General Notes
TestComplete can recognize table view controls of the iOS Open Applications. It allows you to simulate actions on the table view using its methods and properties.
Specifics of Working With Table View Controls
The IOS TableView object layout is split into sections. Each section may contain any number of items, and each item may also include item details. The following image shows how these elements are placed:
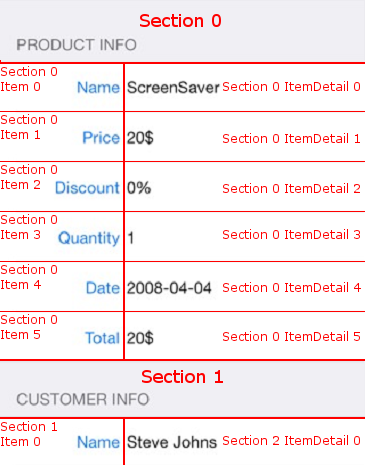
The TableView object provides support for working with these elements.
The information below describes how to specify table view items in your tests:
Addressing Sections
The structure of the table view places items in sections. Each section may have its own title. To address the item from the section, use the wSection property of the TableView object. To address the section, use its name or index:
tableview.TouchItem("Section1", "Item1") // Touch the item from section "Section1"
Addressing Items
Using Item Indexes and Names
It is possible to specify an item using section and item names or indexes. In most cases, you can use item and section names, in the following way:
tableview.TouchItem("Item1", "Section1") // Touch an item
The sample script below simulates a touch on the "Name" item from the "Product Info" section of an application’s table view.
JavaScript, JScript
function Test()
{
// Select the mobile device
Mobile.SetCurrent("iPhone");
// Obtain the TableView object
var p = Mobile.Device().Process("SampleApp");
var tableview = p.Window().TableView();
// Touch the item
tableview.TouchItem("Product Info", "Name");
}
Python
def Test():
# Select the mobile device
Mobile.SetCurrent("iPhone")
# Obtain the TableView object
p = Mobile.Device().Process("SampleApp")
tableview = p.Window().TableView()
# Touch the item
tableview.TouchItem("Product Info", "Name")
VBScript
Sub Test()
Dim p, tableview
' Select the mobile device
Mobile.SetCurrent("iPhone")
' Obtain the TableView object
Set p = Mobile.Device.Process("SampleApp")
Set tableview = p.Window().TableView()
' Touch the item
Call tableview.TouchItem("Product Info", "Name")
End Sub
DelphiScript
procedure Test();
var
p, tableview;
begin
// Select the mobile device
Mobile.SetCurrent('iPhone');
// Obtain the TableView object
p := Mobile.Device.Process('SampleApp');
tableview := p.Window(0).TableView(0);
// Touch the item
tableview.TouchItem('Product Info', 'Name');
end;
C++Script, C#Script
function Test()
{
// Select the mobile device
Mobile["SetCurrent"]("iPhone");
// Obtain the TableView object
var p = Mobile["Device"]["Process"]("SampleApp");
var tableview = p["Window"]()["TableView"]();
// Touch the item
tableview["TouchItem"]("Product Info", "Name");
}
If the table view does not display the text of items, you can find it using the properties of the iOS TableView object. Use the wItem
property to obtain the name of an item.
If an item does not have a name, you can specify it using its index (in other words, the position). Indexes are zero-based: the first item of each section in the table view has index 0, the second - 1, and so on. The total number of items is specified by the wItemCount
property. The last item will have index wItemCount - 1
. To obtain the total number of items in the section, you can use the wItemCount
property. In this case, the last item will have index wItemCount - 1
. For instance, the example below simulates a touch on the first item and a long touch on the third item of the section:
JavaScript, JScript
function Test()
{
// Select the mobile device
Mobile.SetCurrent("iPhone");
// Obtain the TableView object
var p = Mobile.Device().Process("SampleApp");
var tableview = p.Window().TableView();
// Touch the item
tableview.TouchItem("Section-1", 0);
tableview.LongTouchItem("Section-1", 2);
}
Python
def Test():
# Select the mobile device
Mobile.SetCurrent("iPhone")
# Obtain the TableView object
p = Mobile.Device().Process("SampleApp")
tableview = p.Window().TableView()
# Touch the item
tableview.TouchItem("Section-1", 0)
tableview.LongTouchItem("Section-1", 2)
VBScript
Sub Test()
Dim p, tableview
' Select the mobile device
Mobile.SetCurrent("iPhone")
' Obtain the TableView object
Set p = Mobile.Device.Process("SampleApp")
Set tableview = p.Window().TableView()
' Touch the item
Call tableview.TouchItem("Section-1", 0)
Call tableview.LongTouchItem("Section-1", 2)
End Sub
DelphiScript
procedure Test();
var
p, tableview;
begin
// Select the mobile device
Mobile.SetCurrent('iPhone');
// Obtain the TableView object
p := Mobile.Device.Process('SampleApp');
tableview := p.Window(0).TableView(0);
// Touch the item
tableview.TouchItem('Section-1', 0);
tableview.LongTouchItem('Section-1', 2);
end;
C++Script, C#Script
function Test()
{
// Select the mobile device
Mobile["SetCurrent"]("iPhone");
// Obtain the TableView object
var p = Mobile["Device"]["Process"]("SampleApp");
var tableview = p["Window"]()["TableView"]();
// Touch the item
tableview["TouchItem"]("Section-1", 0);
tableview["LongTouchItem"]("Section-1", 2);
}
Checking if Item Is Selected
You can check if an item is selected by using the wItemSelected
property. If the item is selected, this property returns true; otherwise - false. The following example checks whether the item is selected and posts the appropriate message to the test log:
JavaScript, JScript
function Test()
{
// Select the mobile device
Mobile.SetCurrent("iPhone");
// Obtain the TableView object
var p = Mobile.Device().Process("SampleApp");
var tableview = p.Window().TableView();
// Check if the item is selected
if (tableview.wItemSelected(0, 1))
Log.Message("The item is selected.")
else
Log.Message("The item is not selected.")
}
Python
def Test():
# Select the mobile device
Mobile.SetCurrent("iPhone")
# Obtain the TableView object
p = Mobile.Device().Process("SampleApp")
tableview = p.Window().TableView()
# Check if the item is selected
if tableview.wItemSelected[0, 1]:
Log.Message("The item is selected.")
else:
Log.Message("The item is not selected.")
VBScript
Sub Test()
Dim p, tableview
' Select the mobile device
Mobile.SetCurrent("iPhone")
' Obtain the TableView object
Set p = Mobile.Device.Process("SampleApp")
Set tableview = p.Window.TableView
' Check if the item is selected
If tableview.wItemSelected(0, 1) Then
Log.Message("The item is selected.")
Else
Log.Message("The item is not selected.")
End If
End Sub
DelphiScript
procedure Test();
var
p, tableview;
begin
// Select the mobile device
Mobile.SetCurrent('iPhone');
// Obtain the TableView object
p := Mobile.Device.Process('SampleApp');
tableview := p.Window(0).TableView(0);
// Check if the item is selected
if tableview.wItemSelected(0, 1) then
Log.Message('The item is selected.')
else
Log.Message('The item is not selected.')
end;
C++Script, C#Script
function Test()
{
// Select the mobile device
Mobile["SetCurrent"]("iPhone");
// Obtain the TableView object
var p = Mobile["Device"].Process("SampleApp");
var tableview = p["Window"]()["TableView"]();
tableview.TouchItem(0,1)
// Check if the item is selected
if (tableview["wItemSelected"](0, 1))
Log["Message"]("The item is selected.")
else
Log["Message"]("The item is not selected.")
}
Using Case-Sensitive and Case-Insensitive Item Captions
Depending on the Use case-sensitive parameters project option, TestComplete treats titles as case-sensitive or case-insensitive. By default, this option is turned off so that you can specify the title in any case (all upper cases, all lower cases, mixed cases, and so on):
tableview.TouchItem("Item1", "SEction1")
tableview.TouchItem("ITEM1", "SECTION1")
tableview.TouchItem("item1", "section1")
tableview.TouchItem("ItEm1", "SeCtion1")
If Use case-sensitive parameters is checked, you can only specify titles using the correct case:
tableview.TouchItem("Item1", "Section1") // Correct
tableview.TouchItem("item1", "section1") // Incorrect!!!
Using Wildcards and Regular Expressions in Items Captions
If item titles change according to the current context, it may be useful to specify items only by constant parts of their names. To specify arbitrary parts in item names, TestComplete lets you use wildcard characters (* and ?) or regular expressions. The asterisk (*) corresponds to a string of any length (including an empty string), the question mark corresponds to any single character (including none). To specify more complicated parts of a caption, use regular expressions.
For example, the tested application’s table view contains the Undo item whose title depends on the undo buffer: if there is an action to undo, the item's title contains the Undo word and the action name (for example, Undo Typing or Undo Paste); if there are no actions to undo, the item's title is Can't Undo. In order to ensure stable recognition of the Undo menu item whatever name it has, you can replace the variable part of the name with the * wildcard:
tableview.TouchItem("*Undo*", 0)
In this example, we place the wildcard on both sides of the Undo word since it can either precede or follow other text.