When creating automated tests for a .NET application, you may need to create instances of .NET types (classes, structures and so on) used in this application. For example, you may need to pass such an object as a parameter to some native method of this application.
General Procedure
To create an instance of a .NET type used in an application (either defined in the application itself or in its referenced assemblies), you can use the following syntax:
JavaScript, JScript
// Use this if the application process exists in Name Mapping
var obj = Aliases.MyApp.AppDomain("MyApp.exe").dotNET.namespace_name.class_name.zctor(parameters);
// Use this if the application process is not in Name Mapping
var obj = Sys.Process("MyApp").AppDomain("MyApp.exe").dotNET.namespace_name.class_name.zctor(parameters);
Python
# Use this if the application process exists in Name Mapping
obj = Aliases.MyApp.AppDomain("MyApp.exe").dotNET.namespace_name.class_name.zctor(parameters);
# Use this if the application process is not in Name Mapping
obj = Sys.Process("MyApp").AppDomain("MyApp.exe").dotNET.namespace_name.class_name.zctor(parameters);
VBScript
' Use this if the application process exists in Name Mapping
Set obj = Aliases.MyApp.AppDomain("MyApp.exe").dotNET.namespace_name.class_name.zctor(parameters)
' Use this if the application process is not in Name Mapping
Set obj = Sys.Process("MyApp").AppDomain("MyApp.exe").dotNET.namespace_name.class_name.zctor(parameters)
DelphiScript
// Use this if the application process exists in Name Mapping
obj := Aliases.MyApp.AppDomain('MyApp.exe').dotNET.namespace_name.class_name.zctor(parameters);
// Use this if the application process is not in Name Mapping
obj := Sys.Process('MyApp').AppDomain('MyApp.exe').dotNET.namespace_name.class_name.zctor(parameters);
C++Script, C#Script
// Use this if the application process exists in Name Mapping
var obj = Aliases["MyApp"]["AppDomain"]("MyApp.exe")["dotNET"]["namespace_name"]["class_name"]["zctor"](parameters);
// Use this if the application process is not in Name Mapping
var obj = Sys["Process"]("MyApp")["AppDomain"]("MyApp.exe")["dotNET"]["namespace_name"]["class_name"]["zctor"](parameters);
In this syntax:
-
The
AppDomain("MyApp.exe")
object corresponds to the application domain into which the assembly containing the target .NET type is loaded. Typically, the domain name is the same as the application’s file name. However, an application can have multiple domains with different names as well as domains corresponding to different versions of .NET runtime (see below). -
dotNET
is anAppDomain
property that provides access to all namespaces and types in assemblies loaded into the application domain. Its functionality is similar to that of the globaldotNET
object that lets you access classes in arbitrary .NET assemblies. -
namespace_name
andclass_name
are the full namespace name and .NET class name with dots ( . ) replaced with underscores ( _ ). For example, to refer to theSystem.Collections
namespace, useSystem_Collections
. -
zctor
is the TestComplete name for the .NET class constructor method. If the class has several constructors with different parameters, TestComplete appends indexes to constructor names in order to distinguish among them. For example,zctor
,zctor_2
,zctor_3
and so on. To determine which index-suffixed constructor name corresponds to which constructor implementation, examine the constructor declarations in the Object Browser.
Once you have created a .NET class instance, you can get and set its property values, call its methods and pass it as a parameter to methods of other .NET objects.
![]() |
Note that the object you want to pass as a parameter must met the following requirements:
|
For example, the sample Orders application shipped with TestComplete defines a custom namespace Orders
and a structure type Product
with the following constructor:
In scripts, you can create an instance of this structure as follows:
JavaScript, JScript
function Test()
{
var product = Sys.Process("Orders").AppDomain("Orders.exe").dotNET.Orders.Product.zctor("MuchoMoney", 99, 5);
Log.Message("Product name: " + product.ProdName);
Log.Message("Product price: $" + product.Price);
}
Python
def Test():
product = Sys.Process("Orders").AppDomain("Orders.exe").dotNET.Orders.Product.zctor("MuchoMoney", 99, 5);
Log.Message("Product name: " + VarToStr(product.ProdName));
Log.Message("Product price: $" + VarToStr(product.Price));
VBScript
Sub Test
Dim product
Set product = Sys.Process("Orders").AppDomain("Orders.exe").dotNET.Orders.Product.zctor("MuchoMoney", 99, 5)
Log.Message "Product name: " + product.ProdName
Log.Message "Product price: $" + product.Price
End Sub
DelphiScript
procedure Test;
var product;
begin
product := Sys.Process('Orders').AppDomain('Orders.exe').dotNET.Orders.Product.zctor('MuchoMoney', 99, 5);
Log.Message('Product name: ' + product.ProdName);
Log.Message('Product price: $' + VarToStr(product.Price));
end;
C++Script, C#Script
function Test()
{
var product = Sys["Process"]("Orders")["AppDomain"]("Orders.exe")["dotNET"]["Orders"]["Product"]["zctor"]("MuchoMoney", 99, 5);
Log["Message"]("Product name: " + product["ProdName"]);
Log["Message"]("Product price: $" + product["Price"]);
}
Creating .NET Class Instances in Keyword Tests
In keyword tests, you can create .NET class instances using the On-Screen Action or Call Object Method operation, as shown in the following image. For this purpose, specify the .NET class name in the Aliases.MyApp.AppDomain("MyApp.exe").dotNET.namespace_name.class_name
syntax (see above) as the target object name. Then select the needed zctorNNN
constructor method and specify the constructor parameters if any.
You can assign the created .NET object to a test variable and then pass this variable as a parameter to other operations. You can also call access methods and properties of the object stored in a variable, for example, post them to the test log.
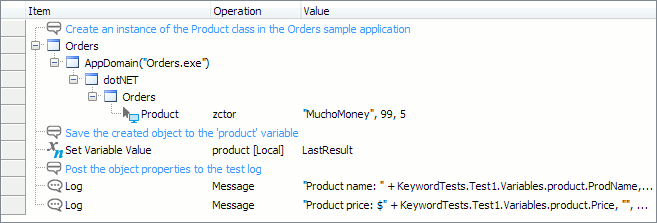
Alternatively, you can write a script routine that will create and use the needed .NET class instance and call this routine from your keyword test using the Run Script Routine operation.
Choosing Between Different Application Domains
.NET applications can host multiple .NET runtimes (CLRs) in the same process. For example, a .NET 4 application can host both .NET 4 and .NET 2 runtimes. In the Object Browser, such applications have several AppDomain
objects corresponding to different versions of .NET runtime:
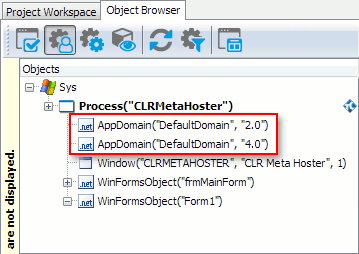
When dealing with such applications, keep in mind that the same .NET class can have different implementations in different versions of .NET runtime. For example, a newer implementation that targets .NET 4 may have additional methods and properties compared to the implementation for .NET 2.
In this case, you need to create the desired .NET class instance in the context of the appropriate application domain. For example, if you need to create a .NET 4-specific class instance, use the AppDomain(DomainName, "4.0")
object.
See Also
Testing .NET Applications
Accessing Native Properties and Methods of .NET Objects
Calling Functions From .NET Assemblies