About
TestComplete provides scripting access to BDD features and scenarios in your project.
The top-level object from which you start is Features
. It provides access to the collection of Feature
elements defined in your project, and contains two methods – Count
and Item
– for iterating through this collection.
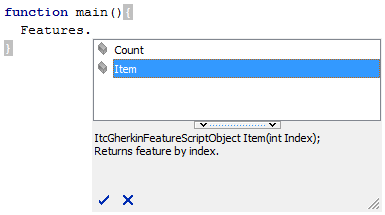
The Features.Item(...)
property provides a scripting interface to individual features defined in your project. This Feature
object has the following members:
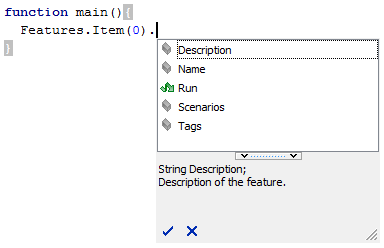
-
The
Name
andDescription
properties return the name and description of the feature (Name
is the text of the first string after theFeature:
element,Description
is text of other strings). -
The
Run
method runs the feature from script code. -
The
Tags
property provides access to the tags assigned to the feature (you can use tags to organize and run features and scenarios). This property is a collection object with the following properties and methods: -
The
Scenarios
property is a collection of scripting objects that correspond to scenarios defined in the feature.Each item of this collection has the following methods and properties:
-
The
Name
andDescription
properties return the name and description of the scenario. -
The
Run
method runs the scenario from script code. -
The
Tags
property provides access to the tags assigned to the scenario.
-
Let’s see how you can use these objects, methods and properties in your script tests.
Example 1 - Run a feature by name
The following code finds a feature by a substring in its name and runs this feature. Similarly, you can find a feature by a substring in its description:
JavaScript, JScript
function runFeatureByName(phrase) {
// Iterate through features
for (i = 0; i < Features.Count; i++) {
var name = Features.Item(i).Name;
// Check the feature name
if (name.indexOf(phrase) >= 0)
Features.Item(i).Run();
// You can also pass the entire feature name and
// compare it using code like
// if (name == phrase) ...
}
}
Python
def runFeatureByName(phrase):
# Iterate through features
for i in range(0, Features.Count):
name = Features.Item[i].Name
# Check the feature name
if aqString.Find(name, phrase) >= 0 :
Features.Item[i].Run()
# You can also pass the entire feature name and
# compare it using code like
# if (name == phrase) ...
VBScript
Sub runFeatureByName(phrase)
' Iterate through features
For i = 0 To Features.Count - 1
name = Features.Item(i).Name
' Check the feature name
If aqString.Find(name, phrase) >= 0 Then
Features.Item(i).Run
' You can also pass the entire feature name and
' compare it using code like
' If (name = phrase) Then ...
End If
Next
End Sub
DelphiScript
// DelphiScript does not support BDD tests.
C++Script, C#Script
function runFeatureByName(phrase) {
// Iterate through features
for (i = 0; i < Features["Count"]; i++) {
var name = Features["Item"](i)["Name"];
// Check the feature name
if (name.indexOf(phrase) >= 0)
Features["Item"](i)["Run"]();
// You can also pass the entire feature name and
// compare it using code like
// if (name == phrase) ...
}
}
Example 2 - Run a scenario by name
The following code finds a feature and a scenario by substrings in their names (phrase1 is a substring in the feature name, phrase2 is a substring in the scenario name), and then runs the found scenario:
JavaScript, JScript
function runScenarioByName(phrase1, phrase2) {
// Iterate through features
for (i = 0; i < Features.Count; i++) {
var name = Features.Item(i).Name;
// Check the feature name
if (name.indexOf(phrase1) >= 0) {
// Iterate through scenarios
for (j = 0; j < Features.Item(i).Scenarios.Count - 1; j++) {
var scenarioName = Features.Item(i).Scenarios.Item(j).Name;
// Check the scenario name
if (scenarioName.indexOf(phrase2) >= 0)
Features.Item(i).Scenarios.Item(j).Run();
}
}
}
}
Python
def runScenarioByName(phrase1, phrase2):
# Iterate through features
for i in range(0, Features.Count):
name = Features.Item[i].Name
# Check the feature name
if aqString.Find(name, phrase1) >= 0 :
# Iterate through scenarios
for j in range(0, Features.Item[i].Scenarios.Count):
scenarioName = Features.Item[i].Scenarios.Item[j].Name
# Check the scenario name
if aqString.Find(scenarioName, phrase2) >= 0 :
Features.Item[i].Scenarios.Item[j].Run()
VBScript
Sub runScenarioByName(phrase1, phrase2)
' Iterate through features
For i = 0 To Features.Count - 1
name = Features.Item(i).Name
' Check the feature name
If aqString.Find(name, phrase1) >= 0 Then
' Iterate through scenarios
For j = 0 To Features.Item(i).Scenarios.Count - 1
scenarioName = Features.Item(i).Scenarios.Item(j).Name
' Check the scenario name
If aqString.Find(scenarioName, phrase2) >= 0 Then
Features.Item(i).Scenarios.Item(j).Run
End If
Next
End If
Next
End Sub
DelphiScript
// DelphiScript does not support BDD tests.
C++Script, C#Script
function runScenarioByName(phrase1, phrase2) {
// Iterate through features
for (i = 0; i < Features["Count"]; i++) {
var name = Features["Item"](i)["Name"];
// Check the feature name
if (name.indexOf(phrase1) >= 0) {
// Iterate through scenarios
for (j = 0; j < Features["Item"](i)["Scenarios"]["Count"] - 1; j++) {
var scenarioName = Features["Item"](i)["Scenarios"]["Item"](j)["Name"];
// Check the scenario name
if (scenarioName.indexOf(phrase2) >= 0)
Features["Item"](i)["Scenarios"]["Item"](j)["Run"]();
}
}
}
}
Example 3 - Run by tag
See Also
Behavior-Driven Development (BDD) With TestComplete
Run BDD Tests