In your tests, you may need to check whether the expected text is rendered on screen, and, depending on the check result, to perform various actions (for example, to simulate user actions or to post a custom message to the test log). You can do this in the following way:
-
Recognize the rendered text in the needed screen area by using the
OCR.Recognize
method. -
Get the recognized text by using the
OCR.Recognize.FullText
property. -
Check whether the obtained text contains the expected substring. To do this, you can use various operations that compare string values. For example, the
aqString.Find
method. See Working With Strings. -
Depending on the check results, perform the needed actions.
Note: To verify the text contents against a pattern and to post a success/fail message to the test log, use OCR checkpoints. See Verify Text Contents.
In scripts
The code below contains the CheckTextContents
routine that gets an onscreen object and a string and verifies that the object’s text includes the string. As the third parameter, the routine takes a Boolean value that specifies whether the check is case-sensitive or case-insensitive. To get the onscreen object’s text, the routine uses the OCR.Recognize.FullText
property. To verify that the rendered text contains the string, the routine uses the aqString.Find
method. If the expected substring is present, the routine returns True; otherwise, it returns False.
JavaScript, JScript
{
// Recognize the text contents of the specified onscreen object
var text = OCR.Recognize(anObject).FullText;
// Search for the occurrence of the specified substring in the recognized text
return (aqString.Find(text, aSubstring, 0, caseSensitive) > -1)
}
function Main()
{
var textToCheck = "substring";
// Get the onscreen object whose text will be checked
var form = Sys.WaitProcess("MyApp").WaitWindow("Window", "*", -1, 3000);
…
// Check the text
if (CheckTextContents(form, textToCheck, false))
Log.Message("The substring '" + textToCheck + "' has been found.");
else
Log.Warning("The substring " + textToCheck + " has not been found.");
…
}
Python
def CheckTextContents(anObject, aSubstring, caseSensitive=False):
# Recognize the text contents of the specified onscreen object
text = OCR.Recognize(anObject).FullText
# Searches for the occurrence of the specified substring in the recognized text
return (aqString.Find(text, aSubstring, 0 , caseSensitive) > -1)
def Main():
textToCheck = "substring"
# Get the onscreen object whose text will be checked
form = Sys.WaitProcess("MyApp").WaitWindow("Window", "*", -1, 3000)
if (form.Exists):
# Check the text
if (CheckTextContents(form, textToCheck, False)):
Log.Message("The substring '" + aqConvert.VarToStr(textToCheck) + "' has been found.")
else:
Log.Warning("The " + aqConvert.VarToStr(textToCheck) + " substring was not found.")
VBScript
' Recognize the text contents of the specified onscreen object
text = OCR.Recognize(anObject).FullText
' Search for the occurrence of the specified substring in the recognized text
CheckTextContents = (aqString.Find(text, aSubstring, 0 , caseSensitive) > -1)
End Function
Sub Main
textToCheck = "substring"
' Get the onscreen object whose text will be checked
Set form = Sys.WaitProcess("MyApp").WaitWindow("Window", "*", -1, 3000)
…
' Check the text
If CheckTextContents(form, textToCheck, false) Then
Log.Message("The substring '" & textToCheck & "' has been found.")
Else
Log.Warning("The substring '" & textToCheck & "' has not been found.")
End If
…
End Sub
DelphiScript
var text;
begin
// Recognize the text contents of the specified onscreen object
text := OCR.Recognize(anObject).FullText;
// Search for the occurrence of the specified substring in the recognized text
result : = (aqString.Find(text, aSubstring, 0, caseSensitive) > -1);
end;
function Main();
var textToCheck, form;
begin
textToCheck := 'substring';
// Get the onscreen object whose text will be checked
form := Sys.WaitProcess('MyApp').WaitWindow('Window', '*', -1, 3000);
…
// Check the text
if CheckTextContents(form, textToCheck, false) then
Log.Message('The substring ''' + aqConvert.VarToStr(textToCheck) + ''' has been found.')
else
Log.Warning('The substring ''' + aqConvert.VarToStr(textToCheck) + ''' has not been found.');
…
end;
C++Script, C#Script
{
// Recognize the text contents of the specified onscreen object
var text = OCR["Recognize"](anObject)["FullText"];
// Search for the occurrence of the specified substring in the recognized text
return (aqString["Find"](text, aSubstring, 0, caseSensitive) > -1);
}
function Main()
{
var textToCheck = "substring";
// Get the onscreen object whose text will be checked
var form = Sys["WaitProcess"]("MyApp")["WaitWindow"]("Window", "*", -1, 3000);
…
// Check the text
if (CheckTextContents(form, textToCheck, false))
Log["Message"]("The substring '" + textToCheck + "' has been found.");
else
Log["Warning"]("The substring '" + textToCheck + "' has not been found.");
…
}
The Main
routine in the example above shows how to call the CheckTextContents
routine to verify that the main form of the tested application renders the expected substring. Depending on the result, it posts the appropriate message to the test log.
In keyword tests
-
Copy the
CheckTextContents
function code from the example above to a script unit in your test project in TestComplete. -
Call this function from your keyword test. To do this, you can use the Run Code Snippet or Run Script Routine operation.
-
Get the function results. Depending on them perform needed actions. To learn how to get the routine results, see Checking Operation Result.
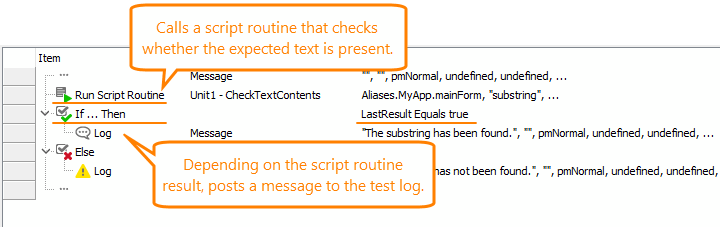
See Also
Verify Text Contents
Optical Character Recognition
Working With Strings