Description
The ImageRepository
object lets you work with image sets and image set items stored in the Image Repository. You can use these images to test black-box applications you cannot make open.
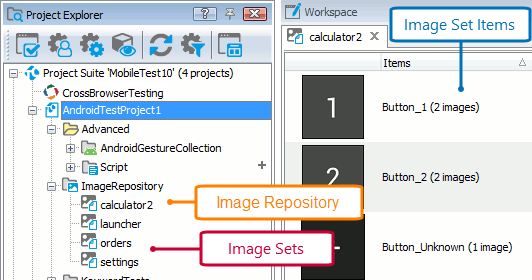
The ImageRepository
object provides access to the repository’s image sets by their names:
ImageRepository.ImageSet_Name
After you obtain an image set, you can work with its items. For more information on working with image set items, see the topic describing the ImageSetItem
object.
Example
The following code gets access to the specified image set (calculator) and simulates user actions over the specified items of this image set:
JavaScript, JScript
function TestCalc()
{
// Specify the current device
Mobile.SetCurrent("MyAndroidDev", 0);
// Run Calculator and simulate touches on its buttons
ImageRepository.launcher.TextView_Calculator.Touch();
var MySet = ImageRepository.calculator;
MySet.Button_5.Touch();
MySet.Button_Question1.Touch();
MySet.Button_2.Touch();
MySet.Button_Equal.Touch();
}
Python
def TestCalc():
# Specify the current device
Mobile.SetCurrent("VirtualBox")
# Run Calculator and simulate touches on its buttons
ImageRepository.Launcher.TextView_Calculator.Touch()
MySet = ImageRepository.Calculator
MySet.Button_5.Touch()
MySet.Button_Question1.Touch()
MySet.Button_2.Touch()
MySet.Button_Equal.Touch()
VBScript
Sub TestCalc
' Specify the current device
Call Mobile.SetCurrent("MyAndroidDev", 0)
' Run Calculator and simulate touches on its buttons
Call ImageRepository.launcher.TextView_Calculator.Touch
Set MySet = ImageRepository.calculator
Call MySet.Button_5.Touch
Call MySet.Button_Question1.Touch
Call MySet.Button_2.Touch
Call MySet.Button_Equal.Touch
End Sub
DelphiScript
procedure TestCalc();
var MySet;
begin
// Specify the current device
Mobile.SetCurrent('MyAndroidDev', 0);
// Run Calculator and simulate touches on its buttons
ImageRepository.launcher.TextView_Calculator.Touch();
MySet := ImageRepository.calculator;
MySet.Button_5.Touch();
MySet.Button_Question1.Touch();
MySet.Button_2.Touch();
MySet.Button_Equal.Touch();
end;
C++Script, C#Script
function TestCalc()
{
// Specify the current device
Mobile["SetCurrent"]("MyAndroidDev", 0);
// Run Calculator and simulate touches on its buttons
ImageRepository["launcher"]["TextView_Calculator"]["Touch"]();
var MySet = ImageRepository["calculator"];
MySet.Button_5["Touch"]();
MySet.Button_Question1["Touch"]();
MySet.Button_2["Touch"]();
MySet.Button_Equal["Touch"]();
}
See Also
Testing Android Applications (Legacy)
Image-Based Testing
ImageSetItem Object