![]() |
Information in this topic applies to desktop applications only. |
![]() |
Connected and Self-Testing applications are deprecated. These technologies will be removed in one of the future releases of TestComplete. To create test code that runs from within your tested apps, use TestLeft, a SmartBear functional testing tool for developers. |
This topics explains how you can expose objects of Visual Basic 6.0 applications as scripting objects in TestComplete. To learn how you can create scripting objects from objects of Visual Basic .NET applications, see Creating Scripting Objects From Visual Basic .NET Application Objects.
Creating Custom Scripting Objects
Preparing Your Visual Basic 6.0 Project
Creating Custom Scripting Objects
TestComplete allows you to create top-level scripting objects from ActiveX objects that reside in Visual Basic 6.0 applications. If you need to expose an existing object of an application (for instance, a specific global or non-visual object), you need to create an ActiveX wrapper that provides access to this object. To learn how you can do this, please refer to the Visual Basic documentation.
To illustrate how ActiveX objects can be added to the TestComplete object model, let’s first create a new ActiveX library containing a sample scripting object. To create a new ActiveX library in Visual Basic:
-
Launch Microsoft Visual Basic 6.0.
-
Select File | New Project from the main menu.
-
In the resulting New Project dialog, select ActiveX DLL and press OK.
In our example, the object will contain one property, Text
, and one method, ShowText
. The property will store a text string, and the method will display this string in a message box:
Visual Basic
' SampleRuntimeObject.cls
Private FText As String
Public Property Get Text() As String
Text = FText
End Property
Public Property Let Text(Value As String)
FText = Value
End Property
Public Sub ShowText()
MsgBox (FText)
End Sub
Tip: | Help strings that you specify for methods and properties will be shown as a method (or a property) description in the Code Completion window. |
Now you can compile the library and register it in the system.
Preparing Your Visual Basic 6.0 Project
In order to be able to expose objects of Visual Basic applications as TestComplete scripting objects, you need to get access to the TestComplete COM server. The easiest way to manage TestComplete via COM is to transform your application into a Connected Application. Visual Basic Connected Applications are created by linking the <TestComplete>\Connected Apps\VB\TCConnect.bas module to the project. This module provides routines that free you from having to manually write the code needed to connect to TestComplete via COM.
For detailed instructions on how to create Visual Basic Connected Applications, see Creating Connected Applications in Visual Basic 6.0.
Registering and Unregistering Custom Scripting Objects
In order to add our custom object to the TestComplete object model, you need to register it in the TestComplete extensibility manager (see Creating Scripting Objects From Application Objects). In Visual Basic Connected Applications, you can address this manager using the TC.Manager
notation.
To register a custom scripting object, call the manager’s AddName
method. The method call can be inserted in the application’s initialization routine, or in a routine that is called upon a specific event. In our example, we will add a button control to the application form and place the registration code in the button’s OnClick
event handler. This way, we will be able to easily re-register our object in subsequent TestComplete sessions while the application is running.
The sample code is provided below. It requires a form that has the class name TForm1 and contains a button named Button1.
Visual Basic
Private RuntimeObj As Object
Private FRegistered As Boolean
Private Const RuntimeObjName = "SampleRuntimeObject"
Private Sub Form_Load()
Set RuntimeObj = CreateObject("SampleLib.SampleRuntimeObject")
FRegistered = False
End Sub
Private Sub Command1_Click()
Dim FSO As Object
Dim ProjectPath As String
ProjectPath = "TCProject\SampleRuntimeObject.pjs"
' Open a project suite in TestComplete
TC.Visible = True
Set FSO = CreateObject("Scripting.FileSystemObject")
TC.Integration.OpenProjectSuite (FSO.GetAbsolutePathName(ProjectPath))
' Register a new runtime object
TC.Manager.AddName RuntimeObjName, False, RuntimeObj
FRegistered = True
End Sub
To unregister an object, use the RemoveName
method of the TestComplete extensibility manager. In our example, we will place the unregistration code in the form’s Unload
event handler, so that the object is unregistered when the user exits the application by closing its main form. Since the unregistration makes sense only if the object has been previously registered and if TestComplete is running, we check both these conditions before calling the RemoveName
method:
Visual Basic
Private Sub Form_Unload(Cancel As Integer)
Dim TCExists As Object
On Error Resume Next
Set TCExists = GetObject(, "TestComplete.TestCompleteApplication.15")
If FRegistered And Not (TCExists Is Nothing) Then
TC.Manager.RemoveName RuntimeObjName
End If
End Sub
Working With Custom Scripting Objects
After a custom scripting object is registered in TestComplete, it is displayed in the Code Completion window and can be used in scripts:
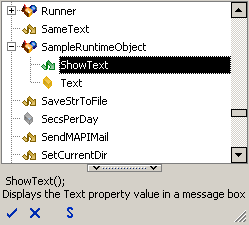
You can get and set the object’s property values, call its methods and check their return values the same way you would with standard scripting objects such as TestedApps
, Log
and others. The only difference is that the processing is performed by your application rather than by TestComplete.
The code snippet below demonstrates how you can work with the sample scripting object we have created earlier in this topic. This object is available in TestComplete from the time you launch the application and click the button on its main form and until the application is closed. Note that, despite that the object is a part of a Visual Basic application, it can be used in scripts written in any scripting language, not only VBScript:
JavaScript, JScript
function Main()
{
SampleRuntimeObject.Text = "Hello, world!";
SampleRuntimeObject.ShowText();
}
Python
def Main():
SampleRuntimeObject.Text = "Hello, world!"
SampleRuntimeObject.ShowText()
VBScript
Sub Main
SampleRuntimeObject.Text = "Hello, world!"
SampleRuntimeObject.ShowText
End Sub
DelphiScript
procedure Main;
begin
SampleRuntimeObject.Text := 'Hello, world!';
SampleRuntimeObject.ShowText;
end;
C++Script, C#Script
function Main()
{
SampleRuntimeObject["Text"] = "Hello, world!";
SampleRuntimeObject["ShowText"]();
}
See Also
Creating Scripting Objects From Application Objects
Connected Applications - Overview
Creating Connected Applications in Visual Basic 6.0
Creating Scripting Objects From Visual Basic .NET Application Objects