In TestComplete, you can measure the execution time of entire keyword tests or script routines, or individual test commands or groups of commands. This is helpful, for example, when you need to check if the execution time of your tests exceeds the specified values.
To time your test execution, you use time counters. A time counter is a timer that counts the number of milliseconds elapsed since it started. This topic explains how you can use counters in your tests.
Time Counter Scripting Methods and Keyword-Test Operations
To measure the execution time of your tests, you use operations of the Performance category or methods and properties of the aqPerformance
object:
Operation | Method (Property) | Description |
---|---|---|
Start | aqPerformance.Start |
Starts a counter. |
Get Value | aqPerformance.Value |
Returns the counter’s value (the number of milliseconds passed after the counter started). |
Check | aqPerformance.Check |
Gets the counter’s value, compares it with the specified limit and posts a message with comparison results to the test log. |
Group Check | – | Measures the execution time of child operations, compares it with the specified limit and posts a message with comparison results to the test log. |
About Counter Names
Both keyword-test operations and scripting methods have a parameter that specifies the counter name. This parameter is optional. If you omit it, the method or operation will work with the default counter. If you specify a name, the method (or operation) will work with the specified counter. The latter approach is useful if you need to use several counters. In many cases, however, using just one counter (the default one) is enough. See below for information on using counters in your tests.
Using Counters in Keyword Tests
To measure the execution time of your keyword test or operations:
-
Add the Start and Get Value operations to our test.
If you want to measure the execution time of the entire keyword test, make these operations the first and the last operations in the test.
If you are going to time several operations, place these operation before the first and the last operation to be measured: -
To output the measured time, add the Log Message operation right after the Get Value operation (as on the image above), and modify the operation properties so that it posts the [Last Operation Result] value to the test log.
Alternatively, you can save the measured time to a variable. To do this, add the Log Message Operation operation right after Get Value and configure its properties to save the [Last Operation Result] value to the desired variable. To learn more, see the Checking Operation Result topic.
To check the execution time of several operations, you can wrap these operations into the Group Check operation:
-
Select the desired operations in the keyword test editor.
-
Right-click the selected operations and choose Make Performance Check Group from the context menu:
-
Specify the desired maximum time and the name of the group (this name will help you identify the check results in the test log). Click OK to confirm the settings.
When you run the test, the Group Check operation will measure the execution time of its child operations, compare it with the expected maximum time and log success or failure to the test log:
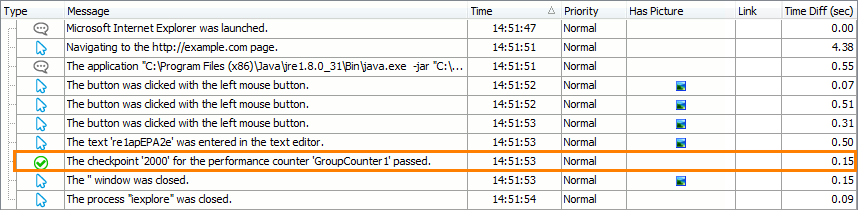
An alternative way to verify the execution time of operations is to use the Start and Check operations. In this case, you can place timing boundaries more freely. Also, you can use several Check operations to verify cumulative execution time:
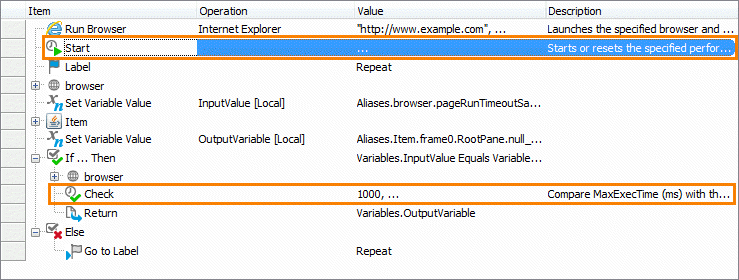
Using Counters in Scripts
The two following code snippets demonstrate how you can measure the execution time of your script code:
Example 1
The code below demonstrates how to get the time passed since a time counter started:
JavaScript
function getTime()
{
// Start a time counter
aqPerformance.Start();
// Do some actions
// Log the elapsed time, in ms
Log.Message(aqPerformance.Value());
}
JScript
function getTime()
{
// Start a time counter
aqPerformance.Start();
// Do some actions
// Log the elapsed time, in ms
Log.Message(aqPerformance.Value);
}
Python
def getTime():
# Start a time counter
aqPerformance.Start()
# Do some actions
# Log the elapsed time, in ms
Log.Message(aqPerformance.__getprop__("Value"))
VBScript
Sub getTime()
' Start a time counter
aqPerformance.Start()
' Do some actions
' Log the elapsed time, in ms
Log.Message(aqPerformance.Value)
End Sub
DelphiScript
function getTime();
begin
// Start a time counter
aqPerformance.Start();
// Do some actions
// Log the elapsed time, in ms
Log.Message(aqPerformance.Value);
end;
C++Script, C#Script
function getTime()
{
// Start a time counter
aqPerformance["Start"]();
// Do some actions
// Log the elapsed time, in ms
Log["Message"](aqPerformance["Value"]());
}
Example 2
The code below demonstrates how to check the execution time by using a time counter:
JavaScript, JScript
function checkTime()
{
// Start a time counter
aqPerformance.Start();
// Do some actions
// Check if the action takes less than 5 sec
aqPerformance.Check(5000);
}
Python
def checkTime():
# Start a time counter
aqPerformance.Start()
# Do some actions
# Check if the action takes less than 5 sec
aqPerformance.Check(5000)
VBScript
Sub checkTime()
' Start a time counter
aqPerformance.Start()
' Do some actions
' Check if the action takes less than 5 sec
aqPerformance.Check(5000)
End Sub
DelphiScript
function checkTime();
begin
// Start a time counter
aqPerformance.Start();
// Do some actions
// Check if the action takes less than 5 sec
aqPerformance.Check(5000);
end;
C++Script, C#Script
function checkTime()
{
// Start a time counter
aqPerformance["Start"]();
// Do some actions
// Check if the action takes less than 5 sec
aqPerformance["Check"](5000);
}
Using Multiple Time Counters
It is possible to use several time counters simultaneously. To do this, simply specify their names in the test commands that start and check counter values. The named counters are independent of each other.
You specify the name of a time counter as a parameter for all counter-related operations:
-
In keyword tests:
-
In scripts:
JavaScript, JScript
function Test()
{
// Start a time counter
aqPerformance.Start("RunApps");
// Run the tested application
TestedApps.Notepad.Run();
// Log the time of the application startup, in ms.
Log.Message(aqPerformance.Value("RunApps"));
// Start the second time counter
aqPerformance.Start("EnteringTextCounter");
// Enter text in the application
Sys.Process("notepad").Window("Notepad", "Untitled - Notepad", 1).Keys("Sample Text");
// Check if text entering takes less than 1 sec. by using the second time counter
aqPerformance.Check(1000, "Entering Text", "EnteringTextCounter");
}Python
def Test():
# Start a time counter
aqPerformance.Start("RunApps")
# Run the tested application
TestedApps.Notepad.Run()
# Log the time of the application startup, in ms.
Log.Message(aqConvert.IntToStr(aqPerformance.Value["RunApps"]))
# Start the second time counter
aqPerformance.Start("EnteringTextCounter")
# Enter text in the application
Sys.Process("notepad").Window("Notepad", "Untitled - Notepad", 1).Keys("Sample Text")
# Check if text entering takes less than 1 sec. by using the second time counter
aqPerformance.Check(1000, "Entering Text", "EnteringTextCounter")VBScript
Sub Test()
' Start a time counter
aqPerformance.Start("RunApps")
' Run the tested application
TestedApps.Notepad.Run()
' Log the time of the application startup, in ms.
Log.Message(aqPerformance.Value("RunApps"))
' Start the second time counter
aqPerformance.Start("EnteringTextCounter")
' Enter text in the application
Sys.Process("notepad").Window("Notepad", "Untitled - Notepad", 1).Keys("Sample Text")
' Check if text entering takes less than 1 sec. by using the second time counter
Call aqPerformance.Check(1000, "Entering Text", "EnteringTextCounter")
End SubDelphiScript
function Test();
begin
// Start a time counter
aqPerformance.Start('RunApps');
// Run the tested application
TestedApps.Notepad.Run();
// Log the time of the application startup, in ms.
Log.Message(aqPerformance.Value('RunApps'));
// Start the second time counter
aqPerformance.Start('EnteringTextCounter');
// Enter text in the application
Sys.Process('notepad').Window('Notepad', 'Untitled - Notepad', 1).Keys('Sample Text');
// Check if text entering takes less than 1 sec. by using the second time counter
aqPerformance.Check(1000, 'Entering Text', 'EnteringTextCounter');
end;C++Script, C#Script
function Test()
{
// Start a time counter
aqPerformance["Start"]("RunApps");
// Run the tested application
TestedApps["Notepad"]["Run"]();
// Log the time of the application startup, in ms.
Log["Message"](aqPerformance["Value"]("RunApps"));
// Start the second time counter
aqPerformance["Start"]("EnteringTextCounter");
// Enter text in the application
Sys["Process"]("notepad")["Window"]("Notepad", "Untitled - Notepad", 1)["Keys"]("Sample Text");
// Check if text entering takes less than 1 sec. by using the second time counter
aqPerformance["Check"](1000, "Entering Text", "EnteringTextCounter");
}