The “Test object was not found” Error (ObjectTreeNodeNotFoundException)
This error occurs when you are trying to obtain a test object by using the Find
method, and the search criteria you use in the method call do not work for the object.
A possible reason for this is that the desired object does not exist in the tested application. For example, an application form or dialog had not been displayed by the moment the method was called. Check if the desired object is in the application (for example, if the form or dialog is visible on screen). If it is not there, modify your test (for example, specify a longer timeout parameter of the Find
method).
If the object exists in the application, then this error means that the search criteria you use do not work. If they worked earlier, this means the application has changed and they do not work anymore. In any case, you need to change the criteria.
Before changing the criteria in test code, you need to learn which object was not found. Tests often use a cascade of Find
methods (see below). The error occurs if any of these objects is missing. The exception’s call stack records typically point to the topmost Find
method in the cascade, which does not correspond to the missing object. For example, in web applications, you may have “stable” objects on the upper and lower levels and lots of “intermediate” containers that developers frequently remove or add:
C#
{
ObjectIdentifier = "chrome"
}).Find<IWebPage>(new WebPagePattern()
{
URL = "https://myWebSite/myPage.aspx",
ObjectGroupIndex = 0
}).Find<IControl>(new WebElementPattern()
{
ObjectType = "Panel",
idStr = "container"
}).Find<IControl>(new WebElementPattern()
{
ObjectType = "Nav",
idStr = "main-topnav"
}).Find<IControl>(new WebElementPattern()
{
ObjectType = "Link",
href = "myOrderForm.aspx",
contentText = "Order"
});
Visual Basic .NET
{
ObjectIdentifier = "chrome"
}).Find(Of IWebPage)(new WebPagePattern()
{
URL = "https://myWebSite/myPage.aspx",
ObjectGroupIndex = 0
}).Find(Of IControl)(new WebElementPattern()
{
ObjectType = "Panel",
idStr = "container"
}).Find(Of IControl)(new WebElementPattern()
{
ObjectType = "Nav",
idStr = "main-topnav"
}).Find(Of IControl)(new WebElementPattern()
{
ObjectType = "Link",
href = "myOrderForm.aspx",
contentText = "Order"
})
Java
{{
ObjectIdentifier = "chrome";
}}).find(WebPage.class, new WebPagePattern()
{{
URL = "https://myWebSite/myPage.aspx";
ObjectGroupIndex = 0;
}}).find(Control.class, new WebElementPattern()
{{
ObjectType = "Panel";
idStr = "container";
}}).find(Control.class, new WebElementPattern()
{{
ObjectType = "Nav";
idStr = "main-topnav";
}}).find(Control.class, new WebElementPattern()
{{
ObjectType = "Link";
href = "myOrderForm.aspx";
contentText = "Order";
}});
To learn which object was not found, take a look at the exception details. It has a line like “The search started from:”. It specifies the object that was found last. The objects below it in the search hierarchy were not found:
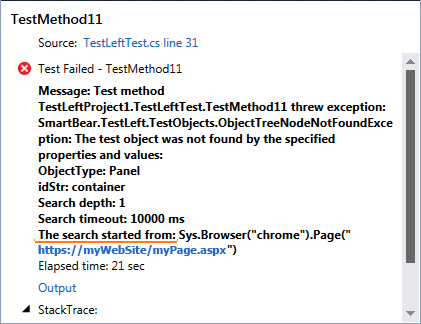
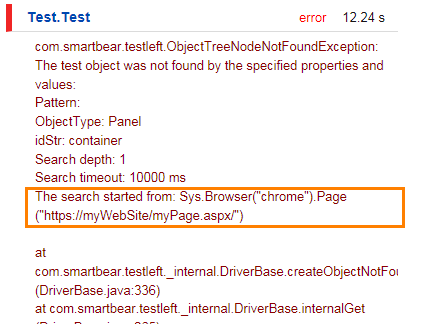
To solve the problem, you can —
-
Select the object from screen anew and use new identification code. See also Generating Identification Code Using UI Spy.
-
Use more specific search conditions. Add custom properties to the search pattern or use “greedier” regular expressions.
-
Remove the “intermediate” objects from the code and increase the depth parameter of the
Find
method to search for the desired object on deeper levels of the object hierarchy. For instance, for the above-mentioned code, you can remove the “intermediate” objects and increase the depth parameter for the lastFind
method:C#
IControl link = Driver.Find<IWebBrowser>(new WebBrowserPattern()
{
ObjectIdentifier = "chrome"
}).Find<IWebPage>(new WebPagePattern()
{
URL = "https://myWebSite/myPage.aspx",
ObjectGroupIndex = 0
}).Find<IControl>(new WebElementPattern()
{
ObjectType = "Link",
href = "myOrderForm.aspx",
contentText = "Order"
}, 10);Visual Basic .NET
IControl link = Driver.Find(Of IWebBrowser)(new WebBrowserPattern()
{
ObjectIdentifier = "chrome"
}).Find(Of IWebPage)(new WebPagePattern()
{
URL = "https://myWebSite/myPage.aspx",
ObjectGroupIndex = 0
}).Find(Of IControl)(new WebElementPattern()
{
ObjectType = "Link",
href = "myOrderForm.aspx",
contentText = "Order"
}, 10)Java
Control link = driver.find(WebBrowser.class, new WebBrowserPattern()
{{
ObjectIdentifier = "chrome";
}}).find(WebPage.class, new WebPagePattern()
{{
URL = "https://myWebSite/myPage.aspx";
ObjectGroupIndex = 0;
}}).find(Control.class, new WebElementPattern()
{{
ObjectType = "Link";
href = "myOrderForm.aspx";
contentText = "Order";
}}, 10);For information on how to use the depth parameter, see the Search Depth section in the Understanding Object Identification topic.
The “Invoked object is missing” Error
This error occurs when you call a method or property of some object that does not exist at the moment of the call. That is, the object was destroyed –
- After your test found the object in the system, and
- Before your test called a method or property of this object.
To find out the exact cause, explore the test log. For example, refer to the stack trace in the test results. It will help you learn where the problematic call is in the source code.
To understand what happened in the tested application, you can also use the TestLeft log:
-
In test results, in Visual Studio’s Test Explorer panel, click Output. This will open the Test Output page.
-
On the Test Output page, in the Attachments section, click index.htm. This will open the TestLeft results in your default web browser. Explore the messages to learn what actions were simulated.
-
Configure your test code to save the test results. To learn how to do it, see Saving Test Logs.
-
After the test run is over, open the TestLeft test log. Explore the messages to learn what actions were simulated.
If the issue persists, you can add commands to your test that will post screenshots of the tested application and its forms to the TestLeft log. You can then view these screenshots in the log to understand what happened in your application during the test run.
Test Code Selects and Works With Another Object
During test execution, TestLeft test code can select a wrong object and simulate user actions on it.
This happens due to ambiguous recognition, that is, because the operating system has several objects that match the search criteria you use. When TestLeft is searching for objects, it selects the first object that matches the specified search pattern. The search order depends on the order in which the operating system returns information about windows and controls. This order may differ from one operating system to another, or even from one user session to another. So, if the recognition criteria are “wide” enough, TestLeft may select a wrong object. This often happens after the application is changed, for instance, after some new control or dialog has been added to it.
A workaround is to update the object recognition code and specify more precise search criteria. For example, you can add custom properties to your search patten or use a more specific regular expression. See Understanding Object Identification for details.