You can extend the default reports by using report scripts that are available for test suite and test case reports. ReadyAPI runs report scripts each time it creates a report. To work with the script, use the script editor on the Report tab of the corresponding editor:
Report scripts can use the following objects:
-
log
– an object for interacting with the log. -
report
– an object that holds all data used to populate the report. Its members allows you to add or adjust data and subreport data the report engine uses to fill the report template. -
params
– an object that contains all defined report parameters and their values. -
Also, scripts can access an object that represents the current test item (
testSuite
ortestCase
).
Mostly, when working with your report data, you will use the report
and params
objects.
Below is information on how to perform some useful tasks by using the report script.
Changing Report Parameters
The params
object allows you to modify parameters declared in a report template or on the Report Parameter tab.
To access a parameter, specify its name after the period:
Groovy
params.<ParameterName> = "New Value";
For example, you define an integer parameter in a report template:
JRXML
<parameter name="MyParameter" class="java.lang.Integer">
<defaultValueExpression>1</defaultValueExpression>
</parameter>
Now, you can change it from script by using the following expression:
Groovy
params.MyParameter = 32;
Adding Metrics to a Report
Each default report includes some metrics produced by the MetricsReport
subreport. This subreport shows the execution-related metrics. You can include extra metrics in this report by using the report.addMetric()
method. For example, the following code snippet adds the version of the operating system:
Groovy
report.addMetric("OS Version", System.getProperty("os.version"));
By default, new metrics are added to the Base Metrics section. You can also add them to a custom section. The code snippet below shows how to list all the system properties under the System Properties category.
Groovy
for (name in System.getProperties().propertyNames())
{
report.addMetric("System Properties", name, System.getProperty(name), null);
}
Working With Subreports
You use subreports to create a report within the main report. Each subreport uses a template and provides a data source to include the needed data in the parent report.
Using the report
object you can access an existing subreport as well as create new ones. The example below shows how to create a subreport containing system properties.
Creating a Helper Class
A system property consists of two parts: the name and value. To pass it to a subreport, you can use a helper class that has two variables: name
and value
. ReadyAPI passes this class to the subreport template for each data record. To declare the class, open the Report script tab and enter the following code:
Groovy
class NVPair
{
String name; // This variable contains the name of a property
String value; // This variable contains the value of a property
public NVPair(String n, String v)
{
name = n;
value = v;
}
}
Creating a Subreport Template
-
From the main menu, select Project > Reporting.
-
In the Reporting dialog, switch to the Subreports tab.
-
Click
to create a new subreport template.
-
Specify the properties of the new template:
-
Enter the following text as the new template content:
JRXML
<jasperReport xsi:schemaLocation="http://jasperreports.sourceforge.net/jasperreports http://jasperreports.sourceforge.net/xsd/jasperreport.xsd" name="report name" language="groovy" pageWidth="535" pageHeight="842" columnWidth="535" leftMargin="0" rightMargin="0" topMargin="0" bottomMargin="0" xmlns="http://jasperreports.sourceforge.net/jasperreports" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<template>"../../styles.jrtx"</template>
<field name="name" class="java.lang.String"/>
<field name="value" class="java.lang.String"/>
<columnHeader>
<band height="59">
<staticText>
<reportElement style="ColumnHeader" x="0" y="35" width="122" height="20"/>
<textElement textAlignment="Left" verticalAlignment="Middle"/>
<text>Name</text>
</staticText>
<staticText>
<reportElement style="ColumnHeader" x="133" y="35" width="40" height="20"/>
<textElement textAlignment="Left" verticalAlignment="Middle"/>
<text>Value</text>
</staticText>
<staticText>
<reportElement style="SmallHeader" x="0" y="0" width="535" height="34"/>
<textElement textAlignment="Left" verticalAlignment="Middle"/>
<text>System Properties</text>
</staticText>
</band>
</columnHeader>
<detail>
<band height="21">
<textField>
<reportElement x="0" y="1" width="122" height="20"/>
<textElement textAlignment="Left" verticalAlignment="Top"/>
<textFieldExpression class="java.lang.String">$F{name}</textFieldExpression>
</textField>
<textField>
<reportElement x="133" y="1" width="350" height="20"/>
<textElement textAlignment="Left" verticalAlignment="Top"/>
<textFieldExpression class="java.lang.String">$F{value}</textFieldExpression>
</textField>
</band>
</detail>
</jasperReport>The
field
elements represent a piece of data obtained from a data source. Thename
attribute of these fields must coincide with variable names declared in a class representing the data record. In this example, two fields,name
andvalue
, match variables declared in theNVPair
class on the previous step.To insert field values, use the
$F{<field name>}
expression. In the example above, the$F{name}
expression inserts the value of thename
variable, and$F{value}
inserts the value of the variable.
Adding a Subreport to a Report Template
To add a subreport to a report, use the subreport
element of the report template. To do this, perform the following steps:
-
Switch to the Available Reports tab of the Reporting dialog.
-
Select the template to which you want to add a template. For example, the standard
Test Case Report
. -
Insert the following text to a
band
element, which is a child element of thedetail
element:JRXML
<subreportExpression class="java.lang.String">"subreport:TestStepParametersReport"</subreportExpression>
</subreport>
<subreport isUsingCache="true">
<reportElement positionType="Float" isPrintRepeatedValues="false" x="0" y="318" width="535" height="100"/>
<dataSourceExpression>$P{SystemProperties}</dataSourceExpression>
<subreportExpression>"subreport:SystemPropertiesReport"</subreportExpression>
</subreport>
</band>
</detail>Make sure the value of the height
attribute of theband
element is greater than the value of theheight
attribute of the subreport footer element. In this example, it must be greater than 418 (the sum of they
andheight
values).The following elements specify subreport details:
-
The
dataSourceExpression
element specifies the data source that will feed a subreport with data. -
The
subreportExpression
element specifies the used subreport template.
-
-
To pass a data source to the subreport, we use the
SystemProperties
parameter. To declare the parameter in the template, specify a newparameter
element at the beginning of the template:JRXML
</parameter>
<parameter name="SystemProperties" class="net.sf.jasperreports.engine.JRDataSource" isForPrompting="false">
<property name="interactive" value="false"/>
</parameter>
<pageHeader>
Generating Data
To grab data to show and produce the needed data source, create a custom SystemPropertiesSubReport
class.
-
Add the following code at the beginning of the Report script
Groovy
import com.eviware.soapui.reporting.reports.support.AbstractJasperSubReport;
import net.sf.jasperreports.engine.data.JRBeanCollectionDataSource;
public class SystemPropertiesSubReport extends AbstractJasperSubReport
{
public SystemPropertiesSubReport (def modelItem)
{
super(modelItem, "SystemProperties", false);
}
// Create an array of the NVpair elements that is used as a data source
public JRBeanCollectionDataSource buildDataSource(def modelItem)
{
List props = new ArrayList();
// Iterate through system properties
for(name in System.properties.propertyNames())
{
// Create an NVPair instance that contains the name and value of a system property
def prop = new NVPair(name, System.getProperty(name));
// Add the system property to the array
props.add(prop)
}
// Return the created array as a data source collection
return new JRBeanCollectionDataSource(props);
}
} -
To build a data source and pass it to the report template, use the following code:
Groovy
// Create a subreport instance
def subreport = new SystemPropertiesSubReport(testCase);
// Generate a data source and pass it to the SystemProperties parameter of the report template
params.SystemProperties = subreport.buildDataSource(report);
To test the created subreport, click and select the template to which you are adding the subreport:
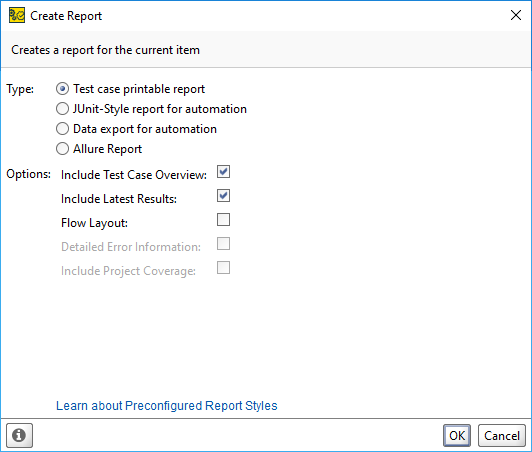
Making a Subreport Optional
You can make this subreport optional:
-
Include the following
parameter
element at the end of parameters element in the main template:XML
...
</parameter>
<parameter class="java.lang.Boolean" name="IncludeSystemProperties">
<property name="interactive" value="true"/>
<property name="label" value="Include System Properties"/>
<parameterDescription>Includes System Properties in Report</parameterDescription>
<defaultValueExpression>true</defaultValueExpression>
</parameter>
<detail>
... -
Add the following conditional to the
subreport
element:JRXML
...
<subreport isUsingCache="true">
<reportElement height="30" isPrintRepeatedValues="false" positionType="Float" width="500" x="0" y="50">
<printWhenExpression>$P{IncludeSystemProperties}</printWhenExpression>
</reportElement>
...
</subreport>
...
Now when you select the created template in the Create Report dialog, you can select whether to include this subreport in the report:
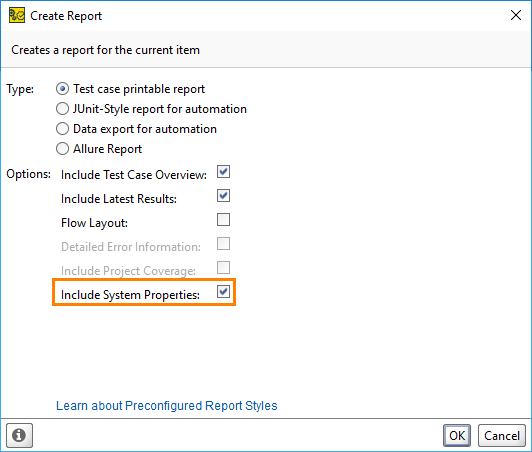
See Also
Managing Report Templates
Customizing Templates Tutorial
Configuring Report Templates