a touch on the first menu item
actionbarObj["TouchItem"](0)
// Posting its name to the log
Log["Message"](actionbarObj["wItemText"](0));
// Simulating a touch on the last navigation menu item
actionbarObj["TouchItem"](actionbarObj.wNavItemCount - 1);
// Posting its name to the log
Log["Message"](actionbarObj["wNavItemText"](actionbarObj["wNavItemCount"] - 1));
}
Using Case-Sensitive and Case-Insensitive Item Captions
Depending on the Use case-sensitive parameters project option, TestComplete treats titles of action bar buttons as case-sensitive or case-insensitive. By default, this option is turned off so that you can specify the item title in any case (all upper case, all lower case, mixed case, and so on):
actionbarObj.TouchItem("Item1")
actionbarObj.TouchItem("ITEM1")
actionbarObj.TouchItem("item1")
actionbarObj.TouchItem("ItEm1")
If Use case-sensitive parameters is checked, you can only specify item titles using the correct case:
actionbarObj.TouchItem("Item1") // Correct
actionbarObj.TouchItem("item1") // Incorrect!!!
Using Wildcards and Regular Expressions in Items Captions
If titles of action bar items change according to the current context, it may be useful to specify items only by constant parts of their names. To specify arbitrary parts in item names, TestComplete lets you use wildcard characters (* and ?) or regular expressions. The wildcard asterisk (*) corresponds to a string of any length (including an empty string), the wildcard question mark corresponds to any single character (including none). To specify more complicated parts of a caption, use regular expressions.
For example, the tested application’s action bar contains the Undo menu item whose title depends on the undo buffer: if there is an action to undo, the item's title contains the Undo word and the action name (for example, Undo Typing or Undo Paste); if there are no actions to undo, the item's title is Can't Undo. In order to ensure stable recognition of the Undo menu item whatever name it has, you can replace the variable part of the name with the * wildcard:
actionbarObj.TouchItem("*Undo*")
In this example, we place the wildcard on both sides of the Undo word, since it can either precede or follow other text.
See Also
Working With Android Action Bar Controls
Android ActionBar Support